WordPress Hooks: How to use actions and filters
WordPress Hooks allow you to not only edit or expand the basic functionality of WordPress but also to modify plugins and themes and allow other developers to integrate their code within your plugins or themes.
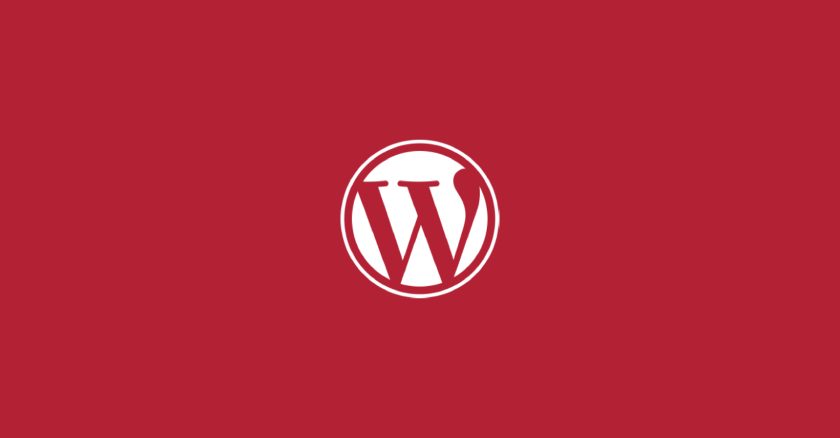
WordPress Hooks are one of the most crucial tools in the arsenal of every WordPress developer. They serve as the foundation for the creation of WordPress plugins and themes. You can utilize WordPress’ various built-in hooks to ‘hook into’ the WordPress Core and do or alter something using your custom code.
WordPress hooks are classified into two types: actions and filters. Hooks are so popular that WordPress Core makes significant use of them. WordPress also allows you to develop your own custom hooks, allowing other developers to hook into your code.
To master WordPress programming, you must first understand how actions, filters, and custom hooks operate.
What exactly are WordPress Hooks?
A WordPress page is put together by the many functions and database queries. The WordPress Core, plugins, and theme all collaborate to generate page components like text, graphics, scripts, and styles. When all of the components are complete, the browser assembles them together and displays the page.
WordPress hooks enable you to “hook into” the build process at certain times and execute custom code. Hooks’ primary goal is to allow you to alter or add functionality to WordPress without modifying the core files.
WordPress hooks are powered by the WordPress Plugin API. Hooks are activated by invoking particular WordPress functions known as Hook Functions at specific times throughout the WordPress runtime.
You may use hook functions to wrap your custom code into a callback function and register it with any hook. Once registered, this callback will be executed anywhere the hook is located, enabling you to supplement or replace the usual WordPress functionality.
Actions and Filters
WordPress has two sorts of “hooks”: Actions and Filters. Actions allow you to do anything at preset points throughout the WordPress runtime, whereas Filters allow you to alter and return any data processed by WordPress.
Let’s look at the distinctions between Actions and Hooks:
Actions | Filters |
WordPress actions are used to execute a specific code written by the developer at a specific point during the execution of WordPress. | The concept of WordPress filters is similar to that of actions, with the primary distinction being that filters are used to manipulate variables. |
add_action(), remove_action(), do_action(), has_action() | add_filter(), remove_filter() |
No need of passing arguments. | You need to pass at least one argument, |
An action stops the normal execution process of the code to perform something with the information it receives, returns nothing, and then exits. | A filter adjusts the information it receives before returning it to the calling hook function, and the result it provides can be used by other functions. |
WordPress Action Hooks
If you want WordPress to do something when the post is published, you simply need to add the custom code to the save_post action.
<?php
add_action("save_post", "do_sumething_on_publish");
function do_sumething_on_publish( $post_id ){
// Your code goes here and it will be executed everytime when the user saves a post.
}
This code may be placed in a plugin or the theme’s functions.php file.
if you want to use a custom hook simply use: do_action(‘custom_action’);
WordPress Filter Hooks
The concept of WordPress filters is similar to that of actions, with the primary distinction being that filters are used to manipulate variables. In contrast to actions, filters code must return a value that is a modified duplicate of the original value.
<?php
add_filter("the_title" , "modify_post_titles" );
function modify_post_titles( $post_title ){
$title = strtoupper( $post_title );
// return the title.
return $title;
}
How to use WordPress hooks?
A WordPress hook accomplishes nothing on its own. It just waits in the code, awaiting some hook function to trigger it. To implement a hook, you must call at least two additional functions.
To begin, you must register the hook using a hook function and provide a callback function within it. Then, in the hook function, you must define the callback function that you described previously. When the hook is triggered, WordPress will execute this callback function.
It doesn’t matter what order you declare these functions in, although it’s a good idea to put them near together.
Hooking an Action
You can implement a callback function hooked to action by doing the following steps:
- Implement the callback function.
- Hook the custom callback function to an action using add_action()
- add_action() accepts two more parametars: priority and number of argumnets.
<?php
// implement the callback function, arguments are optional
function callback_function( $arg1, $arg2 ) {
// your code goes here
}
// hook the callback_function to the 'example_action'
add_action( 'some_action', 'callback_function', $priority, $number_of_args] );
// 'priority' and 'number of arguments' are optional
Other Action Functions
- has_action() – check if an action exists
- do_action() – used for creating a custom actions
- do_action_ref_array() – calls the callback functions attached to an action hook, using parameters specified in an array.
- did_action() – the number of times an action was executed during the current request
- remove_action() – An action hook’s callback function is removed.
- remove_all_actions() – it will remove every function that is hooked to a curtain action
- doing_action() – it a curtain action is run
has_action( 'action_name', 'callback_function' );
This action function determines whether or not an action has been hooked. It only needs two parameters. The first one is the action’s name and the second parameter is optional (this is the name of the callback function).
<?php
remove_action( 'action_name', 'function_to_be_removed', $priority );
remove_all_actions( 'action_name', $priority );
The function to be removed and priority arguments must match those used in the add_action() function.
<?php
// check whether the 'action_name' action is being executed
if ( doing_action( 'action_name' ) ) {
// execute your code here
}
To see if any action is being executed, leave the action name option empty. Every time an action is fired, it will return true.
Hooking a Filter
Filters allow your custom code to manipulate data that is utilized by other WordPress functions. Functions connected to filters, unlike actions, must return a value.
- Implement the callback function.
- Hook the custom callback function to a filter using add_filter()
- add_filter() accepts two more parametars: priority and number of argumnets.
<?php
// define the filter callback function
function callback_function( $arg1, $arg2 ) {
// make your code do something with the arguments and return something
return $output;
}
// hook the callback function
add_filter( 'example_filter', 'callback_function', $priority, $args );
Filters Supported by WordPress
You can almost probably find a filter to hook into and alter anywhere WordPress processes or edits data. Consider filters to be a bridge between the database and the browser.
The Plugin API/Filter Reference page contains an extensive list of all the filters supported by WordPress.
How the filters are used in the WordPress Core
Many of WordPress Core’s built-in filters are used to change data utilized by its many functionalities.
Take, for example, the content filter. It filters post content after it has been fetched from the database but before it is shown in the browser.
add_filter( 'the_content', 'do_blocks', 9 );
add_filter( 'the_content', 'wptexturize' );
add_filter( 'the_content', 'convert_smilies', 20 );
add_filter( 'the_content', 'wpautop' );
add_filter( 'the_content', 'shortcode_unautop' );
add_filter( 'the_content', 'prepend_attachment' );
add_filter( 'the_content', 'wp_make_content_images_responsive' );
add_filter( 'the_content', 'do_shortcode', 11 ); // AFTER wpautop().
Other Filter Functions
add_filter() is the most commonly used filter function, but there are a plethora of other useful filter methods.
- has_filter()
- apply_filters()
- apply_filters_ref_array()
- current_filter()
- remove_filter()
- remove_all_filters()
- doing_filter()
Hooks List and Resources
It’s difficult to remember all of the many hooks available in WordPress. There are hundreds of built-in actions and filters to which you may connect. As a result, finding an acceptable hook might feel like a scavenger quest at times.
Fortunately, there are several tools available to help you find the best hook for your purposes.
- Plugin API – Hooks Function Reference
- Plugin API – Action Reference
- Plugin API – Filter Reference
- Code Reference
The WordPress Codex also contains a search tool for quickly locating all of its functions, hooks, methods, and classes. This page also lists new and updated WordPress components in the most recent version.
The special “all” hook
WordPress includes a specific hook called ‘all’ that you may use to execute a callback function for every single hook, regardless of whether it’s registered with them all or not. It’s important for debugging page failures or determining when a certain event occurs.
Summary
If you’re a WordPress developer, there are several benefits to employing WordPress hooks.
Hooks allow you to not only edit or expand the basic functionality of WordPress but also to modify plugins and themes and allow other developers to integrate their code within your plugins or themes.
Was this post helpful? ( Answers: 0 )
Leave a comment
If you enjoyed this post or have any questions, please leave a comment below. Your feedback is valuable!