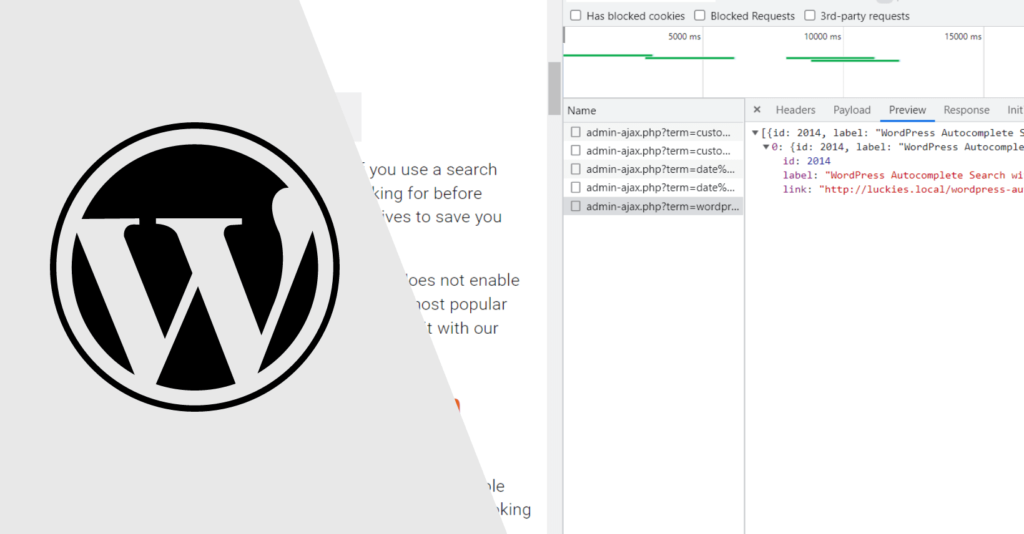
WordPress Autocomplete Search with AJAX
WordPress Autocomplete Search is everywhere. You increase the chances that people will spend more time on your site by implementing WordPress Autocomplete Search. You make it easy for them to find the information they need.
WordPress Autocomplete Search is everywhere. If you use a search tool, it’s likely that it will understand what you’re looking for before you’ve finished typing and will begin offering alternatives to save you time.
You can enhance the likelihood that people will spend more time on your site by implementing WordPress Autocomplete Search. You make it easy for them to find the information they need, which improves their entire experience.
Although WordPress comes with search capabilities, it does not enable ‘autocomplete search’ or ‘live search,’ which are the two most popular terms for this feature. However, you can easily implement it with our help. Let’s dive in.
WordPress Autocomplete Search without a Plugin
Users nowadays expect to get the information they want fast. People have grown accustomed to finding the pages and articles they’re looking for with minimum effort as search engines get more accurate.
As you may think, manually adding WordPress autocomplete search feature to your website requires some coding.
Setting up the code
You can use the functions.php file or an active plugin. It is up to you. The hooks that we are going to use are these:
- wp_enqueue_scripts
- wp_ajax_{action_name}
- wp_ajax_nopriv_{action_name}
Create .js file and named it as you wish:
- autocomplete.js
Add the scripts and styles
Add these lines of code in the functions.php file or the main file of the plugin.
<?php
// Localize the script with new data.
$ajax = [
'ajax_url' => admin_url( "admin-ajax.php" ),
'ajax_nonce' => wp_create_nonce('ajax-nonce'),
];
wp_enqueue_script('pe-autocomplete-search', get_stylesheet_directory_uri() . '/public/js/autocomplete.js',
['jquery', 'jquery-ui-autocomplete'], $this->version, true );
wp_localize_script( 'pe-autocomplete-search', 'ajax_url', $ajax );
$wp_scripts = wp_scripts();
wp_enqueue_style('pe-jquery-ui-css',
'//ajax.googleapis.com/ajax/libs/jqueryui/' . $wp_scripts->registered['jquery-ui-autocomplete']->ver . '/themes/smoothness/jquery-ui.css',
false, $this->version, false
);
The AJAX resuest
These lines of code should be also added to the functions.php file:
add_action('wp_ajax_nopriv_autocomplete_search', 'pe_autocomplete_search');
add_action('wp_ajax_autocomplete_search', 'pe_autocomplete_search');
function pe_autocomplete_search() {
check_ajax_referer('ajax-nonce', 'security');
$term = $_REQUEST['term'];
if (!isset($_REQUEST['term'])) {
echo json_encode([]);
}
$suggestions = [];
$query = new WP_Query([
's' => $term,
'posts_per_page' => 10,
]);
if ($query->have_posts()) {
while ($query->have_posts()) {
$query->the_post();
$suggestions[] = [
'id' => get_the_ID(),
'label' => get_the_title(),
'link' => get_the_permalink()
];
}
wp_reset_postdata();
}
echo json_encode($suggestions);
wp_die();
}
The input field
Add an input field anywhere you want on the front-end part of your website. Add a unique name and id.
<input type="text" name="pe_autocomplete_search" id="pe_autocomplete_search">
Main functionality
Add these lines of code to the autocomplete.js file:
jQuery(function($) {
$('#pe_autocomplete_search').autocomplete({
source: function(request, response) {
$.ajax({
dataType: 'json',
url: ajax_url.ajax_url,
data: {
term: request.term,
action: 'autocomplete_search',
security: ajax_url.site_nonce,
},
success: function(data) {
response(data);
}
});
},
select: function(event, ui) {
window.location.href = ui.item.link;
},
});
});
Let’s make it more interesting
Add a div element right under the input field. Here we will be displaying the result of our request. You can add a simple list or links.
<div class="pe-as-wrapper">
<input type="text" name="pe_autocomplete_search" id="pe_autocomplete_search">
<div class="results"></div>
</div>
Clear the div element before the AJAX is sent. When the request is successful, append the results of the new request.
jQuery(function($) {
$('#pe_autocomplete_search').autocomplete({
source: function(request, response) {
$.ajax({
dataType: 'json',
url: ajax_url.ajax_url,
data: {
term: request.term,
action: 'autocomplete_search',
security: ajax_url.site_nonce,
},
beforeSend: function () {
$('.pe-as-wrapper .results').html('');
},
success: function(data) {
// response(data);
for (let index = 0; index < data.length; ++index) {
let element = data[index];
$('.pe-as-wrapper .results').append('<a href="' + element.link + '">' + element.label + '</a>');
// ...use `element`...
}
}
});
},
select: function(event, ui) {
window.location.href = ui.item.link;
},
});
});
Of course, this needs some styling but you understand the basic idea.
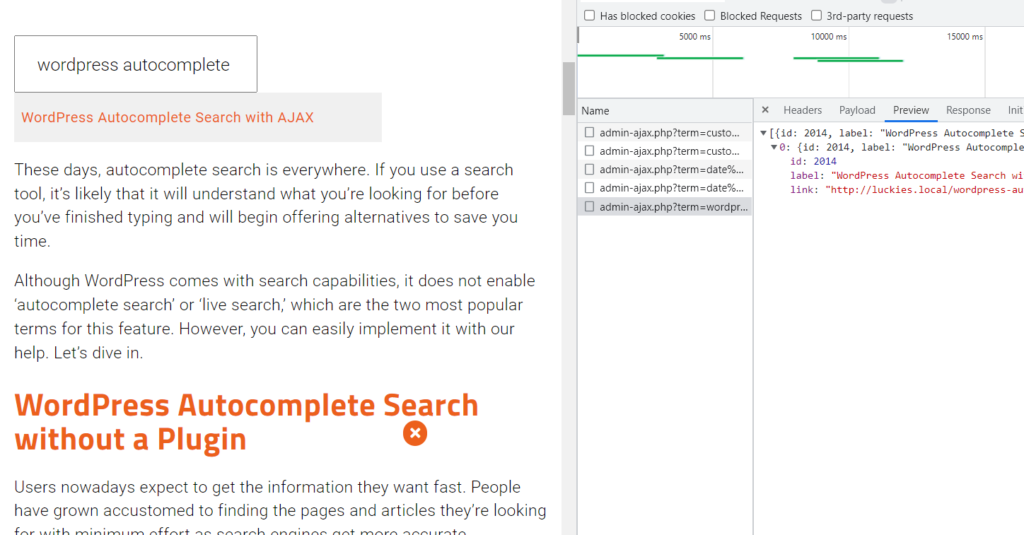