What are WordPress Transients and how to use them
set_transient is a WordPress function used to set a transient, which is a temporary value that can be stored in the database or in the server's memory for a specified amount of time.
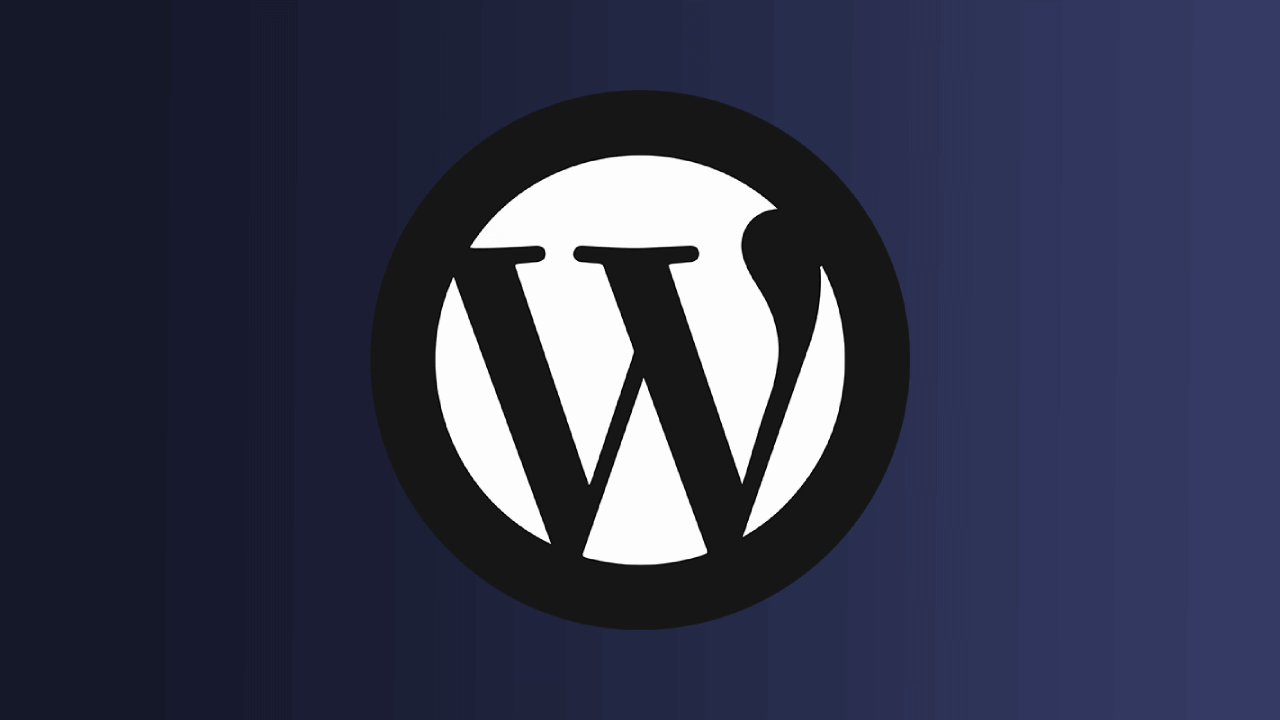
set_transient
is a WordPress function used to set a transient, which is a temporary value that can be stored in the database or in the server’s memory for a specified amount of time. This can be useful for caching data or for improving website performance by reducing the number of database queries.
The set_transient
function takes three parameters:
$transient
: A unique name for the transient.$value
: The value to store in the transient.$expiration
: The length of time, in seconds, that the transient should be stored. After this time has elapsed, the transient will be deleted.
Here’s an example of how to use set_transient
to store the result of a database query for 10 minutes:
$results = get_posts( array( 'post_type' => 'post' ) );
set_transient( 'recent_posts', $results, 10 * MINUTE_IN_SECONDS );
In this example, the get_posts
function is used to retrieve a list of recent posts, which is then stored in a transient named “recent_posts” for 10 minutes using set_transient
.
Later, you can retrieve the transient value using the get_transient
function, like this:
$recent_posts = get_transient( 'recent_posts' );
if ( false === $recent_posts ) {
$recent_posts = get_posts( array( 'post_type' => 'post' ) );
set_transient( 'recent_posts', $recent_posts, 10 * MINUTE_IN_SECONDS );
}
In this example, the get_transient
function is used to retrieve the value of the “recent_posts” transient. If the transient doesn’t exist or has expired, the get_posts
function is called to retrieve a new list of recent posts, which is then stored in the transient for another 10 minutes using set_transient
.
Here are a few more examples and some additional information about set_transient
:
- Caching API responses:
If you’re working with an API that provides data that changes infrequently, you can use set_transient
to cache the API response for a set period of time. Here’s an example:
function get_api_data() {
$data = get_transient( 'api_data' );
if ( false === $data ) {
$response = wp_remote_get( 'https://api.example.com/data' );
if ( is_wp_error( $response ) ) {
return false;
}
$data = json_decode( wp_remote_retrieve_body( $response ) );
set_transient( 'api_data', $data, 1 * HOUR_IN_SECONDS );
}
return $data;
}
In this example, get_api_data
checks to see if the API data is stored in a transient named “api_data”. If the transient exists, it returns the data. Otherwise, it retrieves the data from the API, stores it in the transient for one hour using set_transient
, and returns the data.
- Storing data for a short period of time:
set_transient
can also be useful for storing data temporarily, such as during a single request. For example:
function process_form_submission() {
// Process form submission and generate result
$result = 'success';
set_transient( 'form_result', $result, 30 ); // Store result for 30 seconds
wp_redirect( '/thank-you' ); // Redirect to thank-you page
exit;
}
In this example, process_form_submission
processes a form submission and generates a result. The result is stored in a transient named “form_result” using set_transient
for 30 seconds, after which it will be automatically deleted. The user is then redirected to a thank-you page.
- Using the
$expiration
parameter:
The $expiration
parameter of set_transient
can be set to any integer value, representing the number of seconds the transient should be stored. You can also use the built-in time constants like MINUTE_IN_SECONDS
, HOUR_IN_SECONDS
, DAY_IN_SECONDS
, etc. Here’s an example:
$value = 'Hello, world!';
set_transient( 'greeting', $value, 5 * MINUTE_IN_SECONDS );
In this example, the value “Hello, world!” is stored in a transient named “greeting” for five minutes using set_transient
. After five minutes, the transient will be automatically deleted.
We hope these examples and information help you understand how to use set_transient
in your WordPress development.
Was this post helpful? ( Answers: 0 )
Leave a comment
If you enjoyed this post or have any questions, please leave a comment below. Your feedback is valuable!