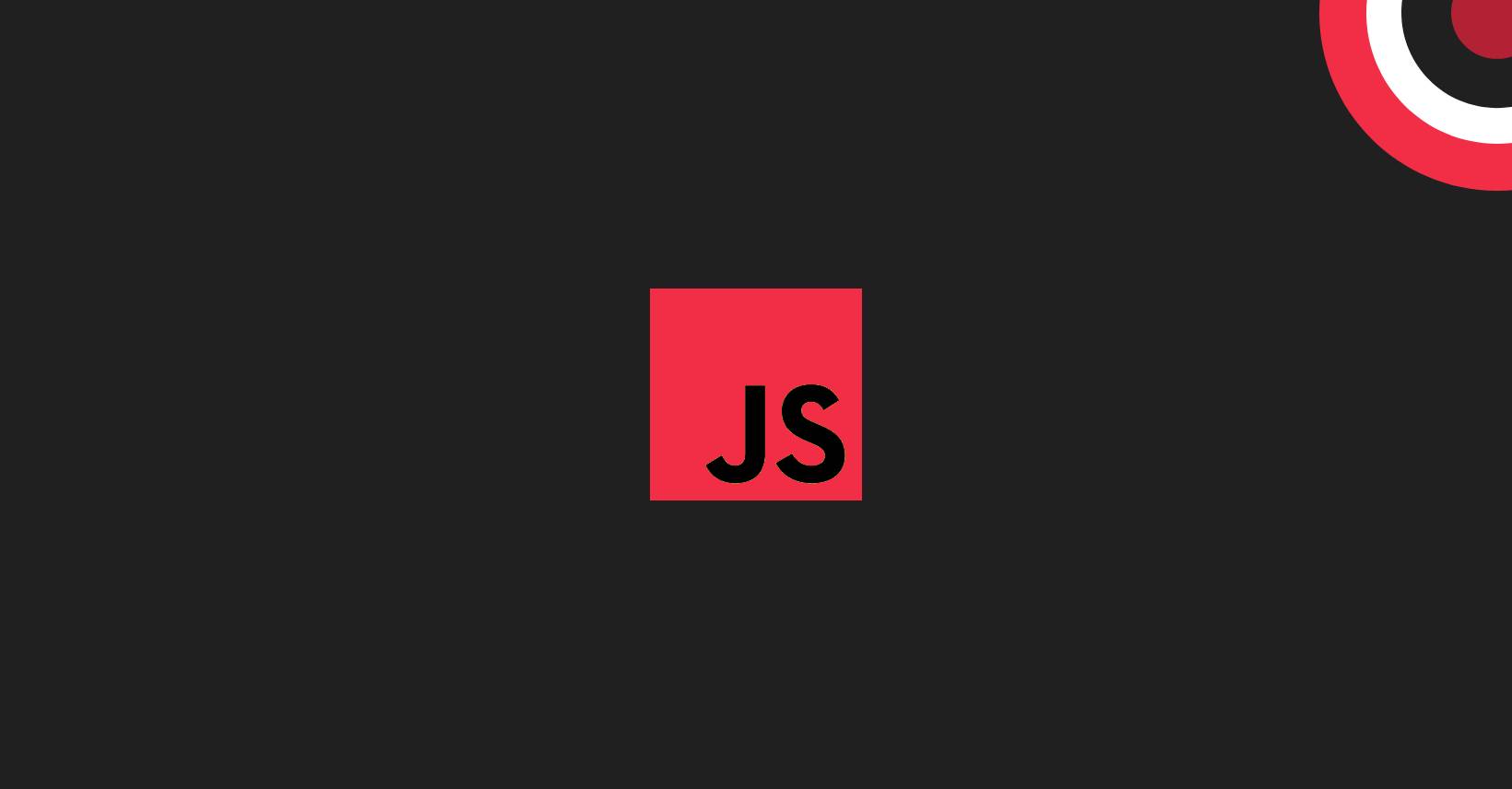
What are Arrow Functions in JavaScript and how to use them
In JavaScript, developers often strive to enhance code readability and efficiency. The arrow function syntax is a powerful tool that helps in this process.
In JavaScript, developers often strive to enhance code readability and efficiency. The arrow function syntax is a powerful tool that helps in this process. This article will explore the details of arrow functions, guide you through the seamless conversion of regular functions, and uncover the reasons why they are recommended over traditional functions.
Arrow functions were introduced in ECMAScript 6 (ES6) to provide a concise syntax for writing functions in JavaScript.
How to convert a regular function to an arrow function
Converting a regular function to an arrow function is a straightforward process. The basic transformation involves removing the function
keyword, adding an arrow (=>
) between the parameters and the function body, and eliminating unnecessary curly braces for single-line expressions.
function greet(name) {
return "Hello, " + name + "!";
}
const greet = name => "Hello, " + name + "!";
Why arrow functions are recommended over regular functions
1. Arrow Functions Are Better for Short Functions
Arrow functions excel in scenarios where brevity is key. Their concise syntax allows developers to express simple functions in a more compact and readable manner, reducing boilerplate code.
2. Arrow Functions Have an Implicit Return Statement
Unlike regular functions, arrow functions automatically return the result of the expression without the need for an explicit return
statement. This further contributes to code brevity.
3. Arrow Functions Don’t Have this
Binding
Arrow functions do not have their this
context. Instead, they inherit the this
value from the enclosing scope. This behavior simplifies the handling of this
in functions, especially within callbacks or event handlers.
When You Should Not Use Arrow Functions?
While arrow functions offer numerous advantages, they are not suitable for all scenarios. Avoid using arrow functions in the following situations:
- Methods in Objects: Arrow functions lack their
this
binding, making them unsuitable for methods within objects that need access to the object’s properties. - Constructors: Arrow functions cannot be used as constructors to create instances of objects.
Supported Browsers
Arrow functions are widely supported in modern browsers, including Chrome, Firefox, Safari, Edge, and others. Ensure that your target audience uses browsers that support ES6 features to leverage arrow functions in your code.
Transformation of a traditional function
Let’s break down the transformation of a traditional anonymous function into the simplest arrow function step-by-step. We’ll use a simple function that adds two numbers for this demonstration.
Step 1: Traditional Anonymous Function
// Traditional anonymous function
const add = function(a, b) {
return a + b;
};
Step 2: Remove the function
keyword
// Arrow function (step 1)
const add = (a, b) => {
return a + b;
};
Step 3: Remove curly braces for single-line expressions
// Arrow function (step 2)
const add = (a, b) => a + b;
At this point, the arrow function has been simplified to its most basic form. The removal of the function
keyword, curly braces, and the return
statement results in a concise and equivalent arrow function.
Step 4: Further Simplification for Single Parameters
// Arrow function (step 3)
const add = (a, b) => a + b;
// If there's only one parameter, you can omit the parentheses
const increment = a => a + 1;
For functions with a single parameter, you can omit the parentheses around the parameter, further simplifying the arrow function syntax.
Step 5: Final Step – The Simplest Arrow Function
// Arrow function (final step)
const add = (a, b) => a + b;
// If there's only one parameter, you can omit the parentheses
const increment = a => a + 1;
The final step represents the simplest form of an arrow function, showcasing the evolution from a traditional anonymous function to a concise arrow function. The syntax is minimal while maintaining clarity and readability.
Conclusion
In conclusion, arrow functions in JavaScript provide a concise and efficient syntax for writing functions, making the code more readable and maintainable. While they are recommended for short functions and scenarios where this
binding simplicity is crucial, it’s essential to be aware of their limitations and use cases where regular functions might be more appropriate. By mastering arrow functions, developers can enhance their coding proficiency and adapt to the evolving landscape of JavaScript programming.