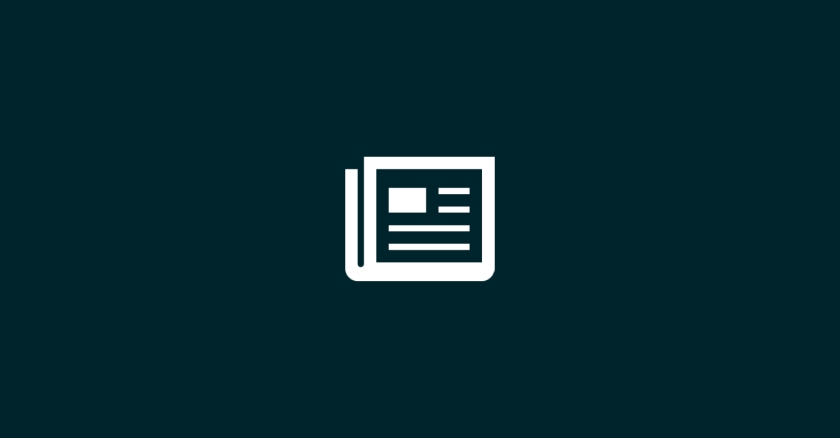
The Impact of Poor Variable Naming on Code Quality
Learn why proper variable naming is crucial for readability, maintainability, and fewer bugs in your code.
In programming, variable names are much more than just placeholders for values. They play a crucial role in making the code readable, maintainable, and free of errors. In this article, we’ll explore how poor variable naming can negatively affect code quality, and why it’s important to follow best practices for naming your variables.
Why Does Variable Naming Matter?
When you or another developer revisit code weeks, months, or even years after it’s been written, the first thing that should stand out is the variable names. Clear and meaningful names make it easier to understand the purpose of a variable without needing to dig through the entire codebase. Conversely, poor variable names can lead to confusion, bugs, and difficulty maintaining the code.
The Consequences of Poor Variable Naming
1. Decreased Readability: If a variable is named poorly, it becomes harder for anyone reading the code to immediately grasp what it represents. This can be especially frustrating when working with complex projects or when collaborating with others.
Example of Poor Naming:
let x = 5;
let y = 10;
let z = x + y;
console.log(z);
Without any context, x
, y
, and z
could represent anything. Wouldn’t it be clearer to name these variables something more descriptive?
Improved Naming:
let firstNumber = 5;
let secondNumber = 10;
let sum = firstNumber + secondNumber;
console.log(sum);
Now, the purpose of each variable is obvious, improving the code’s readability.
2. Increased Maintenance Effort: Code is often maintained or modified by different developers over time. When variables are poorly named, maintaining and refactoring the code becomes more challenging and error-prone. A variable with an unclear name may lead to misinterpretations, causing bugs or unintended side effects during updates.
Example of Poor Naming:
let temp = getUserDetails();
It’s unclear what temp
is storing or why. It could be user details, but we can’t be certain without looking at the entire code. A better name might be userDetails
.
Improved Naming:
let userDetails = getUserDetails();
3. Bug-Prone Code: Reusing variable names or giving variables names that imply a different data type can introduce bugs. For example, if you name a boolean variable isActive
, but assign it a number, anyone reading your code may be confused or expect a different behavior.
Example of Misleading Naming:
let isActive = 5;
This is misleading because isActive
sounds like a boolean, but it holds a number. Using more accurate names will prevent such confusion.
Improved Naming:
let activeUserCount = 5;
Best Practices for Naming Variables
To avoid the negative impact of poor variable naming, follow these best practices:
1. Be Descriptive: The name of a variable should describe its purpose. Avoid vague names like data
, info
, or temp
. Instead, use names that make the variable’s purpose clear.
let userAge = 25;let totalPrice = 100;
2. Follow Consistent Naming Conventions: Stick to one naming convention throughout your codebase. In JavaScript, the standard is camelCase for variable names, where the first word is lowercase and subsequent words are capitalized.
let totalAmount = 50;let userInput = 'Hello';
3. Use Meaningful Boolean Names: For boolean variables, prefix them with words like is
, has
, can
, or should
, to indicate that they store true/false values.
let isActive = true;let hasPermission = false;
4. Avoid Reusing Variable Names: Reusing variable names in the same scope can lead to bugs and confusion. Make sure each variable has a unique name that accurately reflects its content.
let totalAmount = 100;let itemCount = 5;
5. Be Consistent and Predictable: Your code should be easy to follow, so maintain consistency in naming conventions, capitalization, and naming patterns.
Conclusion
Variable naming may seem like a small detail, but it can have a profound impact on your code quality. Poorly named variables can lead to decreased readability, higher maintenance costs, and bug-prone code. By following best practices like being descriptive, consistent, and accurate, you can improve the clarity and maintainability of your code, making it easier to work with and debug. In the long run, investing time in proper variable naming is well worth the effort.