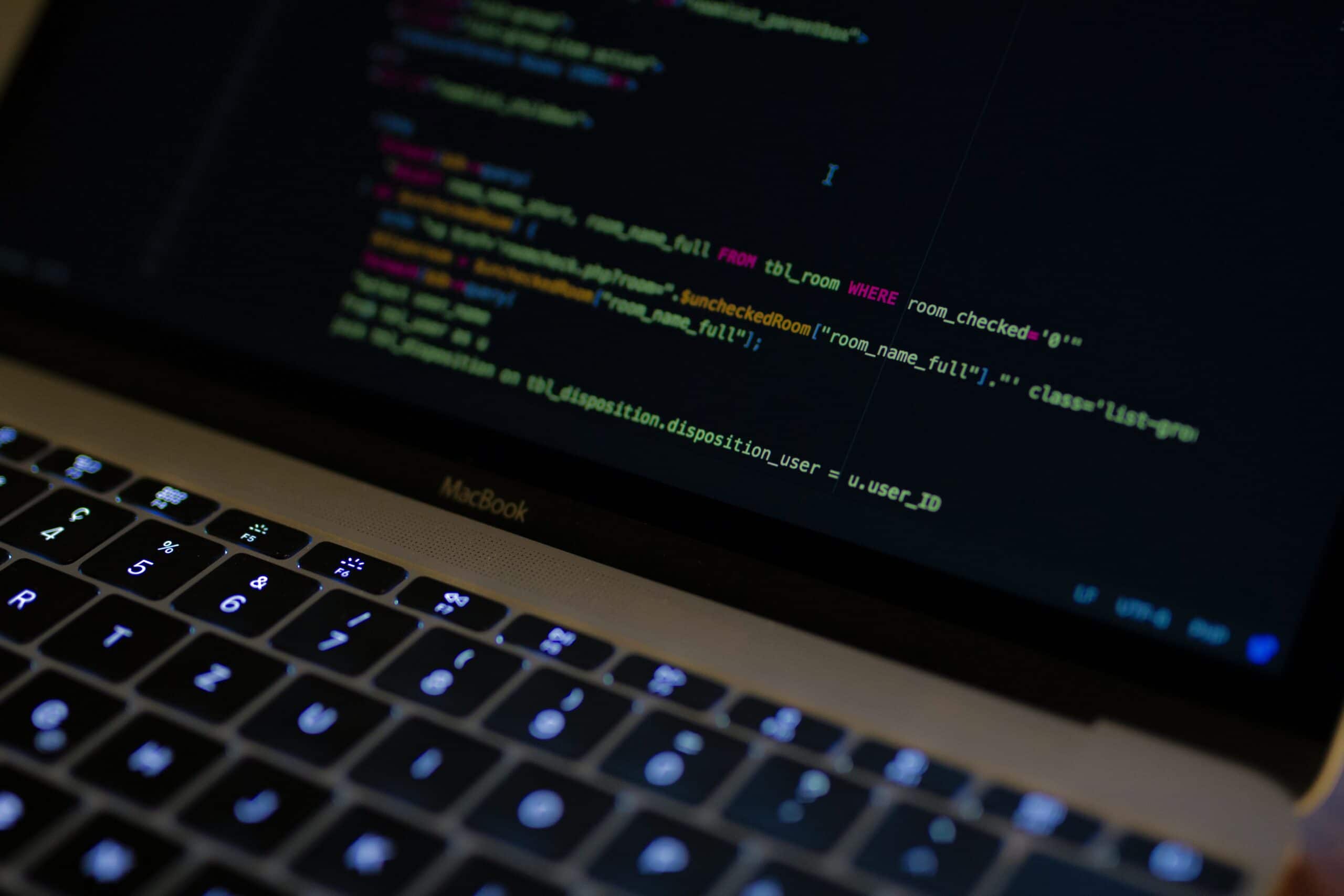
Subscription Form in WordPress
Add a subscription form on any page of your website or blog where the users can fill in the fields with their data to receive emails on topics related to their interests. Let's get started.
Add a subscription form on any page of your website or blog where the users can fill in the fields with their data to receive emails on topics related to their interests. Let’s get started.
1. Create the subscription form
<form id="pe_subscribe" method="post">
<label for="pe_email"></label>
<input name="pe_email" id="pe_email" placeholder="[email protected]">
<button type="submit" class="button">Subscribe
<i class="dashicons dashicons-arrow-right-alt"></i>
</button>
</form>
2. Include your script
wp_enqueue_script( 'pe-public', get_stylesheet_directory_uri() . '/assets/js/script.js', array('jquery'), '1.1.1', true );
// Localize the script with new data.
$ajax = array( 'ajax_url' => admin_url( "admin-ajax.php" ) );
wp_localize_script( 'pe-public', 'ajax_url', $ajax );
3. Edit your script file
jQuery(document).ready(function ($) {
let subscribe = $('#pe_subscribe');
subscribe.submit(function (e) {
e.preventDefault();
let email = $('#pe_email').val();
if(!isEmail(email)) {
console.log('Invalid email address!');
return;
}
$.ajax({
url: ajax_url.ajax_url,
type: 'POST',
data: { action: 'pe_subscribe', email: email },
beforeSend: function () {
// do something before ajax
}
}).success(function (data) {
// do something on ajax success
});
});
});
4. AJAX request
function pe_subscribe() {
$email = $_POST['email'];
$hash = wp_hash($email);
$headers = array('Content-Type: text/html; charset=UTF-8');
$data = [
'user_login' => $email,
'user_email' => $email,
'role' => 'subscriber',
'user_pass' => NULL,
'user_activation_key' => $hash
];
$thanks = 'Thank you for subscribing.';
$activated = 'The email is already activated.';
$message = '<div class="email-subscribe">';
$message .= '<div class="email-subscribe-logo">';
$message.= '<img src="' . site_url() . '/path/img/logo.png">';
$message .= '</div>';
$message .= '<div class="email-subscribe-text">';
$message .= '<p>Click here to activate your subscription.</p>';
$message .= '</div>';
$message .= '</div>';
$message .= '
<style>
.email-subscribe {
text-align: center;
}
.email-subscribe-logo {
display: block;
background-color: #000000;
padding: 1rem;
}
.email-subscribe-text {
display: block;
}
.email-subscribe-text p {
font-size: 20px;
color: #13202B;
}
.email-subscribe-text p a {
text-decoration: none;
color: #B87333;
}
</style>';
if(!email_exists($email)) {
$id = wp_insert_user($data);
wp_mail($email, 'Verify your subscription!', $message, $headers);
echo $thanks;
} else { echo $activated; }
wp_die();
}
add_action( 'wp_ajax_pe_subscribe', 'pe_subscribe' );
add_action( 'wp_ajax_nopriv_pe_subscribe', 'pe_subscribe' );
Add this code in the functions.php
file. After that, when the form is summited, a user will be created in your database. You can create an admin page where you can list and manage your subscribers.
5. Mailtrap
function mailtrap($phpmailer) {
$phpmailer->isSMTP();
$phpmailer->Host = 'smtp.mailtrap.io';
$phpmailer->SMTPAuth = true;
$phpmailer->Port = 2525;
$phpmailer->Username = '';
$phpmailer->Password = '';
}
add_action('phpmailer_init', 'mailtrap');
Use this tool to test if the emails are sent. After that, sign up to an email marketing tool (Sparkpost or Mailchimp). That way you’ll be able to send emails.
Don’t forget to create a function where you’ll list all of your subscribers and send the new post. You can use wp_mail
to do that.
$users = get_users( array( 'role__in' => array( 'subscriber', 'administrator' ) ) );
foreach ($users as $user ) {
// send the the post with wp_mail
}