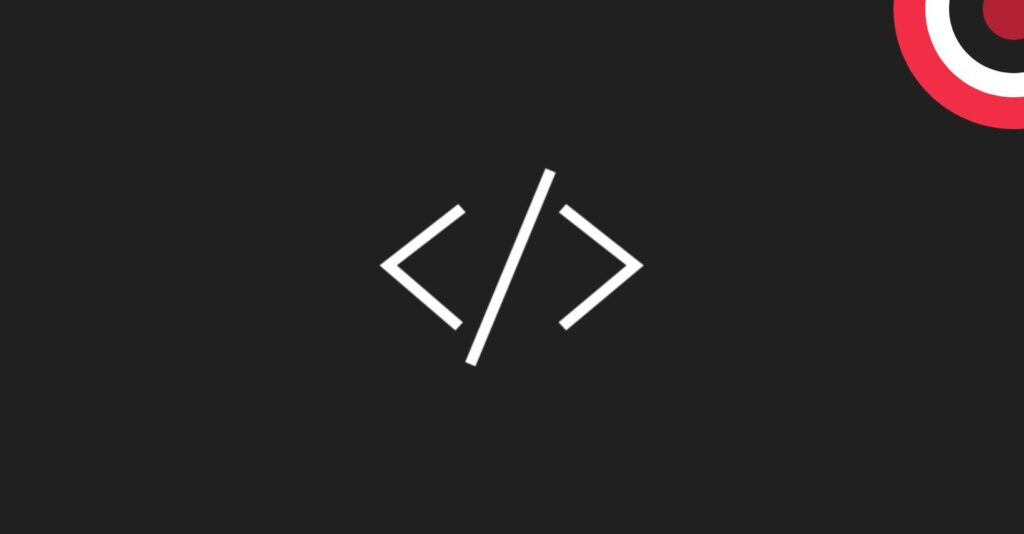
One-liners in JavaScript that will make you code like a pro
JavaScript one-liners are concise, powerful snippets of code that can significantly enhance your coding efficiency and elevate you to a professional level.
Table of Contents
- Why use one-liners?
- Conciseness
- Readability
- Performance
- Essential JavaScript one-liners
- Generate a random string
- Uppercase the first character of each word in a string
- Remove duplicates from an array
- Get unique values from two arrays
- Convert a string to camelCase
- Convert hexadecimal to rgba
- Find the average of an array of numbers
- Remove all whitespace from a string
- Add an element to the beginning/end of an array and concatenate two arrays
- Sort an array in ascending order
- Remove the first/last element of an array and an element from an array at a specific index
- Access a character in a string
- Conclusion
JavaScript one-liners are concise, powerful snippets of code that can significantly enhance your coding efficiency and elevate you to a professional level. This comprehensive guide explores the art of crafting impactful one-liners, providing you with 20 essential examples to supercharge your JavaScript coding experience.
Why use one-liners?
- Conciseness
- Readability
- Performance
Conciseness
One-liners offer a concise way to achieve complex tasks, reducing the need for lengthy code blocks.
Readability
Well-crafted one-liners can enhance code readability, making it easier for you and other developers to understand.
Performance
Sometimes, one-liners can outperform traditional approaches, leading to more efficient code execution.
Essential JavaScript one-liners
Generate a random string
const randomString = () => Math.random().toString(36).slice(2);
console.log( randomString() ); // kbbfubew9d
console.log( randomString() ); // u2ihqnwu9n
It uses Math.random()
to generate a random floating-point number between 0 and 1, then converts it to a base-36 string using toString(36)
, and finally, slice(2)
is used to remove the “0.” prefix from the string. This results in a random alphanumeric string.
Uppercase the first character of each word in a string
const string = 'Uppercase the first character of each word in a string.';
const uppercaseWords = (str) => str.replace(/^(.)|\s+(.)/g, (c) => c.toUpperCase())
console.log( uppercaseWords(string) ); // 'Uppercase The First Character Of Each Word In A String.'
This code takes a string and transforms it by capitalizing the first character of each word. The input string, in this case, is ‘Uppercase the first character of each word in a string.’ The uppercaseWords
function achieves this transformation using the replace
method with a regular expression. The regular expression /^(.)|\s+(.)/g
captures two patterns: the first character of the string or any character that follows a space. The replacement function (c) => c.toUpperCase()
is then applied to each matched pattern, converting the matched character to its uppercase equivalent. As a result, the final output, obtained by calling uppercaseWords(string)
, is ‘Uppercase The First Character Of Each Word In A String.’ where the first letter of each word has been capitalized. The regular expression and the replacement function work together to efficiently achieve the desired uppercase transformation for each word in the given string.
Remove duplicates from an array
const array = [1, 2, 3, 4, 2, 5, 6, 3, 7, 8, 9, 1];
const uniqueArray = [...new Set(array)];
console.log(uniqueArray);
The code snippet is a concise one-liner function in JavaScript that removes duplicates from an array using the Set
data structure.
Get unique values from two arrays
const getUniqueValues = (arr1, arr2) => [...new Set([...arr1, ...arr2])];
const array1 = [1, 2, 3, 4];
const array2 = [3, 4, 5, 6];
const uniqueValues = getUniqueValues(array1, array2);
console.log( uniqueValues ); // [1, 2, 3, 4, 5, 6]
If you want to get unique values from two arrays in JavaScript, you can use the Set
data structure along with the spread operator.
Convert a string to camelCase
const toCamelCase = (str) => str.trim().replace(/[-_]\w/g, match => match.charAt(1).toUpperCase());
console.log( toCamelCase( "convert_this_string" ) ); // convertThisString
console.log( toCamelCase("-convert-a-string") ); // ConvertAString
console.log( toCamelCase("background-color") ); // backgroundColor
str
: This is the input string that you want to convert to camelCase..replace(/[-_]\w/g, match => match.charAt(1).toUpperCase())
: This part of the code uses thereplace
method along with a regular expression to find hyphens (-
) or underscores (_
) followed by a word character (\w
). For each match, it converts the matched character to uppercase. This effectively removes the hyphen or underscore and capitalizes the next character.
Convert hexadecimal to rgba
const hexToRgba = hex => {
const [r, g, b] = hex.match(/\w\w/g).map(val => parseInt(val, 16))
return `rgba(${r}, ${g}, ${b}, 1)`;
}
console.log( hexToRgba('#000000') ); // rgba(0, 0, 0, 1)
console.log( hexToRgba('#ff007f') ); // rgba(255, 0, 127, 1)
hex
: This is the input hexadecimal color code passed to the function.hex.match(/\w\w/g)
: This uses a regular expression to match pairs of alphanumeric characters in the hexadecimal code. It returns an array with two-character substrings..map(val => parseInt(val, 16))
: This maps each two-character substring to its decimal equivalent usingparseInt
with base 16. This converts the hexadecimal values to decimal values for red (r), green (g), and blue (b) components.const [r, g, b] = ...
: This destructures the resulting array into variables for red, green, and blue components.return
rgba(${r}, ${g}, ${b}, 1);
: This constructs and returns the RGBA string with the calculated red, green, and blue values, setting the alpha value to 1 (fully opaque).
Find the average of an array of numbers
const findAverage = arr => arr.reduce((sum, num) => sum + num, 0) / arr.length;
const numbers = [10, 20, 30, 40, 80];
const average = findAverage(numbers);
console.log(average); // Output: 36
arr
: This is the input array of numbers for which you want to find the average.arr.reduce((sum, num) => sum + num, 0)
: Thereduce
method is used to sum up all the elements in the array./ arr.length
: The sum is then divided by the length of the array to get the average.
Remove all whitespace from a string
const removeWhitespace = str => str.replace(/\s+/g, '');
const stringWithWhitespace = ' remove all whitespace from a string ';
const stringWithoutWhitespace = removeWhitespace(stringWithWhitespace);
console.log(stringWithoutWhitespace); // Output: 'removeallwhitespacefromastring'
str
: This is the input string from which you want to remove whitespace.str.replace(/\s+/g, '')
: Thereplace
method is used with a regular expression (/\s+/g
) to match all whitespace characters in the string. Theg
flag ensures that the replacement is applied globally throughout the string. The replacement value is an empty string (''
), effectively removing all whitespace.
Add an element to the beginning/end of an array and concatenate two arrays
const arr1 = [1, 2, 3, 4];
const arr2 = [...arr1, 5];
const arr3 = [6, ...arr2];
console.log(arr2); // (5) [1, 2, 3, 4, 5]
console.log(arr3); // (6) [6, 1, 2, 3, 4, 5]
const newArray = [...arr2, ...arr3];
console.log( newArray ); // (11) [1, 2, 3, 4, 5, 6, 1, 2, 3, 4, 5]
const arr2 = [...arr1, 5];
: This line creates a new arrayarr2
by spreading the elements ofarr1
and adding5
at the end. The result is an array[1, 2, 3, 4, 5]
.const arr3 = [6, ...arr2];
: This line creates another new arrayarr3
by spreading the elements ofarr2
and adding6
at the beginning. The result is an array[6, 1, 2, 3, 4, 5]
.
This usage of the spread operator allows for concise and expressive array manipulation, enabling the creation of new arrays based on existing ones. It’s a powerful feature in modern JavaScript for working with arrays.
Sort an array in ascending order
const arr1 = [1, 2, 3, 4];
const arr2 = [...arr1, 5];
const arr3 = [6, ...arr2];
console.log(arr2); // (5) [1, 2, 3, 4, 5]
console.log(arr3); // (6) [6, 1, 2, 3, 4, 5]
const newArray = [...arr2, ...arr3];
console.log( newArray ); // (11) [1, 2, 3, 4, 5, 6, 1, 2, 3, 4, 5]
const sortedArray = newArray.sort((a, b) => a - b);
console.log( newArray ); // (11) [1, 1, 2, 2, 3, 3, 4, 4, 5, 5, 6]
const arr2 = [...arr1, 5];
: Creates a new arrayarr2
by spreading the elements ofarr1
and adding5
at the end.const arr3 = [6, ...arr2];
: Creates another new arrayarr3
by spreading the elements ofarr2
and adding6
at the beginning.const newArray = [...arr2, ...arr3];
: Combinesarr2
andarr3
into a single arraynewArray
using the spread operator.const sortedArray = newArray.sort((a, b) => a - b);
: Sorts the elements ofnewArray
in ascending order using thesort
method with a comparator function.
Note that the sort
method modifies the array in place, so after sorting, both sortedArray
and newArray
reference the same sorted array. If you want to keep the original array unchanged, you might want to create a copy before sorting.
Remove the first/last element of an array and an element from an array at a specific index
const arr4 = [1, 2, 3, 4, 5];
const [first, ...rest] = arr4;
const lastElement = arr4.pop();
const arr5 = arr4.slice(1); // argument to the slice() method is the index
console.log(first); // Output: 1
console.log(rest); // Output: (4) [2, 3, 4, 5]
console.log(lastElement); // Output: 5
console.log(arr5); // Output: (3) [2, 3, 4]
const indexToRemove = 2; // index of 4 ( 0, 1, 2 )
arr5.splice(indexToRemove, 1); // second parameter 1 specifies that only one element should be removed
console.log(arr5);
const [first, ...rest] = arr4;
: Uses array destructuring to assign the first element ofarr4
to the variablefirst
, and the rest of the elements to the arrayrest
.const lastElement = arr4.pop();
: Removes the last element fromarr4
using thepop
method and assigns it to the variablelastElement
.const arr5 = arr4.slice(1);
: Creates a new arrayarr5
by slicingarr4
starting from index 1. This effectively excludes the first element.arr5.splice(indexToRemove, 1);
: Uses thesplice
method to remove one element fromarr5
at the specified index (indexToRemove
).
These operations allow you to manipulate arrays in various ways, including extracting elements, creating new arrays, and modifying existing ones.
Access a character in a string
const str = "Access a character in a string!";
const char = str.charAt(4);
console.log(char); // Output: s
str.charAt(4)
: ThecharAt
method is used to retrieve the character at the specified index within the string. In this case, it gets the character at index 4, which is “s”.console.log(char)
: Prints the extracted character to the console.
So, the output of this code will be “s” since it is the character at index 4 in the given string “Access a character in a string!”.
Conclusion
Using one-liners in your JavaScript code not only boosts your coding efficiency but also enhances the readability and maintainability of your code.