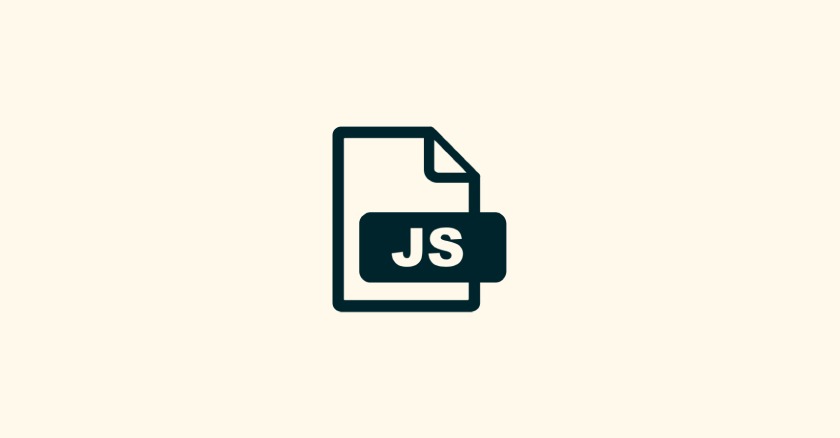
Unleash the hidden power of JavaScript generators
Learn how JavaScript generators simplify asynchronous tasks and iterators, allowing for efficient control flow and lazy execution.
JavaScript generators are a powerful feature introduced in ES6 that allows developers to create functions capable of pausing and resuming execution. They offer a cleaner, more manageable way to handle asynchronous code and iterators, making them essential for modern JavaScript development.
What are JavaScript Generators?
JS generators are functions that return an iterator object, which can be used to control the function’s execution. Unlike regular functions that run from start to finish, generators can pause their execution at any point, yielding control back to the caller. This makes JS generators highly useful for dealing with sequences of data or asynchronous operations.
How Do JavaScript Generators Work?
JS generators are declared using the function*
syntax. Inside the generator function, the yield
keyword is used to pause execution and return a value. Each time the next()
method is called on the generator, it resumes execution until the next yield
or return
statement is encountered.
Here’s a simple example of how JS generators work:
function* simpleGenerator() {
yield 'First step';
yield 'Second step';
return 'Finished';
}
const generator = simpleGenerator();
console.log(generator.next().value); // 'First step'
console.log(generator.next().value); // 'Second step'
console.log(generator.next().value); // 'Finished'
As seen in the example, calling next()
resumes the function from where it was paused.
Real-World Examples
In practical scenarios, JS generators are often used to create iterators or manage asynchronous code in a cleaner, more readable way. Here’s an example of a generator that simulates data fetching:
function* fetchData() {
const data = yield fetch('https://api.example.com/data');
console.log(data);
}
const generator = fetchData();
const promise = generator.next().value;
promise.then(response => generator.next(response));
In this example, the generator pauses after requesting data from an API, waiting until the data is fetched before continuing execution. This asynchronous flow mimics the behavior of async/await
but offers more granular control over execution.
Why Use JavaScript Generators?
JS generators shine in scenarios that involve iterating over data or managing asynchronous code. They provide lazy execution, meaning they generate values on demand rather than calculating everything upfront. This can make your code more efficient, especially when dealing with large datasets or infinite sequences.
Use Cases for Generators
JavaScript generators are versatile and can be applied to various development challenges. Some common use cases include:
- Data Streams: Process incoming data in chunks, such as reading large files, one piece at a time.
- Lazy Evaluation: Handle large datasets by generating values only when needed, reducing memory consumption.
- Asynchronous Control: Manage complex async workflows, such as making multiple API requests in sequence or dealing with event-based tasks.
- Custom Iterators: Build sophisticated iteration logic that goes beyond simple loops, ideal for complex data structures or sequences.
Asynchronous Operations with JS Generators
One of the most significant advantages of JavaScript generators is their ability to handle asynchronous tasks in a synchronous-like manner. When combined with promises, generators can manage complex asynchronous flows more cleanly.
For example, you can use a generator to make an asynchronous request, yielding control back to the caller until the request is complete:
function* fetchData() {
const data = yield fetch('https://api.example.com/data');
console.log(data);
}
const generator = fetchData();
const promise = generator.next().value;
promise.then(response => generator.next(response));
This pattern simplifies error handling and control flow compared to traditional callback methods.
Conclusion
JS generators are a game-changer for developers, offering a powerful way to handle iteration and asynchronous tasks efficiently. Whether you’re managing large datasets or dealing with complex async flows, learning to use JavaScript generators can greatly improve your code’s readability and performance.