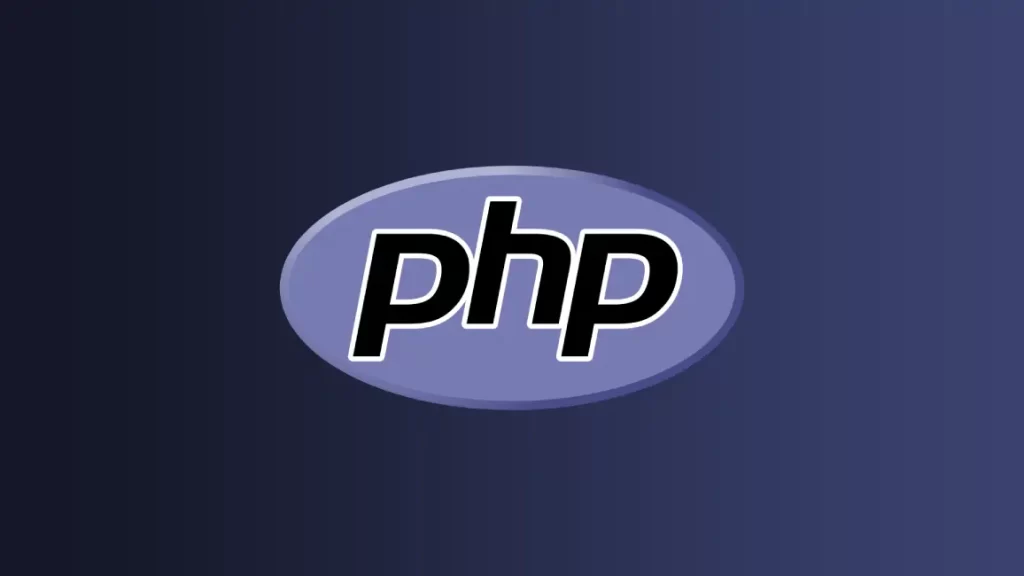
Overview of debugging tools and techniques in PHP
Debugging tools and techniques play a vital role in identifying and resolving issues in PHP applications efficiently.
Debugging tools and techniques play a vital role in identifying and resolving issues in PHP applications efficiently. Here’s an overview of some commonly used debugging tools and techniques.
Error Reporting and Logging
PHP Error Reporting
Configuring PHP’s error reporting settings allows you to display or log errors, warnings, and notices. It helps identify syntax errors, uninitialized variables, and other runtime issues.
Logging
Implementing a logging mechanism enables you to capture and analyze application events, errors, and debugging information. Popular logging libraries in PHP include Monolog and Log4php.
var_dump() and print_r()
These built-in PHP functions help inspect variables by displaying their values and data types. They are useful for quickly examining variable contents and structures during debugging.
Debugging Extensions
Xdebug
A powerful PHP extension that provides advanced debugging capabilities, including breakpoints, stack traces, variable inspection, and remote debugging.
Zend Debugger
Another PHP extension that supports remote debugging, breakpoints, and variable inspection. It integrates well with IDEs like Zend Studio.
Integrated Development Environments (IDEs)
A popular PHP IDE that offers comprehensive debugging features, including step-by-step execution, breakpoints, watches, variable inspection, and code coverage analysis.
Visual Studio Code (with PHP extensions)
VS Code, a lightweight and extensible code editor, can be enhanced with PHP extensions like Xdebug to enable debugging functionalities.
Step-by-Step Execution
Setting breakpoints in the code allows you to pause the execution at specific lines and inspect variables, stack traces, and program flow. This technique helps identify the state of the application at different stages and locate problematic areas.
Remote Debugging
Remote debugging allows you to debug PHP applications running on remote servers, virtual machines, or containers. Tools like Xdebug and DBGp protocol facilitate remote debugging by establishing a connection between the IDE and the remote environment.
Profiling
Profiling tools analyze the performance of PHP applications, identify bottlenecks, and optimize code. Xdebug’s profiler and specialized profiling tools like Blackfire.io provide insights into function call times, memory usage, and execution paths.
Debugging Frameworks:
Laravel Debugbar
A debugging toolbar for Laravel that provides real-time information about queries, views, route details, and more.
Symfony VarDumper
A component in the Symfony framework that enhances variable inspection and debugging with features like pretty-printing, nested data exploration, and detailed debug information.
Error Tracking and Monitoring
Tools like Sentry, Bugsnag, or Rollbar offer error tracking and monitoring services. They capture and report errors and exceptions, allowing you to identify and fix issues in production environments.
Code Profiling and Coverage
Tools like Xdebug’s code coverage, PHP_CodeCoverage, or PHPUnit help measure the effectiveness of unit tests by indicating which parts of the code have been executed. It aids in identifying untested areas and improving overall code coverage.
These are just some of the many debugging tools and techniques available in the PHP ecosystem.
The choice of tools and techniques depends on the specific requirements, preferences, and development environment.
Employing the right combination of tools and techniques can significantly enhance the debugging process, accelerate issue resolution, and improve the overall quality of PHP applications.