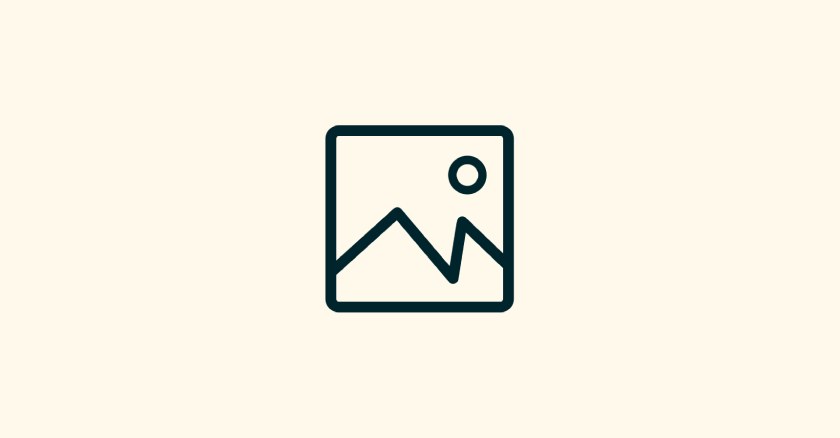
Lesson 1.3: Running Your First Node.js Program
Table of Contents Setting Up a Node.js Project Folder2. Creating Your First Node.js File3. Writing Your First Node.js Program4. Running the Program5. Understanding the Code6. Experimenting with More CodeSummarySetting Up a Node.js Project Folder 2. Creating Your First Node.js File 3. Writing Your First Node.js Program In app.js, write the following code: 4. Running the […]
Setting Up a Node.js Project Folder
- Create a New Folder: Start by creating a new folder where you’ll store your Node.js files. This will be your project’s root directory.
- For example, create a folder named
my_first_node_app
.
- For example, create a folder named
- Open Your Terminal: Open your terminal (or command prompt on Windows) and navigate to your new folder using the
cd
command: cd path/to/my_first_node_app
2. Creating Your First Node.js File
- Create a New File: Inside the
my_first_node_app
folder, create a file namedapp.js
. - Open the File in a Code Editor: You can use any text editor, but VS Code is a popular choice for Node.js development.
3. Writing Your First Node.js Program
In app.js
, write the following code:
console.log("Hello, Node.js!");
4. Running the Program
- Open the Terminal: Make sure your terminal is still open in the
my_first_node_app
directory. - Run the Code: Use the
node
command to execute your file: node app.js
- Check the Output: You should see the following output in the terminal:
Hello, Node.js!
This confirms that Node.js is correctly installed and working on your system.
5. Understanding the Code
- console.log(): This is a JavaScript function that prints a message to the console. In this case, it’s printing “Hello, Node.js!”.
- The
node
Command: Using thenode
command followed by the file name (in this case,app.js
) tells Node.js to run the code in that file.
6. Experimenting with More Code
Try adding some additional code to explore what Node.js can do. Here’s an example:
const os = require("os");
console.log("Hello, Node.js!");
console.log("Your system’s architecture is:", os.arch());
console.log("Your system’s platform is:", os.platform());
console.log("Available memory:", os.freemem());
Explanation: This code uses the built-in os
module to get system information, such as the architecture and platform of your operating system, along with available memory.
Run the file again using the node app.js
command, and observe how it prints system information to the console.
Summary
In this lesson, you created your first Node.js program, ran it, and saw its output in the terminal. This foundational step gives you the confidence that your Node.js setup is working correctly, and it introduces the basics of running and debugging simple Node.js scripts.