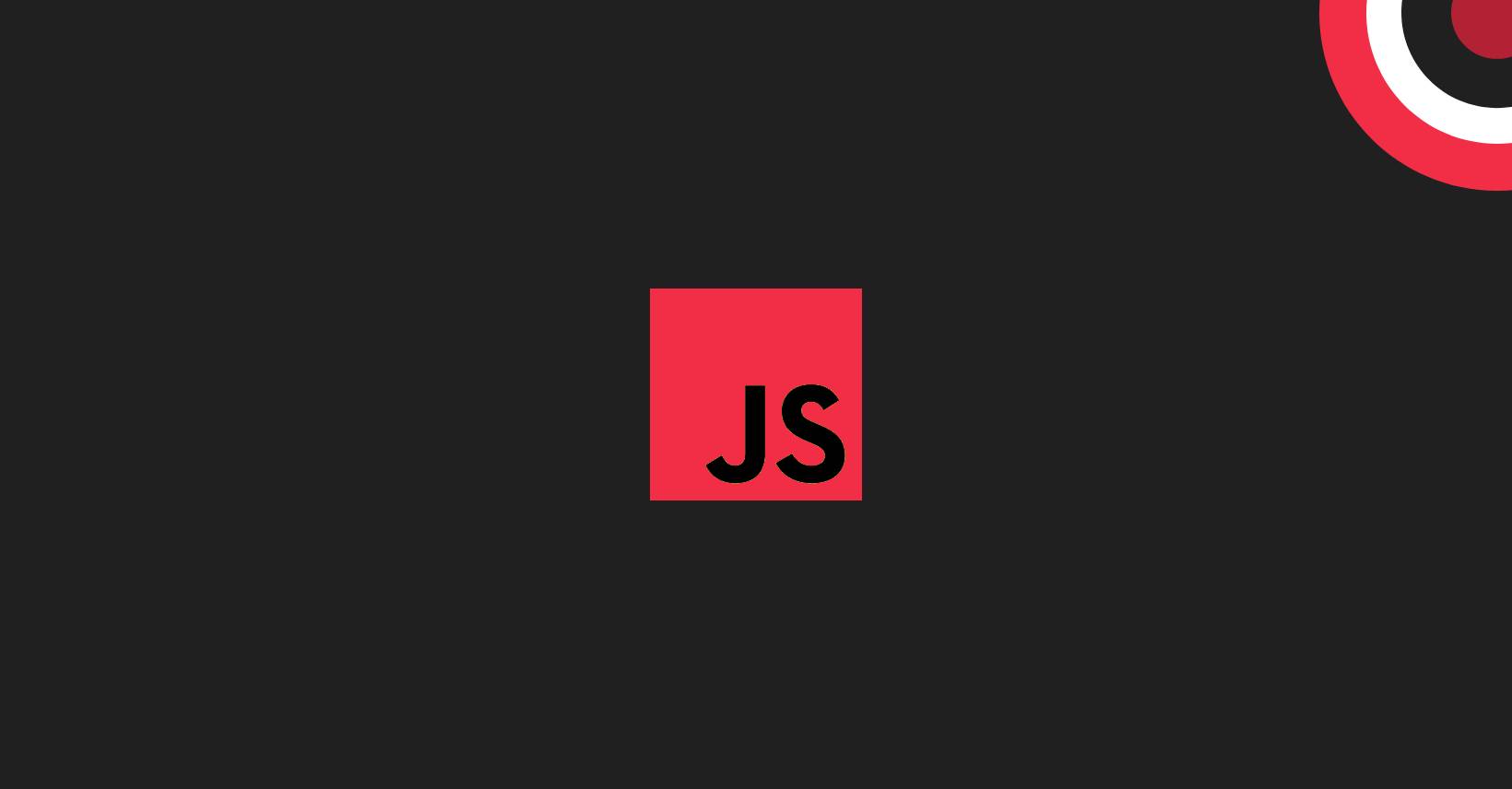
JavaScript in HTML: Unleash the Potential of Your Code with Placement Tips
Learn where to place your JavaScript code in HTML for optimal performance and organization. Explore traditional and modern techniques to make your web pages sing.
Confused about where to put your JavaScript code in your HTML document? You’re not alone! This guide unveils the mysteries of JavaScript placement, offering real-world examples and modern best practices to take your web development from beginner to boss.
The Script Tag: Your Code’s Cozy Home
Imagine the script tag as a cozy apartment for your JavaScript code. Anything inside its <script> and </script> walls gets interpreted as magic spells – I mean, JavaScript – by the browser. And guess what? You can place this apartment practically anywhere within the HTML building!
Top Floor or Basement? Head vs. Body Placement
Technically, the head section is the ideal spot for your script tag. It’s like keeping your code neatly organized away from the main living space (the body). But, in many cases, you’ll find the script tag chilling at the bottom floor – the end of the body. Why?
The Rendering Dance: Timing Your Code Right
The browser reads HTML like a curious explorer, line by line. When it encounters JavaScript, it pauses the entire rendering party. It then carefully executes the code before resuming its exploration. This is crucial because your JavaScript might need to interact with elements already rendered in the body.
Example Time: Targeting Code Elements
In the example below, the script hunts for <code/> elements. But for the browser to find them, they need to be rendered first! That’s why the script tag hangs out at the bottom – after all the code elements have taken their place.
<!DOCTYPE html>
<html>
<head>
<title>Targeting Code Elements</title>
</head>
<body>
<p>Here's a paragraph with some <code>inline code</code> for demonstration.</p>
<code>This is a standalone code block.</code>
<script>
// Select all code elements once they are rendered
const codeElements = document.querySelectorAll('code');
// Customize code elements with JavaScript
codeElements.forEach(codeElement => {
codeElement.style.backgroundColor = 'lightblue'; // Highlight code elements
codeElement.style.border = '1px solid #ccc'; // Add a border
codeElement.addEventListener('click', () => {
codeElement.style.color = 'red'; // Change text color on click
});
});
</script>
</body>
</html>
Key Points:
- Script placement matters when interacting with elements that need to be rendered first.
- Use
document.querySelectorAll
to target specific elements based on their tags or selectors. - JavaScript can dynamically modify the appearance and behavior of elements in the browser.
Modern Magic: Beyond the Script Tag Basement
While this example showcases the “old-school” approach, we have better tools now! Upcoming lessons will reveal modern JavaScript loading techniques that give you more control over how and when your code runs.
Key Takeaways:
- The script tag houses your JavaScript code.
- You can place it anywhere in the HTML document.
- The
head
section is preferred for cleaner organization. - Body placement can be useful for specific scenarios like element manipulation.
- Modern loading techniques offer more advanced control.
Remember: Experiment, explore, and don’t be afraid to break the “basement script” mold! With the right tools and knowledge, you’ll have your JavaScript code living comfortably – and working wonders – in any corner of your HTML document.