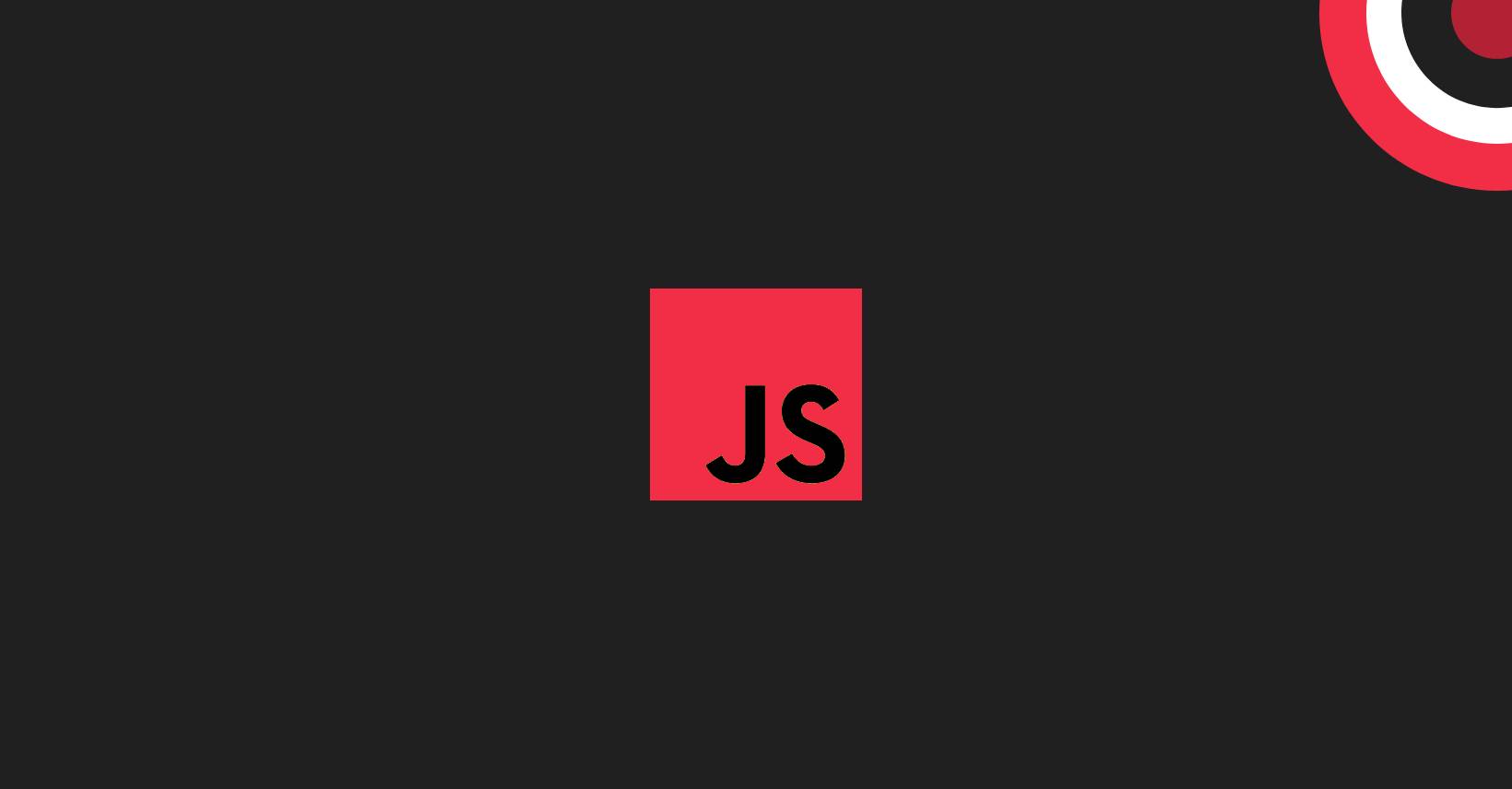
JavaScript Basics: A Deep Dive into Syntax and Style
Welcome, aspiring JavaScript wizards, to an in-depth exploration of the language's foundational elements!
Welcome, aspiring JavaScript wizards, to an in-depth exploration of the language’s foundational elements! Buckle up, because we’re about to delve into the realm of syntax, comments, whitespace, semicolons, and quotation marks – the building blocks of your JavaScript scripts.
1. Top-Down Flow: A Scriptural Sequence
Imagine your JavaScript code as a story, unfolding line by line. That’s precisely how the browser reads it – from top to bottom. So, a golden rule emerges: define all variables, functions, objects, and any other entities before you use them. Think of it as introducing your characters before they take center stage in the narrative. This ensures the browser has encountered everything before you call upon it, preventing any unexpected plot twists.
// Define our hero: a constant backpack with exciting features
const backpack = {
color: "gray",
lidOpen: false,
name: "Everyday Backpack",
};
// Introduce the sidekick: a function to toggle the lid
function toggleLid(lidStatus) {
backpack.lidOpen = lidStatus;
console.log("Lid status changed!");
}
2. The Power of Comments: Speaking to Your Future Self (and Others)
Think of comments as whispered notes left for your future self or fellow JavaScript adventurers. They explain the “why” behind your code, like helpful annotations in the margins of a well-loved book. Browsers simply ignore these green-tinted whispers, but for humans, they’re invaluable. Single-line comments start with “//” and span the remaining line, while multi-line comments use “/* /” to embrace your explanatory text like a warm hug. Advanced editors like VS Code even offer shortcuts like “/ * */” to generate detailed comment templates, capturing function parameters and data types for enhanced clarity. Remember, good comments don’t just document your code – they illuminate it.
// This comment explains what the next line does
const isLidOpen = backpack.lidOpen;
// Multi-line comment describing a complex function
/*
This function checks the backpack's contents and updates the HTML.
*/
function updateBackpack(update) {
// ... code that modifies the page based on the update ...
}
3. Whitespace: More Than Just Empty Space
While to the browser, whitespace-like spaces and tabs might seem as empty as a forgotten attic, to us humans, it’s the architect of code readability. Proper indentation reflects hierarchy, visually separating your script’s chapters and subplots. Imagine a messy manuscript crammed onto a single line – that’s what unformatted code feels like. Embrace the power of indentation to structure your script like a well-organized novel, making it easier for you and others to navigate its depths.
// Indented code block showing the backpack's contents
if (isLidOpen) {
console.log("The " + backpack.color + " " + backpack.name + " is open!");
} else {
console.log("The " + backpack.name + " is closed.");
}
4. Semicolons: A Matter of Preference, Not Law
The semicolon debate – to add or not to add – has ignited countless campfire discussions amongst developers. Fear not, for the truth is liberating: JavaScript itself doesn’t care. Semicolons act as sentence endings, but whether you sprinkle them throughout your code is purely a matter of personal style and coding standards. Tools like ESLint and Prettier can be your semicolon champions, enforcing consistency across your scripts, like trusty editors ensuring all sentences in your novel end with proper punctuation.
5. Quotation Marks: A Tale of Two Styles
Just like fashion trends, JavaScript embraces diversity in quotation marks. Single quotes (”) or double quotes (“”) – the choice is yours, and different coding standards may dictate a preferred style. Consistency is key, so pick your side and let the tools like ESLint and Prettier handle the rest. Remember, the true magic lies in the code you craft within those quotes, not the marks themselves.
console.log("My " + backpack.name + " is full of surprises!");
console.log('I can change the color to "' + backpack.color + '" anytime.');
Both styles achieve the same goal, so focus on expressing your code clearly and consistently, letting the quotation marks simply frame your words.
Beyond the Basics: A Journey of Continuous Exploration
This is just the first chapter in your JavaScript adventure. As you delve deeper, you’ll encounter advanced concepts like data types, operators, control flow, and functions. Each element adds complexity and power to your script, like mastering new chords to unleash musical symphonies of code. Embrace the learning journey, experiment, and never hesitate to seek guidance from fellow developers and online resources. Remember, the JavaScript community is always there to cheer you on as you write your own unique code story.
Bonus Tips:
- Utilize online coding playgrounds to experiment with JavaScript basics without setting up a full environment.
- Explore interactive tutorials and challenges to solidify your understanding through practical application.
- Join online communities and forums to connect with other JavaScript learners and seasoned developers.
- Most importantly, have fun! The joy of discovery and creation fuels the journey of mastering any language, including the magnificent JavaScript.
So, with these foundational elements in your toolbox and a thirst for knowledge fueling your spirit, embark on your JavaScript coding adventure! Remember, consistency is your compass, comments are your guideposts, and the community is your cheering squad. Now go forth and write your code, line by beautiful line!