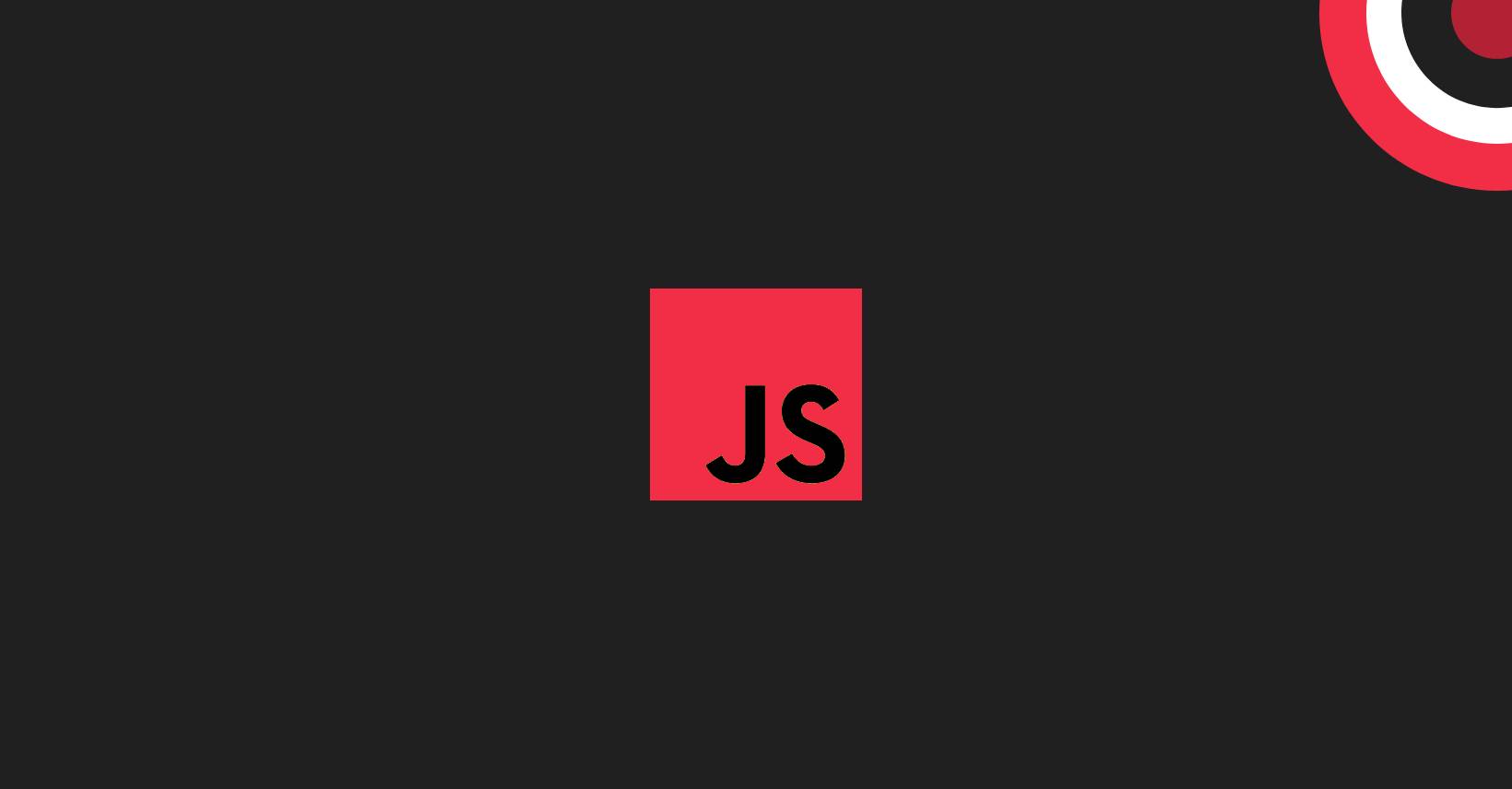
Introduction to JavaScript
Forget the traditional beginner's climb from simple concepts to complex ones. We'll dive headfirst into the wilderness, dissecting intricate JavaScript components to understand their inner workings.
As a newbie web developer, venturing online for tools and tutorials unveils a bewildering landscape. Frameworks like React, Vue, Angular, and Svelte dominate the scene, seemingly pushing aside the good old days of HTML, CSS, and sprinkles of JavaScript. Fear not, intrepid explorer! This article equips you with the map and compass to navigate this rapidly evolving JavaScript jungle.
Forget the traditional beginner’s climb from simple concepts to complex ones. We’ll dive headfirst into the wilderness, dissecting intricate JavaScript components to understand their inner workings. This deconstruction-reconstruction approach fosters deep learning and keeps you ahead of the curve.
Behold, a typical React component – your potential web-building ally. At first glance, it might seem like hieroglyphics. But remember, this isn’t pure JavaScript; it’s JSX, a React-specific extension. Should you ditch vanilla JavaScript and learn JSX? Not!
function ToDoList() {
const [tasks, setTasks] = useState([]); // useState hook to store tasks
const addTask = (task) => {
setTasks([...tasks, task]); // Add new task to the state
};
const removeTask = (index) => {
const newTasks = tasks.slice(0, index).concat(tasks.slice(index + 1));
setTasks(newTasks); // Remove task at specific index
};
return (
<div className="todo-list">
<h1>To-Do List</h1>
<input type="text" placeholder="Enter a new task" onKeyUp={(e) => addTask(e.target.value)} />
<ul>
{tasks.map((task, index) => (
<li key={index}>
{task}
<button onClick={() => removeTask(index)}>Remove</button>
</li>
))}
</ul>
</div>
);
}
export default ToDoList;
Breakdown of the Code:
- The component is called
ToDoList
. - It uses the
useState
hook to keep track of the list of tasks in an array namedtasks
. - The
addTask
function takes a new task as input and adds it to thetasks
array using the spread operator (…). - The
removeTask
function takes the index of the task to be removed and updates thetasks
array accordingly. - The component returns a JSX structure representing the to-do list UI:
- An
h1
element for the title. - An input field for adding new tasks.
- A
ul
element to display the existing tasks. - Each task is displayed as an
li
element with its text and a “Remove” button.
- An
Running the Code:
This is just a basic example, and you’ll need to set up a React project and environment to run it. However, it should give you a good understanding of how React components work and how they can be used to build interactive web applications.
Think of JSX as a fancy dress on the JavaScript core. Master the core first, and picking up JSX and other flavors becomes a breeze. This course meticulously breaks down this React component, demystifying objects, methods, functions, template literals, arrays, and more. By the end, you’ll not only comprehend this complex beast but also be able to tackle any JavaScript framework with confidence.
So, strap on your coding belt, sharpen your curiosity, and get ready to conquer the JavaScript jungle! Embrace the challenge, savor the deconstructions, and celebrate the joy of building your web-wonders.