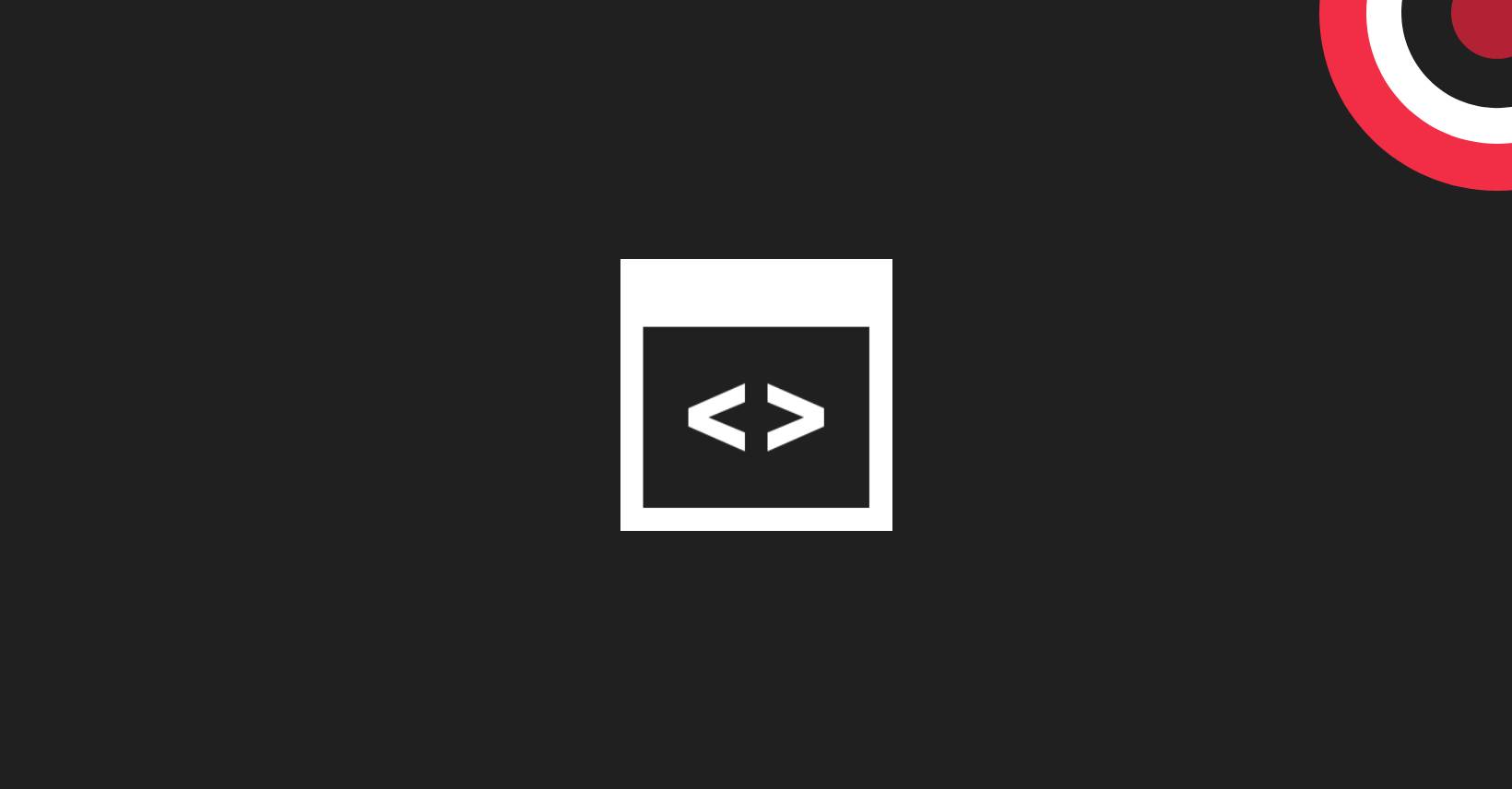
Expert insights on strip_tags and substr functions in PHP
Elevate your PHP coding with expert insights on strip_tags and substr functions. Explore real-world examples for effective and secure string manipulation.
Table of Contents
In the dynamic field of web development, PHP stands tall as a versatile scripting language, offering essential functions like strip_tags
and substr
. These functions, often underrated, play an important role in efficient string manipulation, offering a secure and flexible approach for developers.
The strip_tags
function is a key player in fortifying web applications against potential security threats. Its primary function involves the surgical removal of HTML and PHP tags from a given string, ensuring data integrity and thwarting malicious exploits.
$input = "<p>Projects <b>Engine</b>!</p>";
$cleanedText = strip_tags($input);
echo $cleanedText; // Output: Projects Engine!
$input = "<p>Projects <b>Engine</b>!</p>";
$allowedTags = "<b>";
$cleanedText = strip_tags($input, $allowedTags);
echo $cleanedText; // Output: Projects, <b>Engine</b>!
The substr
function proves indispensable for extracting substrings based on specified starting points and lengths, providing developers with fine-tuned control over text manipulation.
$text = "The quick brown fox jumps over the lazy dog";
$substring = substr($text, 10, 15);
echo $substring; // Output: brown fox jumps
$text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
$maxLength = 20;
$truncatedText = substr($text, 0, $maxLength) . '...';
echo $truncatedText; // Output: Lorem ipsum dolor...
Getting the hang of PHP‘s basic tricks like strip_tags
and substr
is like having some coding superpowers. These functions aren’t just about keeping your apps safe; they’re your trusty sidekicks for playing with data. Try out the examples we threw in here, and you’ll be slinging out robust, safe, and slick web solutions in no time.
How to use these functions in WordPress
This function is designed to provide a truncated version of a post title, ensuring it doesn’t exceed a specified character length. If the function already exists, it won’t be redefined, allowing for better compatibility in various contexts.
if ( ! function_exists( 'pe_get_title' ) ) {
/**
* Return post title with custom characters amount
*
* @param int $length post title length.
* @param null|int $post_id post_id.
*
* @returns string
*/
function pe_get_title( $length = 90, $post_id = null ) {
if ( ! $post_id ) {
$post_id = get_the_ID();
}
if ( ! $post_id ) {
return false;
}
$page_object = get_post( $post_id );
// phpcs:ignore
return substr( strip_tags( $page_object->post_title ), 0, $length ) . '...';
}
}