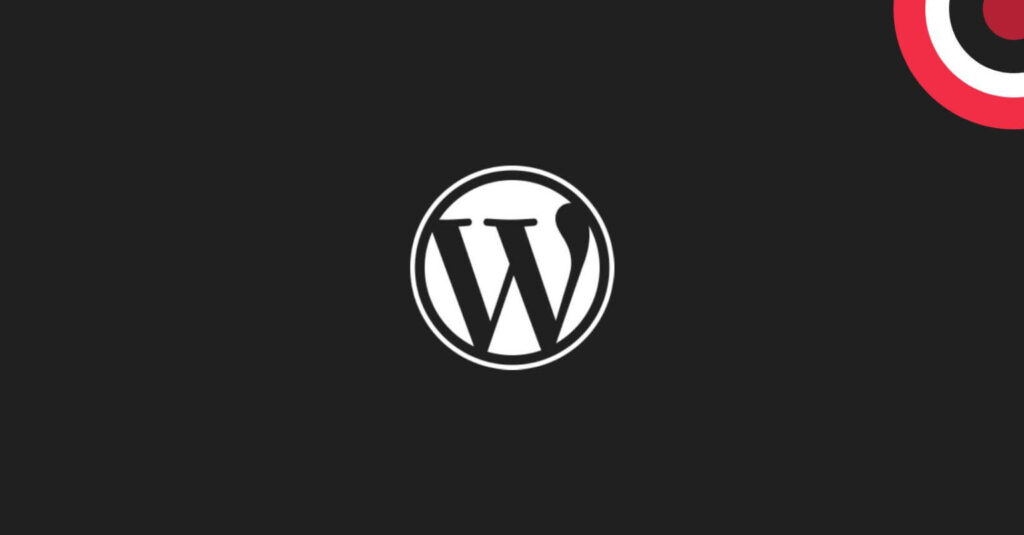
Implementing a Custom Media Upload Button with Media Uploader and JavaScript in WordPress
Implementation of a custom media upload button within a WordPress environment using Media Uploader and JavaScript.
Table of Contents
Customizing features in WordPress to improve user experience is a standard practice among developers. The following PHP code demonstrates the implementation of a custom media upload feature within a WordPress environment. Each line serves a specific purpose, contributing to the seamless integration of this functionality.
// Get WordPress' media upload URL
$upload_link = esc_url( get_upload_iframe_src( null, $post->ID ) );
// See if there's a media id already saved as post meta
$file_id = get_post_meta( $post->ID, '_file_id', true );
// Get the file src
$file_src = wp_get_attachment_image_src( $file_id, 'full' );
// For convenience, see if the array is valid
$file_exists = is_array( $file_src );
$upload_link = esc_url(get_upload_iframe_src(null, $post->ID));
Retrieves the media upload URL for WordPress, ensuring proper sanitization using esc_url
. The link is associated with the current post ID.
$file_id = get_post_meta($post->ID, '_file_id', true);
Fetches the file ID stored as post metadata, using the key ‘_file_id’ associated with the current post.
$file_src = wp_get_attachment_image_src($file_id, 'full');
Obtains the file source URL for the full-sized image based on the retrieved file ID, storing it in the variable $file_src
.
$file_exists = is_array($file_src);
Check whether the $file_src
variable contains a valid array, creating a boolean flag indicating the existence of a file.
HTML
Custom File Container
<!-- Your file container, which can be manipulated with js -->
<div class="custom-file-container">
<?php if ($file_exists): ?>
<img src="<?php echo $file_src[0]; ?>" alt="Projects Engine" />
<?php endif; ?>
</div>
Initiates a container element for the custom file, allowing manipulation with JavaScript. If a file exists, an image tag is generated with the file source.
File Upload and Delete Links
<!-- Your add & remove file links -->
<p class="hide-if-no-js">
<a class="custom-file-upload <?php echo ( $file_exists ) ? 'hidden' : ''; ?>" href="<?php echo $upload_link ?>">
<?php _e('Set file') ?>
</a>
<a class="custom-file-delete <?php echo ( ! $file_exists ) ? 'hidden' : ''; ?>" href="#">
<?php _e('Remove this file') ?>
</a>
</p>
Displays links for setting or removing a file. The visibility of each link is conditionally modified based on the existence of a file.
Hidden Input for file ID
<!-- A hidden input to set and post the chosen image id -->
<input class="custom-file-id" name="custom-file-id" type="hidden" value="<?php echo esc_attr( $file_id ); ?>" />
Creates a hidden input field to store and post the chosen file ID when the form is submitted.
This PHP code serves as a foundation for integrating a custom file upload feature into WordPress, allowing developers to customize and extend the functionality according to project requirements. Understanding each line’s purpose facilitates effective modification and adaptation for specific use cases.
JavaScript
The following JavaScript code showcases the implementation of a custom file upload functionality in a WordPress environment. This breakdown will help you understand each line’s role in facilitating the interaction between the user interface and the underlying functionality.
Variable Initialization
// Set all variables to be used in scope
let frame,
metaBox = document.getElementById('upload_plugin'), // Your meta box id here
addFileLink = metaBox.querySelector('.custom-file-upload'),
delFileLink = metaBox.querySelector('.custom-file-delete'),
fileContainer = metaBox.querySelector('.custom-file-container'),
fileIdInput = metaBox.querySelector('.custom-file-id');
Initializes variables for the media frame, meta box, add and delete file links, file container, and file ID input.
File Upload Link Event
// add file link
addFileLink.addEventListener('click', ( event ) => {
event.preventDefault();
// If the media frame already exists, reopen it.
if ( frame ) {
frame.open();
return;
}
// Create a new media frame
frame = wp.media({
title: 'Select and Upload Media',
button: {
text: 'Use this media'
},
multiple: false // Set to true to allow multiple files to be selected
});
// When a file is selected in the media frame...
frame.on( 'select', function() {
// Get media attachment details from the frame state
let attachment = frame.state().get('selection').first().toJSON();
// Send the attachment URL to our custom image input field.
// or a file with a custom thumbnail.
fileContainer.innerHTML = '<img src="'+attachment.url+'" alt="Projects Engine" />';
// Send the attachment id to our hidden input
fileIdInput.value = attachment.id;
// Hide the add image link
addFileLink.classList.add( 'hidden' );
// Unhide the remove image link
delFileLink.classList.remove( 'hidden' );
});
// Finally, open the modal on click
frame.open();
});
Listens for a click event on the “Add File” link. Check if the media frame already exists; if it does, reopen it; otherwise, create a new media frame. When a file is selected, retrieves attachment details, updates the file container with a thumbnail, sets the file ID in the hidden input, and toggles the visibility of the add and remove file links.
File Delete Link Event
// delete file
delFileLink.addEventListener('click', ( event ) => {
event.preventDefault();
// Clear out the preview image
fileContainer.innerHTML = '';
// Un-hide the add image link
addFileLink.classList.remove( 'hidden' );
// Hide the delete image link
delFileLink.classList.add( 'hidden' );
// Delete the image id from the hidden input
fileIdInput.value = '';
});
Listens for a click event on the “Delete File” link. Clears the preview image, shows the add file link, hides the delete file link, and resets the hidden input’s value.
This JavaScript code complements the PHP implementation, providing an interactive user interface for handling file uploads and deletions in a WordPress environment. Understanding each line helps developers customize and extend this functionality to suit their specific project requirements.
The combined PHP and JavaScript code results in a seamlessly integrated custom file upload feature within a WordPress environment. Users can effortlessly interact with the user interface, allowing them to select and upload a media file. Upon selecting a file, the preview is dynamically updated in real time, providing a visual representation of the chosen media.
The interface also offers the option to remove the uploaded file, reset the display, and allow users to choose a different file if needed. The entire process is smooth and user-friendly, enhancing the overall content management experience for WordPress users. This solution not only adds a practical and aesthetically pleasing feature but also demonstrates the synergy between server-side and client-side scripting in crafting interactive web applications.
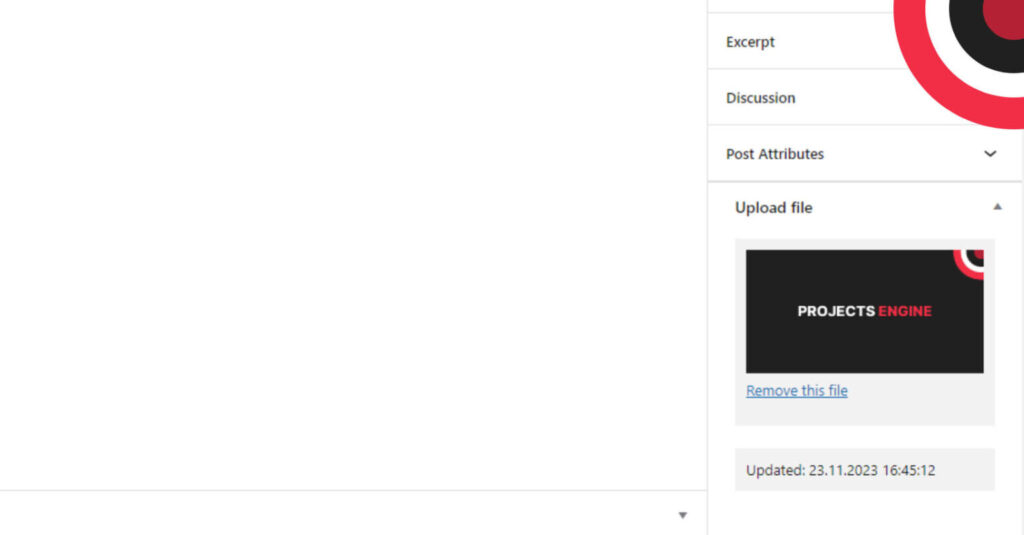
The presented PHP and JavaScript code is designed to operate within a meta box added to the WordPress admin area, specifically on the post-editing screen. This integration allows users to manage and associate media files with their posts seamlessly.
However, for those seeking to implement this custom file upload feature on the front end of their WordPress site, an essential step is to include the wp_enqueue_media()
function. This function ensures that the WordPress media library scripts and styles are properly enqueued, providing the necessary infrastructure for media handling.
function pe_enqueue_scripts() {
wp_enqueue_media();
}
add_action( 'wp_enqueue_scripts', 'pe_enqueue_scripts' );
By including wp_enqueue_media()
in the front end, users can extend this interactive file upload functionality beyond the confines of the admin area, offering a consistent and user-friendly experience for managing media across different parts of their WordPress website.
This ensures that the code operates smoothly in both the backend and frontend environments, catering to diverse user needs and scenarios.