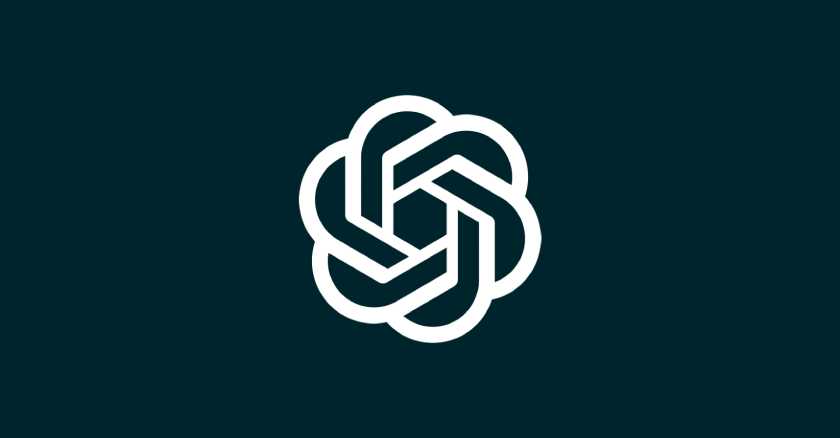
How to use the ChatGPT API and integrate a ChatGPT prompt with HTML and JavaScript
In this tutorial, we'll walk through the process of using the ChatGPT API and integrating a ChatGPT prompt into a web page using HTML and JavaScript.
ChatGPT, developed by OpenAI, is a powerful language model capable of generating human-like text based on the input it receives. Integrating ChatGPT into web applications allows for interactive and engaging user experiences. In this tutorial, we’ll walk through the process of using the ChatGPT API and integrating a ChatGPT prompt into a web page using HTML and JavaScript.
Prerequisites
Before we begin, ensure you have the following:
- An OpenAI API key. You can sign up for access on the OpenAI website.
- Basic knowledge of HTML, JavaScript, and web development concepts.
Setting Up the HTML Structure
We’ll start by creating the HTML structure for our web page. This will include elements for user input, displaying chat history, and a button to send messages.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Stream ChatGPT Response</title>
</head>
<body>
<h1>Stream ChatGPT Response</h1>
<textarea id="inputText" rows="10" cols="50"></textarea>
<button id="startButton" style="display: block;">Start Connection</button>
<h2>Response:</h2>
<textarea id="output" rows="30" cols="100" readonly></textarea>
</body>
<html>
Interacting with the ChatGPT API
Next, we’ll add JavaScript code to handle interactions with the ChatGPT API. This code will initiate the connection, send user messages to ChatGPT, and display the responses.
<script>
const inputText = document.getElementById('inputText');
const outputDiv = document.getElementById('output');
const startButton = document.getElementById('startButton');
const MODEL = 'gpt-4';
let currentInput = 'Write me something about React.';
let isStreaming = false;
inputText.addEventListener('input', async function() {
if (!isStreaming) {
currentInput = inputText.value;
}
});
startButton.addEventListener('click', async function() {
if (!isStreaming) {
await startStreaming();
}
});
async function startStreaming() {
try {
isStreaming = true;
outputDiv.innerText = '';
const data = {
'model': MODEL,
'messages': [
{ 'role': 'user', 'content': currentInput },
{ 'role': 'system', 'content': '' }
],
'stream': true
};
const response = await fetch('https://api.openai.com/v1/chat/completions', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_OPENAI_API_KEY'
},
body: JSON.stringify(data)
});
const reader = response.body.getReader();
let partialData = '';
while (isStreaming) {
const { done, value } = await reader.read();
if (done) break;
const chunk = new TextDecoder().decode(value);
const data = partialData + chunk;
const chunks = data.split('data: ');
for (let i = 1; i < chunks.length; i++) {
const jsonData = chunks[i];
try {
const parsedData = JSON.parse(jsonData);
if (parsedData.choices && parsedData.choices[0] && parsedData.choices[0].delta && parsedData.choices[0].delta.content) {
outputDiv.innerText += parsedData.choices[0].delta.content;
}
} catch (error) {
console.error('Error parsing JSON:', error);
}
}
partialData = chunks[chunks.length - 1];
}
} catch (error) {
console.error('Error streaming response:', error);
isStreaming = false;
} finally {
isStreaming = false;
}
}
</script>
This JavaScript code sets up a real-time streaming connection with the OpenAI ChatGPT API and handles the interaction between the user input and the API response. Let’s break down each part of the code:
- Variable Declarations:
inputText
: Retrieves the textarea element with the ID “inputText” from the HTML document.outputDiv
: Retrieves the div element with the ID “output” from the HTML document.startButton
: Retrieves the button element with the ID “startButton” from the HTML document.MODEL
: Defines the model to be used for generating responses, set to ‘gpt-4’.currentInput
: Initializes a variable to store the current user input.isStreaming
: Initializes a boolean variable to track whether the streaming connection is active or not.
- Event Listeners:
inputText.addEventListener('input', async function() {...})
: Listens for input events on the inputText textarea. When the user types or modifies the text, it updates thecurrentInput
variable with the latest input.startButton.addEventListener('click', async function() {...})
: Listens for click events on the startButton. When clicked, it initiates the streaming connection by calling thestartStreaming
function.
- startStreaming Function:
- Initiates the streaming connection with the ChatGPT API.
- Clears the outputDiv content.
- Constructs the data payload to be sent to the API, including the model, current user input, and streaming flag.
- Sends a POST request to the ChatGPT API endpoint with the constructed data payload.
- Sets up a reader to read the response stream.
- Asynchronously processes chunks of data received from the stream:
- Decodes the data from binary to text.
- Splits the data into chunks based on the ‘data: ‘ delimiter.
- Parses each chunk as JSON data.
- Appends the content of the response from ChatGPT to the outputDiv.
- Handles any errors that occur during the streaming process.
Conclusion
In this tutorial, we’ve learned how to create a real-time streaming chat-like interface using the ChatGPT API, HTML, and JavaScript. By initiating a streaming connection with the ChatGPT API, we can provide users with immediate responses as they type. Experiment with different models and customize the user interface to suit your application’s needs. Happy coding!