How to use Sass in WordPress
Sass for WordPress is essential if you want your code to be simpler to read and substantially easier to understand.
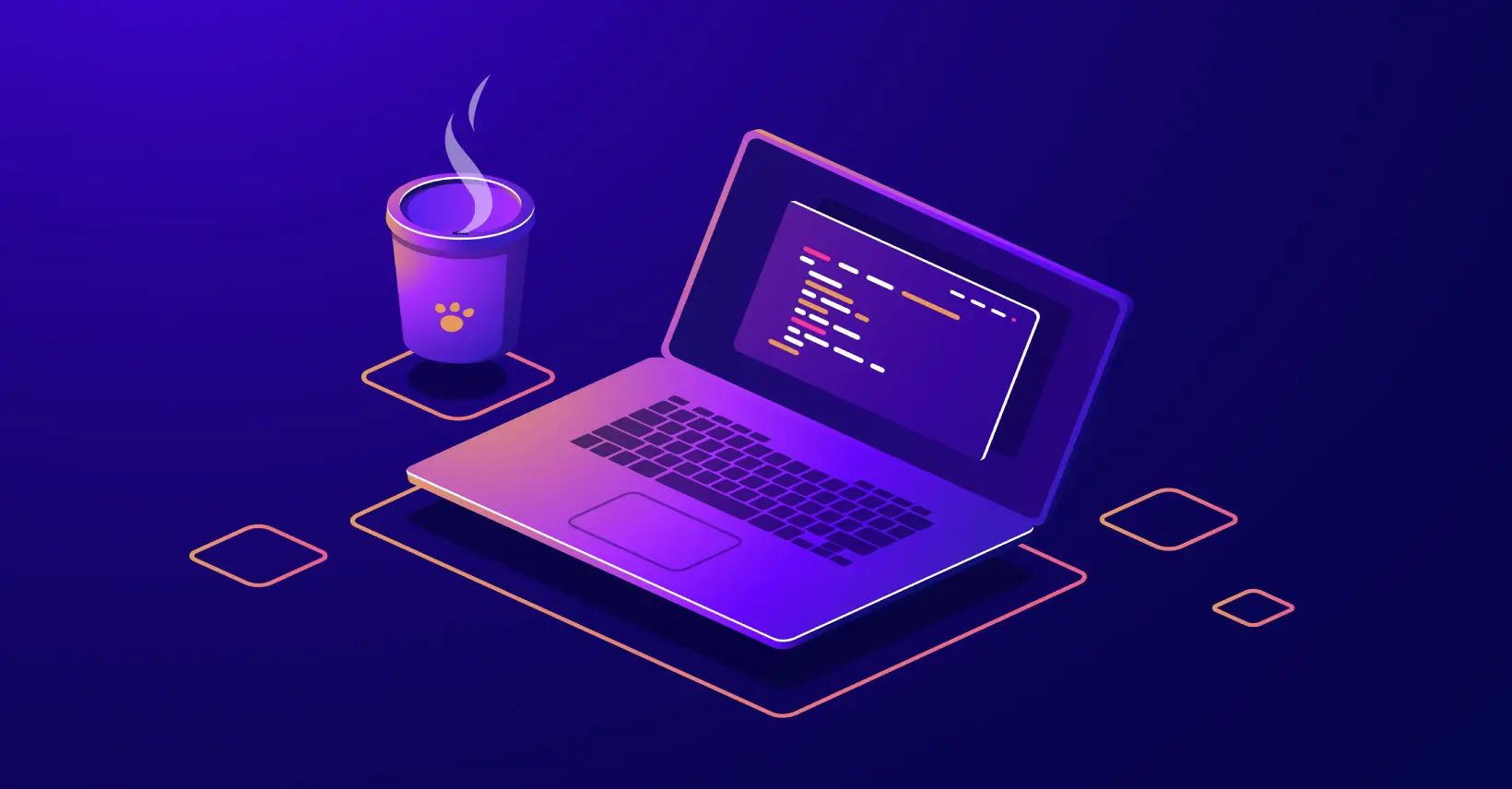
To use Sass in WordPress, you can follow these steps:
- Install a Sass compiler: First, you need to install a Sass compiler on your computer. There are various options available, including Ruby Sass, Node Sass, and Dart Sass. You can choose any of them depending on your preference.
- Set up your Sass folder structure: Create a new folder in your WordPress theme directory, named “
sass
“. This folder will contain your Sass files, organized in a way that makes sense to you. For example, you can create separate folders for variables, mixins, and partials. - Create a
style.scss
file: In the “sass” folder, create a file named “style.scss
“. This file will be the entry point for your Sass styles. In this file, you can import all the other Sass files you have created, using the@import
rule. - Compile your Sass code: Once you have written your Sass code, you need to compile it into CSS so that it can be used by WordPress. You can compile your Sass code using a command line tool or a task runner like Grunt or Gulp. Alternatively, you can use a WordPress plugin like WP-SCSS, which will compile your Sass code automatically every time you save changes.
- Enqueue the compiled CSS in WordPress: Finally, you need to enqueue the compiled CSS in WordPress. You can do this by adding a function to your
functions.php
file that calls thewp_enqueue_style
function and points to the compiled CSS file.
By following these steps, you can use Sass in your WordPress theme and take advantage of its features such as variables, mixins, and nested syntax to write more efficient and maintainable CSS code.
What improvements does SASS make over CSS?
Sass for WordPress is essential if you want your code to be simpler to read and substantially easier to understand. To simplify your work, Sass adds the following functionalities to CSS:
- Variables
- Nested rules
- Partials
- Modules
- Mixins
- Extends/inheritance
- Operators
Modules
So when you break up your Sass into distinct portions with the @use rule, it will start to load the Sass file as a module. In other words, you may use the namespace, which is based on the name of your file, to refer to its variables, functions, and mixins in your Sass files.
// _base.scss
$font-stack: 'Poppins', sans-serif;
$primary-color: #000;
body {
font: 100% $font-stack;
color: $primary-color;
}
// styles.scss
@use 'base';
.wrapper {
background-color: base.$primary-color;
color: #fff;
}
CSS
body {
font: 100% 'Poppins', sans-serif;
color: #000;
}
.wrapper {
background-color: #000;
color: #fff;
}
Mixins
CSS has a lot of features that can make writing code a bit tiresome. By establishing a collection of CSS declarations that you can subsequently reuse across your coding, a mixin
helps you prevent it. To further increase the flexibility of your mixin
, it is also possible to pass in specific values. The vendor prefixes are one instance when mixin
use is suitable. Take transform
as an example:
Sass
@mixin transform($deg) {
-webkit-transform: $deg;
-ms-transform: $deg;
transform: $deg;
}
.box { @include transform(rotate(40deg)); }
CSS
.box {
-webkit-transform: rotate(40deg);
-ms-transform: rotate(40deg);
transform: rotate(40deg);
}
Use the @mixin
directive to create a mixin
, and then give it a name.
Operators
This Sass feature includes several handy math operators, including +, -, *, /, and %, because it may be quite beneficial to perform calculations in your CSS on occasion. You may find some basic arithmetic calculations to determine the width for aside
and article
in the example below:
SCSS
.container {
width: 100%;
}
article[role="wrapper"] {
float: left;
width: 600px / 900px * 100%;
}
aside[role="complementary"] {
float: right;
width: 300px / 900px * 100%;
}
OUTPUT
.container {
width: 100%;
}
article[role=wrapper] {
float: left;
width: 66.6666666667%;
}
aside[role=complementary] {
float: right;
width: 33.3333333333%;
}
In conclusion, CSS contains all the tools you need to build amazing websites, but using CSS preprocessors like Sass will make the process simpler and less time-consuming. By allowing developers to employ a variety of helpful features including variables, nested rules, mixins, modules, partials, extends/inheritance, and operators, Sass for WordPress greatly increases the CSS’s capability. Finally, it will compile your code and output it as CSS so that the browser can read it.
Was this post helpful? ( Answers: 0 )
Leave a comment
If you enjoyed this post or have any questions, please leave a comment below. Your feedback is valuable!