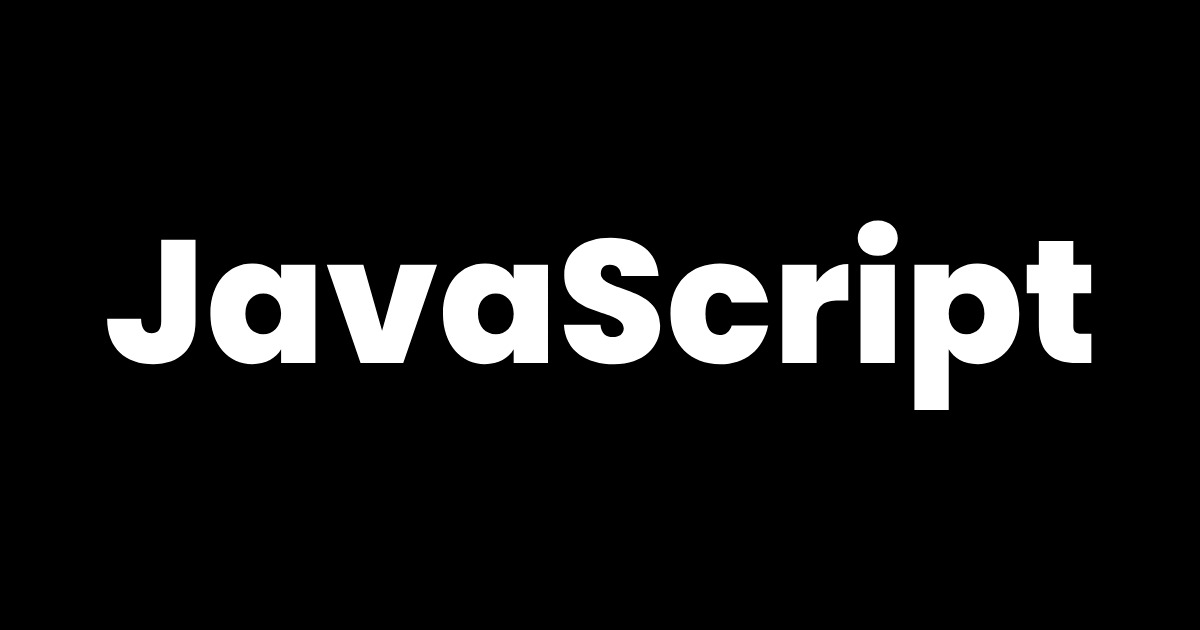
How to use object destructuring in JavaScript?
Object destructuring is a feature in JavaScript that allows you to extract properties from an object and assign them to variables in a concise and convenient way.
When working with JavaScript, it is common to encounter situations where you need to extract specific items from an object. JavaScript provides various techniques to accomplish this task, including object destructuring and non-destructuring approaches. In this article, we will explore both methods and discuss their advantages and use cases.
What is object destructuring in JavaScript?
Object destructuring is a feature in JavaScript that allows you to extract properties from an object and assign them to variables in a concise and convenient way. It provides a shorter syntax for accessing and working with object properties, making code more readable and expressive.
With object destructuring, you can create variables that directly correspond to the properties of an object, eliminating the need for repetitive object dot notation. This technique simplifies the process of extracting values from objects and allows for more flexible code manipulation.
To use object destructuring, you enclose the desired property names in curly braces {}
on the left side of an assignment expression. The property names within the curly braces should match the properties of the object you want to destructure. The values of those properties will be assigned to the corresponding variables.
When to use destructuring in JavaScript?
Destructuring in JavaScript is useful in various scenarios where you want to extract specific properties from an object or elements from an array. Here are some situations where object destructuring can be particularly beneficial:
Extracting Object Properties
When you need to access and work with individual properties of an object, destructuring simplifies the code by allowing you to assign property values to variables directly. It reduces the need for repetitive dot notation when accessing object properties.
Function Parameters
Destructuring is handy when defining function parameters. Instead of passing an entire object and accessing its properties within the function body, you can destructure the properties directly in the function parameter list. This approach makes the function signature more expressive and self-contained.
Multiple Return Values
When a function returns an object with multiple properties, destructuring enables you to extract specific values easily. By assigning the returned object to variables with the corresponding property names, you can access those values directly, making the code more readable and concise.
Renaming Variables
Object destructuring allows you to assign extracted properties to variables with different names. This feature is useful when you want to rename variables for clarity or avoid naming conflicts. By specifying new variable names during the destructuring assignment, you can achieve more expressive and descriptive code.
Importing from Modules
When importing modules in JavaScript, destructuring simplifies the process of importing specific functions or values from a module. Instead of importing the entire module and referencing its members through dot notation, you can destructure the desired functions or values during the import statement, enhancing code readability and reducing the amount of imported code.
It’s important to note that while object destructuring provides convenience and readability, it may not always be the most appropriate choice. For simpler cases or when working with a small number of properties, using object dot notation might be more straightforward and sufficient. Choose destructuring when it enhances code readability, reduces repetition, and simplifies complex property extraction scenarios.
How to use object destructuring in JS?
Object destructuring is a convenient feature introduced in ECMAScript 6 (ES6) that allows you to extract properties from an object and assign them to variables with the same name. Let’s consider an example object called person
:
const person = {
name: 'John Doe',
age: 30,
profession: 'Developer',
};
To extract specific properties from the person
object using destructuring, you can simply enclose the desired property names in curly braces and assign them to variables:
const { name, age } = person;
console.log(name); // Output: John Doe
console.log(age); // Output: 30
Destructuring enables you to access object properties directly without explicitly referencing the object. It provides a concise and readable way to extract multiple items from an object in a single line of code.
How to get the object items without destructuring?
While object destructuring is a powerful tool, there may be situations where it is unnecessary or impractical to use. In such cases, you can rely on traditional methods to access object items individually. Here’s an example of extracting properties from the person
object without using destructuring:
const name = person.name;
const age = person.age;
console.log(name); // Output: John Doe
console.log(age); // Output: 30
This approach explicitly assigns the desired properties to separate variables using dot notation. Although it requires more lines of code compared to destructuring, it provides a straightforward and accessible way to retrieve object items, especially when dealing with a small number of properties.
Use Cases and Considerations
Both object destructuring and non-destructuring approaches have their own strengths and use cases. Here are a few scenarios to help you decide which technique is more suitable for your needs:
- Object destructuring is particularly useful when you want to extract multiple properties from an object and assign them to variables in a concise and readable manner. It simplifies the code and improves code maintainability.
- Non-destructuring is beneficial when you only need to extract a few properties from an object or when you prefer a more explicit and traditional coding style. It may be preferred in situations where object deconstruction introduces unnecessary complexity.
Additionally, it’s worth noting that object destructuring can handle nested objects and provide default values for properties. This flexibility makes it a powerful tool for extracting data from complex structures.
In conclusion, both object destructuring and non-destructuring methods offer viable solutions for retrieving object items in JavaScript. Object destructuring provides a concise and elegant approach, while non-destructuring offers a more traditional and explicit way to access object properties. The choice between the two techniques ultimately depends on your coding style, readability requirements, and the complexity of the object structure you are working with.
How to use array destructuring in JavaScript?
Here’s an example that demonstrates how to use object destructuring and non-destructuring techniques to extract items from an array of objects:
// Array of objects
const users = [
{ id: 1, name: 'John Doe', age: 25 },
{ id: 2, name: 'Jane Smith', age: 30 },
{ id: 3, name: 'Bob Johnson', age: 40 },
];
// Using object destructuring
const [{ name: user1Name, age: user1Age }, { name: user2Name, age: user2Age }] = users;
console.log(user1Name); // Output: John Doe
console.log(user1Age); // Output: 25
console.log(user2Name); // Output: Jane Smith
console.log(user2Age); // Output: 30
// Without object destructuring
const user3 = users[2];
console.log(user3.name); // Output: Bob Johnson
console.log(user3.age); // Output: 40
In the example above, we have an array called users
that contains multiple objects, each representing a user.
Using object destructuring, we extract the name
and age
properties from the first two objects in the array and assign them to variables user1Name
, user1Age
, user2Name
, and user2Age
. This allows us to access these properties directly without explicitly referencing the objects.
On the other hand, without object destructuring, we retrieve the third object from the users
array using array indexing (users[2]
). We can then access the name
and age
properties of that object using dot notation (user3.name
, user3.age
).
Both approaches provide a way to extract object items from an array, but object destructuring offers a more concise and readable syntax when you need to extract multiple properties at once. However, if you only need to access a single object or a small number of properties, the non-destructuring method can be a simpler alternative.
If you want to loop through the array of objects and extract the items using object destructuring or non-destructuring, you can use a loop such as for...of
or forEach()
. Here’s an example:
// Array of objects
const users = [
{ id: 1, name: 'John Doe', age: 25 },
{ id: 2, name: 'Jane Smith', age: 30 },
{ id: 3, name: 'Bob Johnson', age: 40 },
];
// Using object destructuring with a for...of loop
for (const { name, age } of users) {
console.log(name); // Output: John Doe, Jane Smith, Bob Johnson
console.log(age); // Output: 25, 30, 40
}
// Without object destructuring using forEach()
users.forEach(user => {
console.log(user.name); // Output: John Doe, Jane Smith, Bob Johnson
console.log(user.age); // Output: 25, 30, 40
});
In the example above, we iterate over the users
array using a for...of
loop. Within each iteration, we destructure the name
and age
properties of each object and directly log them to the console.
Alternatively, we can use the forEach()
method on the users
array, passing a callback function that takes each user object as an argument. Within the callback function, we access the name
and age
properties of each object using dot notation and log them to the console.
Both approaches allow you to loop through the array of objects and extract the desired items using either object destructuring or non-destructuring techniques. Choose the method that suits your preference and coding style.