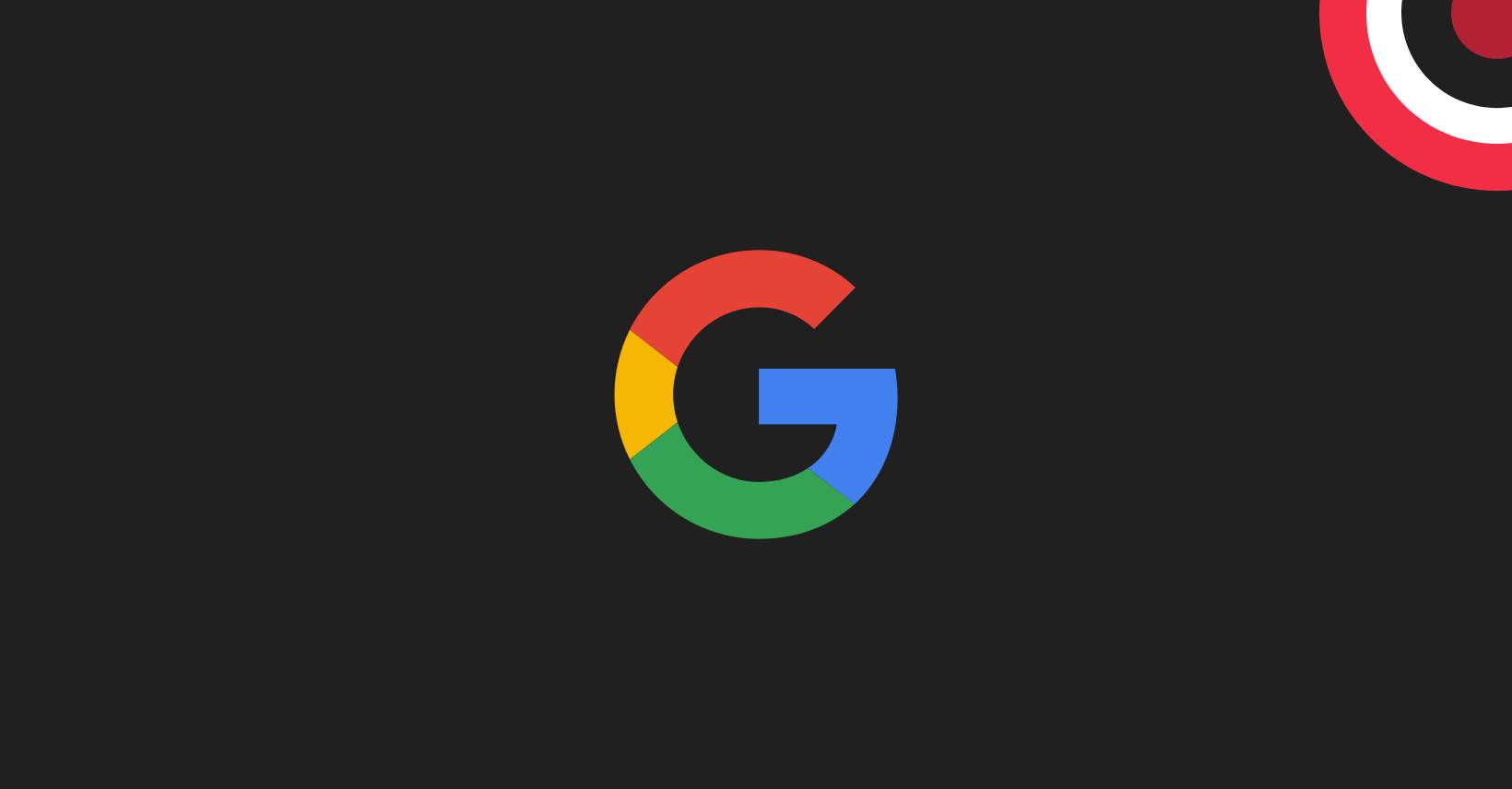
How to use OAuth 2.0 to access Google APIs and how to obtain access and refresh tokens in PHP
This guide will walk you through the process of using OAuth 2.0 to obtain access and refresh tokens, ensuring secure and efficient integration with Google services.
This guide will walk you through the process of using OAuth 2.0 to obtain access and refresh tokens, ensuring secure and efficient integration with Google services.
Understanding OAuth 2.0
OAuth 2.0 (Open Authorization 2.0) is an authorization framework that enables third-party applications to obtain limited access to a user’s account on a web service, without exposing the user’s credentials to the application. It is widely used for delegated access, allowing applications to access resources on behalf of a user after the user grants permission.
Setting up the Google API Project and obtaining OAuth 2.0 credentials from the Google API Console
To begin, create a project in the Google Cloud Console. Enable the desired APIs and set up the OAuth 2.0 credentials, obtaining a client ID and client secret.
Authentication Process
You will need a method named auth
that generates a URL for initiating the OAuth 2.0 authentication process with Google.
public function auth( $state ) {
$auth = 'https://accounts.google.com/o/oauth2/auth';
$scopes = 'https://www.googleapis.com/auth/userinfo.email https://www.googleapis.com/auth/userinfo.profile';
$client_id = 'your_client_id';
$client_secret = 'your_client_secret';
$redirect_uri = 'https://projectsengine.com/verify';
$response_type = 'code';
$access_type = 'offline';
return $auth . '?' . http_build_query([
'client_id' => $client_id,
'response_type' => 'code',
'state' => $state,
'scope' => $scopes,
'access_type' => $access_type,
'redirect_uri' => $redirect_uri,
]);
}
Authentication URL and Scopes:
- The base URL for Google OAuth 2.0 authentication is stored in the
$auth
variable. - The
$scopes
variable contains a space-separated list of requested access scopes. In this case, it requests access to the user’s email and profile information.
Client Credentials:
- The client ID (
$client_id
) and client secret ($client_secret
) are provided. These are unique identifiers assigned by Google when registering the application in the Google Cloud Console. - The
$redirect_uri
variable holds the URI where the user will be redirected after granting or denying permission. In this case, it is set to ‘https://site.com/your-url‘.
Authentication Parameters:
- The
$response_type
variable is set to ‘code’, indicating that the authorization server should return an authorization code. - The
$access_type
is set to ‘offline’, indicating that the application needs to access the user’s data when they are not present.
Building the Authorization URL:
- The method returns the constructed URL by appending the authentication parameters as query parameters using
http_build_query
.
Returned URL Format:
- The returned URL looks like this:
https://accounts.google.com/o/oauth2/auth?client_id=<client_id>&response_type=code&state=<state>&scope=<scopes>&access_type=offline&redirect_uri=<redirect_uri>
Usage:
- This URL can redirect users to Google’s authorization endpoint to grant the necessary permissions to the application.
Google OAuth 2.0 Token Management in PHP
This next code defines two methods, refresh_token
and access_token
, for managing OAuth 2.0 tokens in the context of Google authentication. The code facilitates the retrieval of refresh and access tokens using a code and the retrieval of access token using a previously obtained refresh token.
/**
* Get Google refresh and access token.
*
* @param string $code
*
* @return json
*/
public function refresh_token( $code ) {
$auth = 'https://accounts.google.com/o/oauth2/token';
$grant_type = 'authorization_code';
$client_id = '';
$client_secret = '';
$redirect_uri = 'https://site.com/your-url';
$parameters = http_build_query([
'client_id' => $client_id,
'client_secret' => $client_secret,
'code' => $code,
'grant_type' => 'authorization_code',
'redirect_uri' => $redirect_uri,
]);
// Set up cURL
$ch = curl_init();
// Set the URL and parameters for the access token request
curl_setopt($ch, CURLOPT_URL, $auth );
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $parameters );
// Set the headers for the access token request
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Accept: application/json',
'Content-Type: application/x-www-form-urlencoded',
));
// Get the response from the access token request
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Refresh and access token
$response = curl_exec($ch);
$info = curl_getinfo($ch);
if( 200 === $info['http_code'] ):
$response = json_decode( $response );
// save the refresh and access token.
$refresh_token = $response->id_token;
$access_token = $response->access_token;
$expires_in = $response->expires_in;
return true;
endif;
return false;
}
- This method is responsible for exchanging an authorization code for refresh and access tokens.
- It sets up the necessary parameters, including the client ID, client secret, authorization code, and redirect URI.
- A cURL request is initiated to the Google OAuth 2.0 token endpoint with these parameters.
/**
* Get access token using refresh token.
*
* @return void
*/
public function access_token( $refresh_token ) {
// The token retrieved in the previous step.
$refresh_token = $refresh_token ?: '';
if( '' === $refresh_token ) {
return false;
}
$auth = 'https://oauth2.googleapis.com/token';
$grant_type = 'refresh_token';
$client_id = '';
$client_secret = '';
$parameters = http_build_query([
'client_id' => $client_id,
'client_secret' => $client_secret,
'grant_type' => $grant_type,
'refresh_token' => $refresh_token
]);
// Set up cURL
$ch = curl_init();
// Set the URL and parameters for the access token request
curl_setopt($ch, CURLOPT_URL, $auth );
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $parameters );
// Set the headers for the access token request
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Accept: application/json',
'Content-Type: application/x-www-form-urlencoded',
));
// Get the response from the access token request
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
$info = curl_getinfo($ch); // refresh and access token
if( 200 === $info['http_code'] ):
$response = json_decode( $response );
// save the refresh and access token.
$refresh_token = $response->id_token;
$access_token = $response->access_token;
$expires_in = $response->expires_in;
return true;
endif;
return false;
}
- This method retrieves a new access token using a previously obtained refresh token.
- Similar to the
refresh_token
function, a cURL request is made to the Google token endpoint with the client ID, client secret, grant type (refresh_token), and the refresh token. - If the request is successful, the new refresh and access tokens, along with their expiration time will be returned.
Google OAuth 2.0 User Information Retrieval in PHP
This PHP code defines a function named get_user
designed to retrieve user information from the Google OAuth 2.0 userinfo endpoint. The code uses OAuth tokens previously obtained during the authentication process.
/**
* Get user
* @api https://www.googleapis.com/oauth2/v2/userinfo
*
* @return booelan
* @since 1.0.0
*/
public function get_user() {
$refresh_token = '';
$access_token = '';
$expires_in = '';
$auth = 'https://www.googleapis.com/oauth2/v2/userinfo';
if( '' === $access_token ) {
return false;
}
// Set up cURL
$ch = curl_init();
// Set the URL and parameters for the access token request
curl_setopt($ch, CURLOPT_URL, $auth );
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'GET');
// Set the headers for the access token request
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Accept: application/json',
'Content-Type: application/x-www-form-urlencoded',
'Authorization: Bearer ' . $access_token,
));
// Get the response from the access token request
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
$info = curl_getinfo($ch); // refresh and access token
if( 200 === $info['http_code'] ):
$user = json_decode( $response );
$email = $user->email;
$first_name = $user->given_name;
$last_name = $user->family_name;
$picture = $user->picture;
return $user;
endif;
return false;
}
- The primary purpose of the
get_user
method is to fetch user information from Google’suserinfo
endpoint. - The function sets up a cURL (Client URL) request to communicate with the Google
userinfo
endpoint. - It uses the retrieved access token in the request headers to authenticate the API call.
By understanding the authentication flow, retrieving access and refresh tokens, and exploring real-world examples, you can seamlessly integrate Google services into your applications while ensuring data security and user privacy.