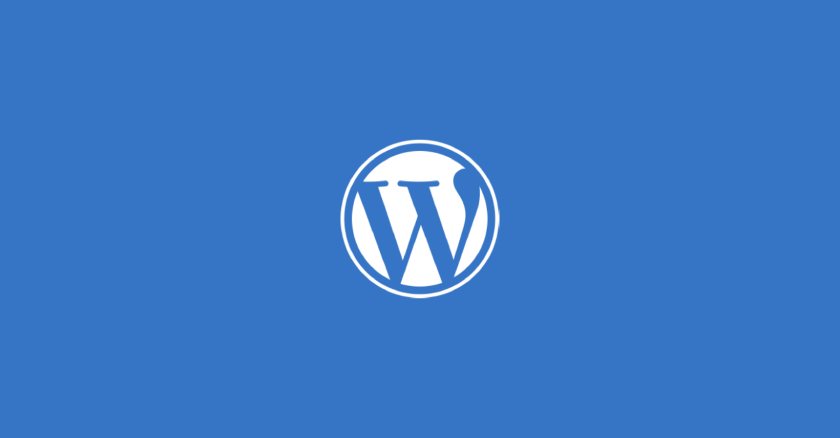
How to update usernames in WordPress via code and no plugin
WordPress, by default, doesn't allow users to change their usernames.
WordPress, by default, doesn’t allow users to change their usernames. The platform disables this feature to maintain database integrity and prevent potential security risks. While users can update their email addresses, changing usernames requires a different method.
Check if the username is available
Before proceeding with username updates, it’s crucial to check for username availability using this custom function. This step prevents conflicts and ensures a smooth transition.
/**
* Get user by field and value.
*
* @param $field
* @param $value
*
* @return array|object|null
*/
function zing_get_user( $field, $value ) {
global $wpdb;
return $wpdb->get_results(
$wpdb->prepare( "SELECT * FROM {$wpdb->prefix}users WHERE $field=%s", $value )
);
}
This function, zing_get_user
, is designed to retrieve user information from the WordPress database based on a specific field and its corresponding value.
You can use it to retrieve a user with a specified username. It can be used to verify that the current username entered by the user does not exist in the database. Once that’s cleared you can proceed to the next step.
/**
* Update the username.
*
* @param string $username
* @param string $userID
* @return array
*/
function zing_update_username( $username, $userID ) {
global $wpdb;
$table = $wpdb->prefix.'users';
return $wpdb->update(
$table, // $table string Required
array(
'user_login' => $username
), // $data array Required
array( 'ID' => $userID ), // $where array Required
array( '%s' ), // $format
array( '%s' ) // $where_format
);
}
Once we are sure that the username does not exist we can use this function to update the field. The user will be automatically logged out once the username is changed.
If the username is not available
$user = zing_get_user( 'user_login', $username );
if( $user && $user[0]->ID != get_current_user_id() ) {
return array(
'response' => false,
'code' => 'username_exists',
);
}
This code snippet uses the zing_get_user
function to check if a user with a specific username already exists in the WordPress database. Here’s a breakdown of how it works:
- First, it calls the
zing_get_user
function with two arguments:'user_login'
: This specifies that we want to search for users based on their username.$username
: This variable holds the username we want to check for.
- The result of the
zing_get_user
function is stored in the$user
variable. - The
if
statement checks two conditions:$user
: This checks if the$user
variable is not empty, meaning a user with the specified username was found in the database.$user[0]->ID != get_current_user_id()
: This checks if the ID of the first user in the$user
array (if any) is not the same as the ID of the currently logged-in user. If it’s not the same, it means the username already exists for a different user.
- If both conditions are met, the function returns an array with two elements:
'response' => false
: This indicates that the response is unsuccessful.'code' => 'username_exists'
: This provides a specific code (‘username_exists’) to identify the reason for the unsuccessful response.
Overall, this code snippet allows you to check if a username already exists in the WordPress database and provides a response indicating whether it’s available or not.
Updating the other fields
$args = array(
'ID' => get_current_user_id(),
'first_name' => $first_name,
'last_name' => $last_name,
'display_name' => "$first_name $last_name",
'user_login' => $username,
'user_nicename' => $username,
'user_email' => $email,
'description' => $description,
'user_url' => $website
);
// $userID = wp_insert_user( $args );
// $userID = wp_update_user( $args );
- The function
wp_insert_user()
is called with the$args
array as its argument. This function inserts or updates a user in the WordPress database, depending on whether an existing user ID is provided in the'ID'
parameter. - When creating a new user, if the
user_login
field is not provided, WordPress automatically generates auser_login
based on the user’s email address. This ensures that each user has a unique login identifier associated with their account. - When using
wp_update_user()
, theuser_login
field is required. However, you need to use the one created during the initial user registration. - For updating the user, you can use the functions mentioned earlier.
- Both functions essentially achieve the same result of updating a user’s profile information in the WordPress database.
- However,
wp_insert_user()
is typically used when creating a new user, whilewp_update_user()
is used for updating an existing user’s information. Depending on your specific use case, you may choose one function over the other.
Happy coding!