How to set up cookies using JavaScript
Cookies are like little notes that websites leave on your device when you visit them.
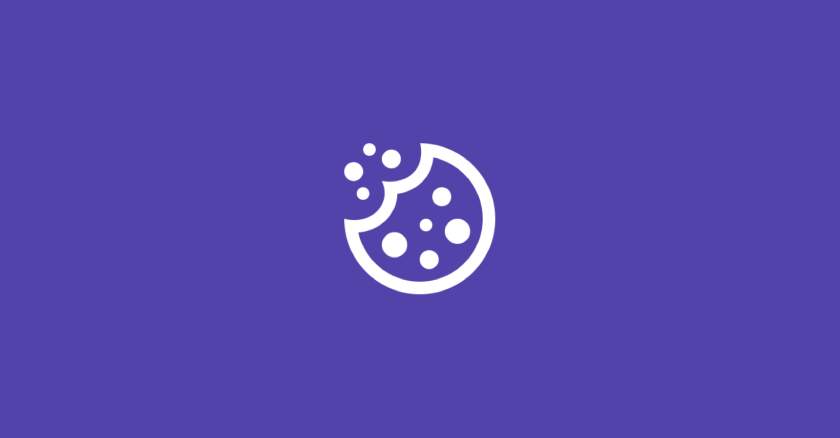
Cookies are like little notes that websites leave on your device when you visit them. They help with things like remembering your preferences, keeping you logged in, and tracking what you do online. In JavaScript, there are handy tools called functions that let you work with cookies easily. In this guide, we’ll walk through how to use these functions to set up, get, and erase cookies, with easy-to-follow examples.
Understanding cookies
Cookies are small files that websites save on your computer. They’re like reminders that help the website remember things about you, such as your username or preferences. They’re used for things like keeping you logged in when you come back to a site and remembering what’s in your shopping cart.
Cookie functions in JavaScript
Here are the main functions we’ll be using to work with cookies:
- setCookie(name, value, days): This function puts a new cookie on your device with a name, value, and optional expiration date.
- getCookie(name): This one retrieves the value of a cookie with a specific name.
- eraseCookie(name): And this function deletes a cookie so it’s no longer stored on your device.
Let’s see how these functions work in action:
const setCookie = ( name, value, days ) => {
let expires = "";
if (days) {
let date = new Date();
date.setTime( date.getTime() + ( days*24*60*60*1000 ) );
expires = "; expires=" + date.toUTCString();
}
document.cookie = name + "=" + (value || "") + expires + "; path=/";
}
const getCookie = ( name ) => {
var nameEQ = name + "=";
var ca = document.cookie.split(';');
for( var i = 0; i < ca.length; i++ ) {
var c = ca[i];
while (c.charAt(0) === ' ') c = c.substring(1,c.length);
if (c.indexOf(nameEQ) === 0) return c.substring(nameEQ.length,c.length);
}
return null;
}
const eraseCookie = ( name ) => {
document.cookie = name +'=; Path=/; Expires=Thu, 01 Jan 1970 00:00:01 GMT;';
}
Once you’ve defined the cookie functions in your JavaScript code, putting them to work is a breeze. All you have to do is call the functions with the right parameters, and they’ll take care of the rest for you. Whether you’re setting up a new cookie, retrieving an existing one, or clearing out outdated information, these functions simplify the process, allowing you to focus on building a seamless user experience.
Setting a cookie
setCookie('username', 'JohnDoe', 7); // Set a cookie named 'username' with the value 'JohnDoe' that lasts for 7 days
Retrieving a cookie:
const username = getCookie('username'); // Get the value of the 'username' cookie
console.log(username); // Output: 'JohnDoe'
Deleting a cookie:
eraseCookie('username'); // Delete the 'username' cookie
Ccheck if a cookie exists:
// Check if the 'visitedBefore' cookie exists
if ( getCookie('visitedBefore') ) {
// If the cookie exists, display a message
console.log('Welcome back to our website!');
} else {
// If the cookie does not exist, set it and display a welcome message
setCookie('visitedBefore', 'true', 30); // Set a cookie indicating the user has visited before (expires in 30 days)
console.log('Welcome to our website for the first time!');
}
In a nutshell, cookies are handy tools that websites use to remember things about you. With JavaScript and the functions we mentioned before you can easily work with cookies in your web projects. Whether you’re keeping track of user preferences or managing logins, these functions make it simple to create a personalized and user-friendly experience for your website visitors.
Was this post helpful? ( Answers: 0 )
Leave a comment
If you enjoyed this post or have any questions, please leave a comment below. Your feedback is valuable!