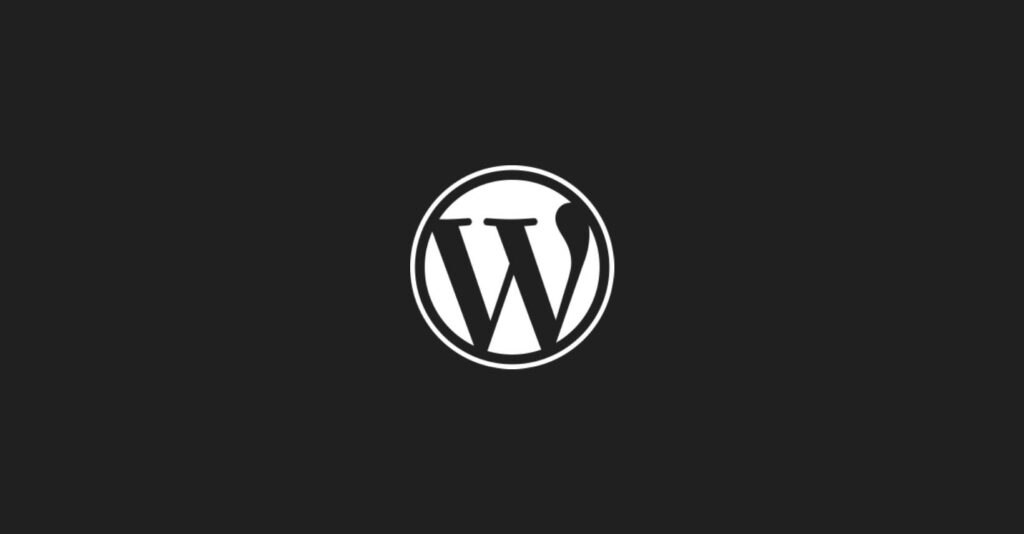
How to replace the default emails in WordPress
Enhance your WordPress site's email communication by learning how to replace default emails with this comprehensive guide.
In the ever-evolving world of WordPress, maintaining a strong online presence and user engagement is crucial. Customizing and optimizing your website’s communication is an essential aspect of this process. By replacing default emails, you can enhance user experience and make your site more distinctive. In this article, we will guide you through the process of replacing default emails in WordPress and provide you with ten examples of filter hooks to get you started.
Why replace default emails?
WordPress uses default email templates for various notifications, such as password change requests, user registration, and more. While these default emails serve their purpose, they often lack personalization and branding. By replacing them, you can:
- Enhance Branding: Customized emails reflect your brand identity and create a more professional appearance.
- Improve User Engagement: Personalized emails are more engaging and can lead to higher user interaction.
- Increase Trust: Users are more likely to trust and open emails that appear customized and tailored to their experience.
Now, let’s dive into the process of replacing default emails using filter hooks. We’ll explore eight useful filters to help you get started.
password_change_email
The password_change_email
filter allows you to customize the content and appearance of the email sent to users when they request a password change. You can modify the subject, message, and sender details.
// custom class for sending emails
use projectsengine\Email;
/**
* Filters the contents of the email sent when the user’s password is changed.
*
* @param pass_change_email array
* @param user array
* @param userdata array
*
*/
function replace_password_change_email( $pass_change_email, $user, $userdata) {
$headers[] = 'Content-Type: text/html';
$object = new Email;
$object->set_email( $user->ID, site_url() . '/contact' , 'Contact' );
$pass_change_email['subject'] = __( 'Projects Engine | Password change confirmation', 'projectsengine' );
$content = __( 'If you did not initiate this change, please <a href="' . site_url() . '/contact">contact</a> our support team immediately.', 'projectsengine' );
$pass_change_email['message'] = $object->email( 'Password change confirmation', $content );
$pass_change_email['headers'] = $headers;
return $pass_change_email;
}
add_filter( 'password_change_email', 'replace_password_change_email', 10, 3 );
wp_mail_from
The wp_mail_from
filter allows you to change the “From” address for all WordPress emails. This is handy for ensuring emails appear to come from a specific address, such as your domain’s contact email.
/**
* Mail from.
*/
public function custom_wp_mail_from( $original_email_address ) {
// Make sure the email is from the same domain
//as your website to avoid being marked as spam.
return '[email protected]';
}
/**
* Name form.
*/
public function custom_wp_mail_from_name( $name ) {
return 'Projects Engine';
}
add_filter( 'wp_mail_from', 'custom_wp_mail_from' );
add_filter( 'wp_mail_from_name', 'custom_wp_mail_from_name' );
email_change_email
The email_change_email
filter lets you customize the email sent to users when they change their email address on your site. You can adjust the email’s content and design to suit your branding.
send_password_ change_email
The send_password_ change_email
filter enables you to modify the parameters of the email sent when a user changes their password. You can alter the email’s subject, message, and sender information.
/**
* Filters whether to send the password change email.
*
* @param bool $send
* @param array $user
* @param array $userdata
* @return void
*/
function replace_send_password_change_email( $send, $user, $userdata ) {
return false;
}
add_filter( 'send_password_change_email' , 'replace_send_password_change_email' );
send_email_ change_email
The send_email_ change_email
filter allows you to customize the email sent to users when they request to change their email address. You can personalize the email’s content and sender details.
/**
* Filters whether to send the email change email.
*
* @param bool $send
* @param array $user
* @param array $userdata
* @return void
*/
function replace_send_email_change_email( $send, $user, $userdata ) {
return false;
}
add_filter( 'send_email_change_email' , 'replace_send_email_change_email' );
auth_cookie_expiration
The auth_cookie_expiration
filter empowers you to change the expiration time for the authentication cookies in WordPress. This is essential for improving security and controlling user sessions.
/**
* Filters the duration of the authentication cookie expiration period.
*
* @param int $length - Duration of the expiration period in seconds.
* @param int $user_id
* @param bool $remember
* @return void
*/
function replace_auth_cookie_expiration( $length, $user_id, $remember ) {
return YEAR_IN_SECONDS;
// MINUTE_IN_SECONDS
// HOUR_IN_SECONDS
// DAY_IN_SECONDS
// WEEK_IN_SECONDS
// MONTH_IN_SECONDS
}
add_filter( 'auth_cookie_expiration' , 'replace_auth_cookie_expiration' );
wp_new_user_ notification_email
The wp_new_user_ notification_email
filter lets you customize the email sent to the new user when a new user registers on your site. You can modify the email’s content and subject.
// custom class for sending emails
use projectsengine\Email;
/**
* Filters the contents of the new user notification email sent to the new user.
*
* @param wp_new_user_notification_email array
* @param user WP_User
* @param blogname string
*
*/
function replace_wp_new_user_notification_email( $wp_new_user_notification_email, $user, $blogname ) {
$headers[] = 'Content-Type: text/html';
$object = new Email;
$object->set_email( $user->ID, site_url() . '/contact' , 'Contact' );
$wp_new_user_notification_email['subject'] = __( $blogname . ' | Welcome new user', 'projectsengine' );
$content = __( 'You created an account with us.', 'projectsengine' );
$wp_new_user_notification_email['message'] = $object->email( 'Welcome new user', $content );
$wp_new_user_notification_email['headers'] = $headers;
return $wp_new_user_notification_email;
}
add_filter( 'wp_new_user_notification_email', 'replace_wp_new_user_notification_email', 10, 3 );
Other hooks: wp_new_user_notification _email_admin, wp_send_new_user_ notification_to_admin, wp_send_new_user_ notification_to_user.
user_request_confirmed_ email_subject
The user_request_confirmed_ email_subject
filter allows you to personalize the subject of the email sent to users when their request has been confirmed on your WordPress site. This is particularly useful for scenarios where users make requests, and you want to provide a more informative or engaging subject line to enhance their experience.
function custom_user_request_confirmed_email_subject( $subject, $sitename, email_data ) {
return 'Your request has been confirmed!';
}
add_filter('user_request_confirmed_email_subject', 'custom_user_request_confirmed_email_subject', 10, 3 );
By using these filter hooks, you can replace default emails in WordPress and create a more engaging and branded experience for your users. Customizing emails with these filters allows you to control the content, appearance, and branding of your communication, ultimately improving user engagement. Give it a try and watch your WordPress site thrive with enhanced email communications.