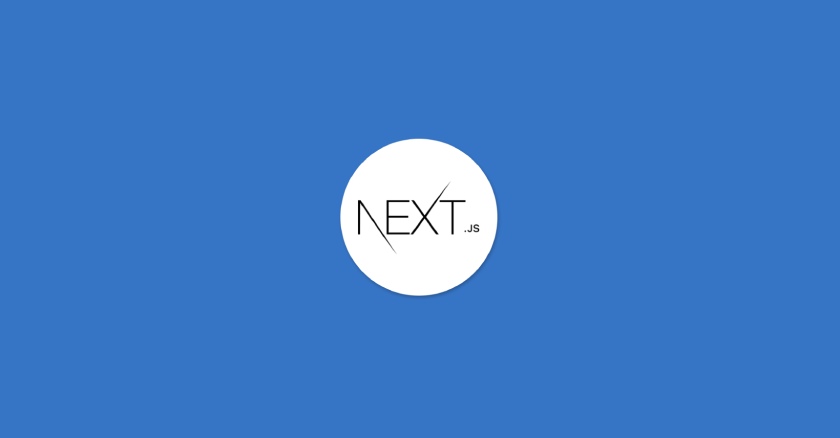
How to Get Started with Next.js
Starting with Next.js can be an exciting journey for web developers. Next.js is a React framework that enables functionality such as server-side rendering (SSR), static site generation (SSG), and more, making it an excellent choice for building modern web applications.
Starting with Next.js can be an exciting journey for web developers. Next.js is a React framework that enables functionality such as server-side rendering (SSR), static site generation (SSG), and more, making it an excellent choice for building modern web applications.
Prerequisites:
- Node.js: You’ll need Node.js version 18 or higher installed on your system. You can download it from the official website: https://nodejs.org/
- Basic understanding of HTML, CSS, and React: While not mandatory, understanding these will make learning Next.js much easier. There are many resources available online if you need to brush up on your skills.
Setting Up Your Environment
- Install the
create-next-app
tool: Open your terminal and run the following command:
npm install -g create-next-app
- Create a new Next.js project: Navigate to your desired project directory and run:
npx create-next-app my-next-js-app
Replace my-next-js-app
with your desired project name.
- Start the development server: Run the following command:
npm run dev
This will launch the development server and open your Next.js app in the browser (usually at http://localhost:3000).
The command npx create-next-app@latest
is a great way to get started with a new Next.js project quickly and easily. Here’s what happens when you run it:
- Download and setup: It automatically downloads and sets up the necessary tools and dependencies for a Next.js project. This includes the Next.js framework itself, as well as other essential packages like React and Webpack.
- Project creation: It creates a new directory with the name you choose (default is “my-app”).
- Interactive prompts: They guide you through some basic configuration options through an interactive process. You can choose things like:
- Whether to use TypeScript
- Whether to use ESLint
- Whether to use Tailwind CSS
- Whether to use the
src
directory for code organization - Whether to include App Router
- Custom import alias for components
- Installation completes: Once you finish answering the prompts, it installs all the required dependencies and prepares your project for development.
Overall, npx create-next-app@latest
is a convenient way to bootstrap a new Next.js project, taking care of all the initial setup steps so you can start building your application right away.
Creating directories with App routes and Layouts in Next.js
The App Router in Next.js 13 allows you to structure your project and define layouts easily. Here’s how:
1. Directories based on routes:
- Each folder within the
app
directory represents a route segment. - Example:
app/products/categories
defines the/products/categories
route. - A
page.js
file within a folder defines the page content. - Example:
app/products/categories/page.js
defines the/products/categories
page.
2. Nested routes with nested directories:
- Create subfolders within existing folders for nested routes.
- Example:
app/products/categories/product/id.js
defines/products/categories/:product/id
route.
3. Layouts for shared UI:
- Create a
layout.js
file within a folder to define a layout for that folder and its subfolders. - The
layout.js
component receives achildren
prop containing the page content. - Use nested layouts for further hierarchy.
- Example:
app/products/layout.js
defines a layout for all/products
routes.
Here’s a basic example with a layout and nested routes:
app/
products/
layout.js // Layout for all products routes
categories/
page.js // /products/categories
product/
id.js // /products/categories/:product/id
page.js // /products
about/
page.js // /about
In this example, the products/layout.js
defines a shared layout for all pages under /products
, and the product/id.js
page utilizes it.
Additional considerations:
- You can create layouts for specific routes by placing
layout.js
within the corresponding folder. - The root
app/layout.js
defines the overall app layout. - Remember to export the default component from each
layout.js
andpage.js
file.
Project Structure in Next.js
Next.js offers a well-defined project structure that combines file-based routing with customization options for optimal organization. Here’s a breakdown of the key elements:
Top-level folders:
- public: Contains static assets like images, fonts, and other files directly served by the server.
- styles: Houses global CSS stylesheets applying to the entire application.
- lib: Holds reusable components, utilities, or custom libraries not directly related to specific pages.
- node_modules: (Generated) Contains downloaded dependencies managed by npm or yarn.
- .next: (Generated) Internal directory used by Next.js for build outputs and caching.
Core directories:
- app: Houses your application’s routing and content structure.
- Folders within
app
represent route segments (e.g.,app/products
). - Files within a folder define the content for that route (e.g.,
app/products/page.js
). - Use nested folders for deeper routes (e.g.,
app/products/categories/product/id.js
). - Optional
layout.js
file within a folder defines a layout for that folder and its subfolders.
- Folders within
- pages: Contains standalone pages not part of the
app
directory’s routing structure (e.g.,pages/404.js
). - components: Houses reusable React components used throughout your application.
Important files:
- _app.js: (Optional) Defines global application-wide state and context.
- _document.js: (Optional) Customizes the HTML document structure (e.g., adding meta tags).
Routing conventions:
- File names within
app
folders define routes (e.g.,app/products/categories.js
maps to/products/categories
). index.js
within a folder maps to its parent route (e.g.,app/products/index.js
maps to/products
).- Dynamic routes use square brackets (e.g.,
app/products/[id].js
for/products/:id
).
Flexibility:
- You can create custom directory structures within
app
andpages
based on your project needs. - Layouts provide flexibility for shared UI elements across pages.
- Utilize
app
for complex routing and components for reusability.
Tips:
- Keep the structure organized and consistent for maintainability.
- Use
pages
for simpler standalone pages. - Consider using TypeScript for type safety and better code organization.
Remember, this is a general overview, and the optimal structure might vary based on your specific project requirements. Don’t hesitate to adapt and customize as needed!
Client Components in Next.js
In Next.js, client components refer to components that execute and render directly in the user’s browser. They are distinct from server components, which render on the server before being sent to the client. Here’s a breakdown of key points:
What they are:
- Written in JavaScript using React syntax.
- Executed and rendered dynamically on the client side.
- Offer advantages like interactivity, dynamic state management, and access to browser APIs.
- Examples: interactive forms, user authentication, real-time data updates.
How they work:
- By default, all components in Next.js are assumed to be client components.
- You can explicitly mark a component as a client component using the
use client
directive at the top of the file. - This helps optimize bundle size and server performance by keeping server-side rendering focused on static content.
When to use them:
- Prefer client components for functionalities that require browser-specific features like local storage, geolocation, or WebSockets.
- Use them for interactive UI elements like forms, user profiles, or real-time chat features.
- Consider client components for dynamic data fetching triggered by user interactions.
Things to remember:
- Client components can take longer to load and interact with compared to server components.
- They may not be suitable for initial page content requiring fast rendering.
- Use
getStaticProps
orgetServerSideProps
for data fetching in client components when needed.
Client Component Examples in Next.js
Here are some examples of how you might use client components in Next.js:
1. Interactive Form:
// components/ContactForm.js
import { useState } from 'react';
function ContactForm() {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const [message, setMessage] = useState('');
const handleSubmit = (event) => {
event.preventDefault();
// Handle form submission using client-side logic (e.g., AJAX)
};
return (
<form onSubmit={handleSubmit}>
<label htmlFor="name">Name:</label>
<input type="text" id="name" value={name} onChange={(e) => setName(e.target.value)} />
<label htmlFor="email">Email:</label>
<input type="email" id="email" value={email} onChange={(e) => setEmail(e.target.value)} />
<label htmlFor="message">Message:</label>
<textarea id="message" value={message} onChange={(e) => setMessage(e.target.value)} />
<button type="submit">Send</button>
</form>
);
}
export default ContactForm;
2. Real-time Chat Feature:
// components/Chat.js
import { useEffect, useState } from 'react';
function Chat() {
const [messages, setMessages] = useState([]);
useEffect(() => {
// Use a WebSocket or polling to fetch messages from a server
const fetchMessages = async () => {
// ...fetch data
setMessages([...messages, data]);
};
fetchMessages();
}, []);
// ...functionality for sending new messages
return (
<div>
<h2>Chat</h2>
{/* Display messages */}
{/* Form for sending new messages */}
</div>
);
}
export default Chat;
3. User Profile with Local Storage:
// components/UserProfile.js
import { useEffect, useState } from 'react';
function UserProfile() {
const [user, setUser] = useState(null);
useEffect(() => {
const localUser = localStorage.getItem('user');
if (localUser) {
setUser(JSON.parse(localUser));
}
}, []);
// ...functions for updating user profile and storing in local storage
return (
<div>
{user ? (
<>
<h2>Welcome, {user.name}!</h2>
{/* Display user information */}
{/* Edit profile form */}
</>
) : (
<p>Please log in.</p>
)}
</div>
);
}
export default UserProfile;
These are just a few examples, and you can adapt them to fit your specific needs. Remember, use client components when browser-specific features or dynamic user interactions are crucial. For static content or SEO purposes, consider server-side rendering.
CSS Modules in Next.js
CSS Modules are a technique for scoping CSS styles to specific components in your application. This helps prevent conflicts and promotes better maintainability by ensuring styles are isolated to their respective components.
Here’s a breakdown of key points about CSS Modules:
What they are:
- CSS files where class names are scoped locally by default.
- Class names are automatically transformed into unique identifiers during build time.
- This prevents accidental style overrides from other parts of your project.
- Popular in React development and used with tools like Webpack or Rollup.
How they work:
- You create a CSS file with the
.module.css
extension (e.g.,MyComponent.module.css
). - Define your styles within this file using regular CSS syntax.
- When you import the CSS file into your component, it gets transformed, and:
- Class names are prefixed with a unique hash (e.g.,
MyComponent_myClass__3a9b1
). - Only styles associated with that specific component are applied.
- Class names are prefixed with a unique hash (e.g.,
Benefits:
- Avoids global styles collisions: Ensures styles stay confined to their components.
- Improves code organization: Keeps styles clearly linked to their corresponding components.
- Promotes reusability: Allows components to have their own independent styles.
- Enables dynamic class names: Useful for conditional styling or state-based styling.
Things to remember:
- You need a bundler or build tool configured to handle CSS Modules transformations.
- Class names become less readable due to the added hash.
- Consider using CSS-in-JS solutions for more advanced styling needs.
Examples:
// MyComponent.js
import styles from './MyComponent.module.css';
function MyComponent() {
return (
<div className={styles.container}>
<h1 className={styles.title}>My Component</h1>
</div>
);
}
export default MyComponent;
// MyComponent.module.css
.container {
background-color: #f0f0f0;
padding: 20px;
}
.title {
font-size: 24px;
font-weight: bold;
}
Sass Modules in Next.js
Sass Modules offer an even more powerful and convenient way to manage component-specific styles in your Next.js projects compared to vanilla CSS Modules. Here’s a breakdown:
What they are:
- An extension of CSS Modules that leverages the power of Sass preprocessor syntax.
- Allows you to import Sass files with
.module.scss
extension into your components. - Styles within these files get automatically scoped and transformed like CSS Modules.
- You benefit from additional Sass features like variables, mixins, functions, and nesting for cleaner and more maintainable styles.
How they work:
- Create a Sass file with the
.module.scss
extension (e.g.,MyComponent.module.scss
). - Write your styles using Sass syntax, taking advantage of features like variables and mixins.
- Import the Sass file into your component like a regular CSS module.
- During build time, the Sass is compiled and the class names get prefixed with a unique hash, ensuring scoped styles.
Benefits over CSS Modules:
- Sass features: Utilize variables, mixins, functions, and nesting for cleaner and more organized styles.
- Dynamic class names: Leverage Sass features for conditional styling based on variables or states.
- Better organization: Maintain styles alongside components for improved readability and maintainability.
Example:
JavaScript
// MyComponent.js
import styles from './MyComponent.module.scss';
function MyComponent() {
return (
<div className={styles.container}>
<h1 className={styles.title}>My Component</h1>
</div>
);
}
export default MyComponent;
// MyComponent.module.scss
$primary-color: blue;
.container {
background-color: lighten($primary-color, 20%);
padding: 20px;
}
.title {
font-size: 24px;
font-weight: bold;
color: $primary-color;
}
Learning and Building
- Next.js Documentation: The official Next.js documentation is a great starting point. It covers everything from installation and basic concepts to advanced features and deployment.
- Next.js Learn: This interactive tutorial helps you learn Next.js step-by-step by building a dashboard application.
- Community Resources: Many resources are available online, including tutorials, articles, and videos created by the Next.js community. You can find them on the Next.js website and forums.
Additional Tips
- Choose a code editor or IDE: You can use any code editor you like, but popular choices for web development include Visual Studio Code, Sublime Text, and Atom. Some code editors have specific plugins for Next.js that can enhance your development experience.
- Start small and experiment: Don’t try to build a complex application right away. Start with small projects and gradually learn new features as you go.
- Join the Next.js community: The Next.js community is very active and helpful. You can ask questions on the official forums or join the Discord server: <invalid URL removed>