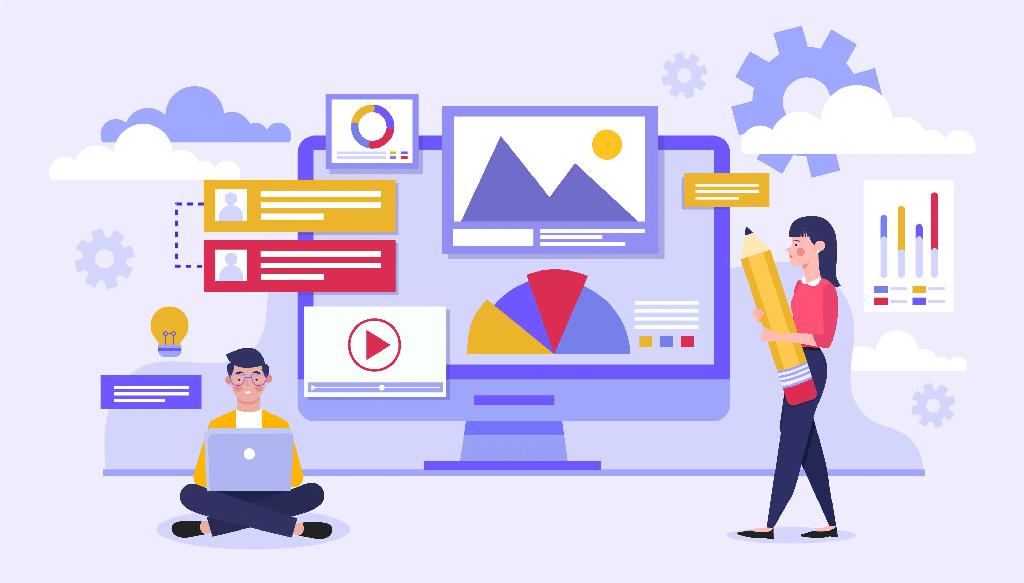
How to Enhance Your WordPress Menu
A WordPress website would be incomplete without menus and submenus. Customizing such menus from within WordPress is equally simple. As a result, your WordPress menu is a “first line” tool for achieving a good search ranking and keeping people on your site.
Table of Contents
Because this is a bit more complicated, we’ll make the following assumptions before proceeding:
- You understand how to use PHP to construct a WordPress menu.
- Your PHP knowledge is sufficient to follow along with certain advanced subjects.
- You understand how to install and activate a WordPress plugin.
Although it is outside the scope of this post, you may utilize the WordPress Plugin Boilerplate Generator to develop a new, standardized plugin template.
When you’re set, generate and upload your plugin to WordPress. You can use your theme’s files to implement this functionality as well.
Also, before you dig in, we recommend reading this article about how to create WordPress Menus.
Next, go to the plugin‘s (or your theme’s) folder and open the main file (if you editing your theme open the functions.php file). Insert the following code here:
/**
* Add menu meta box
*
* @param object $object The meta box object
*
* @link https://developer.wordpress.org/reference/functions/add_meta_box/
*/
function pe_custom_add_menu_meta_box( object $object ) {
add_meta_box( 'pe-menu-metabox', __( 'Tags' ), 'pe_custom_menu_meta_box', 'nav-menus', 'side' );
return $object;
}
add_filter( 'nav_menu_meta_box_object', 'pe_custom_add_menu_meta_box', 10, 1);
The WordPress add_meta_box() function adds a meta box to the WordPress admin interface. There are a few arguments in the official documentation that you should refer to. Also, the nav_menu_meta_box_object() is used because within the nav-menus.php file there is no action to hook into.
Defining a Callback Function
Then, to generate the HTML content for the meta box, we may construct a callback function:
/**
* Displays a metabox for a tag menu item.
*
* @global int|string $nav_menu_selected_id (id, name or slug) of the currently-selected menu
*/
function pe_custom_menu_meta_box(){
global $nav_menu_selected_id;
$walker = new Walker_Nav_Menu_Checklist();
// the rest of the code will be placed here.
}
The global variable stores the current menu ID, whereas $walker keeps a new Walker_Nav_Menu_Checklist object instance. This will generate the HTML list of menu items.
From here, we must decide on the active tab in the custom meta box. To do this, we set the value of $current tab while remaining within the ellipsis defined in the preceding code block.
We’re using three tabs here, but you may add as many as you want:
$current_tab = 'all';
if ( isset( $_REQUEST['tags-tab'] ) && 'wordpress' == $_REQUEST['tags-tab'] ) {
$current_tab = 'wordpress';
} elseif ( isset( $_REQUEST['tags-tab'] ) && 'custom' == $_REQUEST['tags-tab'] ) {
$current_tab = 'custom';
} elseif ( isset( $_REQUEST['tags-tab'] ) && 'all' == $_REQUEST['tags-tab'] ) {
$current_tab = 'all';
}
The following line will retrieve all tags and will store the tags that have the word ‘wordpress’ in them will be stored in the $wordpress array and the tags that have the word ‘custom‘ in them will be stored in the $custom array:
$tags = get_tags(array(
'taxonomy' => 'post_tag',
'orderby' => 'name',
'hide_empty' => false // for development
));
$wordpress = array();
$custom = array();
/* set values to required item properties */
foreach ( $tags as &$tag ) {
$tag->classes = array();
$tag->type = 'tag-custom';
$tag->object_id = $tag->term_id;
$tag->title = $tag->name;
$tag->object = 'tag-custom';
$tag->url = '';
$tag->attr_title = $tag->name;
if( strpos( 'wordpress' , $tags->name ) ) {
$wordpress[] = $tag;
}
if( strpos( 'custom' , $tags->name ) ) {
$custom[] = $tag;
}
}
The meta box markup may now be printed. Let’s start by creating the tab labels and links.
<div id="tagsarchive" class="categorydiv">
<ul id="tags-tabs" class="tags-tabs add-menu-item-tabs">
<li <?php echo ( 'all' == $current_tab ? ' class="tabs"' : '' ); ?>>
<a class="nav-tab-link" data-type="tabs-panel-tags-all" href="<?php if ( $nav_menu_selected_id ) echo esc_url( add_query_arg( 'tags-tab', 'all' ) ); ?>#tabs-panel-tags-all">
<?php _e( 'View All' ); ?>
</a>
</li>
<li <?php echo ( 'wordpress' == $current_tab ? ' class="tabs"' : '' ); ?>>
<a class="nav-tab-link" data-type="tabs-panel-tags-wordpress" href="<?php if ( $nav_menu_selected_id ) echo esc_url( add_query_arg( 'tags-tab', 'wordpress' ) ); ?>#tabs-panel-tags-wordpress">
<?php _e( 'Wordpress' ); ?>
</a>
</li>
<li <?php echo ( 'custom' == $current_tab ? ' class="tabs"' : '' ); ?>>
<a class="nav-tab-link" data-type="tabs-panel-tags-custom" href="<?php if ( $nav_menu_selected_id ) echo esc_url( add_query_arg( 'tags-tab', 'custom' ) ); ?>#tabs-panel-tags-custom">
<?php _e( 'Custom' ); ?>
</a>
</li>
</ul>
<div id="tabs-panel-tags-all" class="tabs-panel tabs-panel-view-all <?php echo ( 'all' == $current_tab ? 'tabs-panel-active' : 'tabs-panel-inactive' ); ?>">
<ul id="authorarchive-checklist-all" class="categorychecklist form-no-clear">
<?php
echo walk_nav_menu_tree( array_map('wp_setup_nav_menu_item', $tags), 0, (object) array( 'walker' => $walker) );
?>
</ul>
</div>
<div id="tabs-panel-tags-wordpress" class="tabs-panel tabs-panel-view-wordpress <?php echo ( 'wordpress' == $current_tab ? 'tabs-panel-active' : 'tabs-panel-inactive' ); ?>">
<ul id="tags-checklist-wordpress" class="categorychecklist form-no-clear">
<?php
echo walk_nav_menu_tree( array_map('wp_setup_nav_menu_item', $wordpress), 0, (object) array( 'walker' => $walker) );
?>
</ul>
</div>
<div id="tabs-panel-tags-custom" class="tabs-panel tabs-panel-view-custom <?php echo ( 'custom' == $current_tab ? 'tabs-panel-active' : 'tabs-panel-inactive' ); ?>">
<ul id="tags-checklist-custom" class="categorychecklist form-no-clear">
<?php
echo walk_nav_menu_tree( array_map('wp_setup_nav_menu_item', $custom), 0, (object) array( 'walker' => $walker) );
?>
</ul>
</div>
<p class="button-controls wp-clearfix">
<span class="list-controls">
<a href="<?php echo esc_url( add_query_arg( array( 'tags-tab' => 'all', 'selectall' => 1, ) )); ?>#tagsarchive" class="select-all"><?php _e('Select All'); ?></a>
</span>
<span class="add-to-menu">
<input type="submit"<?php wp_nav_menu_disabled_check( $nav_menu_selected_id ); ?> class="button-secondary submit-add-to-menu right" value="<?php esc_attr_e('Add to Menu'); ?>" name="add-tags-menu-item" id="submit-tagsarchive" />
<span class="spinner"></span>
</span>
</p>
</div>
<?php
The final result should look like this:
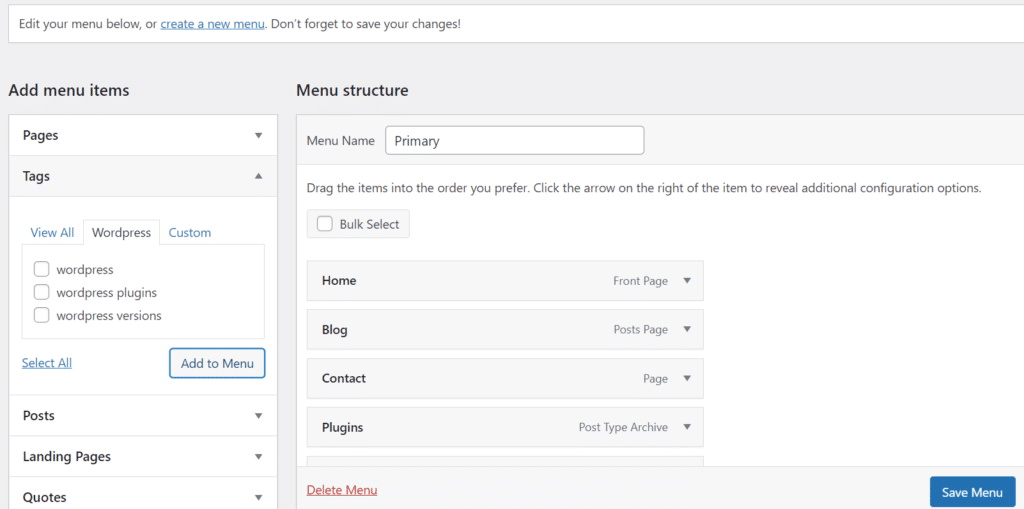
Remember to provide the meta box components the right class names, IDs, and data properties; otherwise, the menu will not function properly.
You can add practically any feature to your menus with this knowledge! It’s worth noting that the plugin has a public Gist on GitHub that you may download for free.
Summary
A WordPress menu is an essential component of your website. As a result, the platform includes a native and powerful panel that allows you to personalize any menu on your site. However, it will not offer you all you require by default.