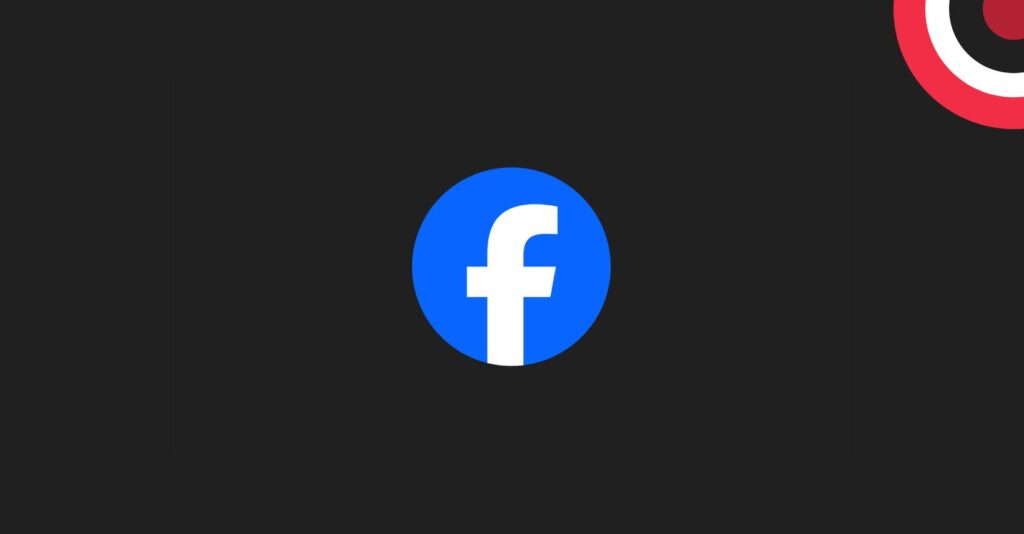
How to Create Facebook Login with the JavaScript SDK in WordPress
To enable Facebook login functionality on your WordPress website, you need to create a Facebook app and obtain the App ID.
In today’s digital age, social media platforms play a vital role in connecting people and facilitating seamless user experiences. Integrating social login functionality into your WordPress website can significantly enhance user engagement and simplify the registration process. In this comprehensive guide, we will explore how to create a Facebook login using the JavaScript Software Development Kit (SDK) in WordPress. Follow the step-by-step instructions below to empower your users with a convenient and efficient login experience.
Set up a Facebook Developer Account
Before diving into the implementation process, you need to have a Facebook Developer account. If you don’t already have one, visit the Facebook Developer website and create an account. Once your account is set up, proceed to create a new app.
Create an App
To enable Facebook login functionality on your WordPress website, you need to create a Facebook app and obtain the App ID. Inside your Facebook Developer account, navigate to the “My Apps” section and click on “Create App.” Provide a name for your app, select the appropriate category, and create your app. Once your app is created, you’ll be redirected to the app dashboard. Go to the “Settings” tab and under the “Basic” section, you will find your App ID. This ID is crucial for the integration process.
Load the Facebook SDK for JavaScript
You don’t need to download or install any standalone files for the Facebook SDK for JavaScript. Instead, you can include a small snippet of regular JavaScript in your HTML code, which will dynamically load the SDK into your pages. This asynchronous loading method ensures that it won’t hinder the loading of other elements on your page.
<script>
window.fbAsyncInit = function() {
FB.init({
appId : 'your-app-id',
autoLogAppEvents : true,
xfbml : true,
version : 'v17.0'
});
};
</script>
<script async defer crossorigin="anonymous" src="https://connect.facebook.net/en_US/sdk.js"></script>
Log in with Facebook
To get started, add a visually appealing and clearly labeled button on your website, inviting users to log in with their Facebook accounts. Upon clicking the button, the JavaScript function associated with the button’s click event should call the FB.login
method from the Facebook JavaScript SDK.
The FB.login
method triggers a Facebook login dialog, prompting users to provide their Facebook credentials and authorize your app. Upon successful login, the SDK provides your application with an access token, allowing you to authenticate the user and retrieve necessary profile information.
const facebookLogin = $('a.facebook');
facebookLogin.on('click', function () {
FB.login(function(response) {
if ( 'connected' === response.status ) {
access_token = response.authResponse.accessToken; //get access token
user_id = response.authResponse.userID; //get FB UID
$.ajax({
url: '/wp-json/namespace/facebook-login',
type: 'GET',
data: { access_token, user_id },
headers: { 'content-type': 'application/json' },
}).done(function (data) {
const user = data.user;
window.location.href = '/verify?type=facebook&user=' + user;
});
} else {
console.log('User cancelled login or did not fully authorize.');
}
}, {
scope: 'public_profile,email'
});
});
In addition to implementing the “Log in with Facebook” feature, you can also leverage AJAX to create a user on your website using the retrieved Facebook data.
In the example above, we are using a custom route for the AJAX call. Also, the jQuery code is added in a file that is enqueued to the website after loading the Facebook SDK.
Get user information
/**
* Get facebook user information with access token.
*/
public function facebook_me( WP_REST_Request $request ) {
$params = $request->get_params();
$userID = $params['user_id'];
$accessToken = $params['access_token'];
$curl = curl_init();
$url = 'https://graph.facebook.com/v17.0/';
curl_setopt_array($curl, array(
CURLOPT_URL => $url . $userID . '/?fields=id,first_name,last_name,email,picture&access_token=' . $accessToken,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$response = curl_exec($curl);
$json_respose = json_decode( $response );
curl_close( $curl );
if( isset( $json_respose->error ) ) {
$message = __( $json_respose->error->message, $this->theme_domain );
return new WP_REST_Response( ['response' => false, 'message' => $message, 'class' => 'alert-error' ] );
}
$createUser = $this->create_user( $json_respose, $userID, $accessToken );
return new WP_REST_Response( $createUser );
}
The provided code is a PHP function that retrieves Facebook user information using an access token. The function is named facebook_me
and accepts a WordPress REST API request object as a parameter.
Inside the function, the code retrieves the request parameters from the REST API request object. Specifically, it extracts the user_id
and access_token
from the parameters using the $request->get_params()
method.
To retrieve the user information from Facebook’s Graph API, the code initializes a cURL session using curl_init()
. It sets the URL to this
and appends the user_id
, fields to fetch (such as id
, first_name
, last_name
, email
, picture
), and the access_token
.
The cURL options are set using curl_setopt_array()
. These options include settings like enabling the return of the response as a string (CURLOPT_RETURNTRANSFER
), following any redirects (CURLOPT_FOLLOWLOCATION
), and specifying the HTTP version to use (CURLOPT_HTTP_VERSION
).
The code then performs a GET request to the specified URL using curl_exec()
. The response is stored in the $response
variable.
Next, the response is decoded from JSON format to a PHP object using json_decode()
and stored in the $json_response
variable.
After the cURL request is complete, the session is closed using curl_close()
.
If an error occurs during the API call (checked by isset( $json_response->error )
), the function retrieves the error message from the response object and returns a WordPress REST API response with the error message.
If the API call is successful, the function calls the create_user
method, passing the decoded JSON response, user_id
and access_token
as parameters. The result of the create_user
method is returned as a WordPress REST API response.
In summary, this PHP function utilizes cURL to retrieve Facebook user information by making a GET request to the Facebook Graph API. It handles any potential errors and returns the user information or an error message through a WordPress REST API response.
Create a user with the received information from the GET request. Just make sure to return the ID of the newly created user because later we are going to use it to log in the user without a password.
Log in
On successful request, the user will be redirected to the verify page.
window.location.href = '/verify?type=facebook&user=' + user;
As parameters, we’ll send a type and the user ID. When the user accesses the page you can set up another AJAX call where another custom route can be called. Make sure to send the user ID.
const queryString = window.location.search;
const urlParams = new URLSearchParams(queryString);
const type = urlParams.get('type');
const user = urlParams.get('user');
On load send the AJAX call with this information. Make sure that this part of the code is only loaded on that page. You can add simple if
condition.
/**
* Log in the user without password.
*/
public function social_login( WP_REST_Request $request ) {
$fields = $request->get_params();
$userID = $fields['user'];
wp_clear_auth_cookie();
wp_set_current_user( $userID );
wp_set_auth_cookie( $userID );
return new WP_REST_Response( [ 'response' => true, 'message' => __( 'You are logged in.', $this->theme_domain ), 'class' => 'alert-success' ] );
}
Log in the user return the response for the AJAX call and reload the page. The user will be logged in and all of the user’s information is already stored in our database.
Log out
The FB.logout
function
is a part of the Facebook JavaScript SDK and is used to log the user out of their Facebook session. When called, it will log the user out of Facebook and revoke the app’s access to the user’s Facebook account.
FB.logout(function(response) {
if ( 'connected' === response.status ) {
window.location.href = '/';
} else {
console.log( 'There has been an error.' );
}
}, {
access_token: accessToken
});
If you don’t use the access token the request might throw an error like this:
FB.logout() called without an access token
To use the access token with the FB.logout method, you need to ensure that you have obtained the access token during the login process and that it is available when you want to log out the user.
Delete user permissions
this code allows you to delete user permissions on Facebook by making a DELETE request to the Facebook Graph API. The function uses cURL to handle the HTTP request and response, and it returns the decoded JSON response.
/**
* Delete user permissions.
*/
public function fb_delete_user_permissions( $fbUserID, $fbAccessToken ) {
$curl = curl_init();
$url = 'https://graph.facebook.com/v17.0/';
curl_setopt_array($curl, array(
CURLOPT_URL => $url . $fbUserID . '/permissions?access_token=' . $fbAccessToken,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'DELETE',
));
$response = curl_exec($curl);
curl_close( $curl );
return json_decode( $response );
}
Get login status
The FB.getLoginStatus
function is a part of the Facebook JavaScript SDK and is used to retrieve the login status of a user. It determines whether a user is currently logged in to Facebook and whether your app has been authorized by the user.
Here’s an example of how to use FB.getLoginStatus
in JavaScript:
FB.getLoginStatus(function(response) {
if (response.status === 'connected') {
// User is logged in and has authorized the app
var accessToken = response.authResponse.accessToken;
console.log('User is logged in with access token: ' + accessToken);
} else if (response.status === 'not_authorized') {
// User is logged in to Facebook but has not authorized the app
console.log('User is logged in but has not authorized the app.');
} else {
// User is not logged in to Facebook
console.log('User is not logged in to Facebook.');
}
});
In the above code, the FB.getLoginStatus
function
is called, and a callback function is provided as an argument. This callback function will be executed with the response object containing the login status information.
Inside the callback function, the response.status
is checked to determine the user’s login status. If the response.status
is 'connected'
, it means the user is logged in and has authorized the app. The accessToken
is then extracted from the response.authResponse
object. A message is logged to the console indicating the user’s login status and displaying the access token.
If the response.status
is 'not_authorized'
, it means the user is logged in to Facebook but has not authorized the app. A different message is logged to the console.
If the response.status
is neither 'connected'
nor 'not_authorized'
, it means the user is not logged in to Facebook. Another message is logged accordingly.
By using FB.getLoginStatus
, you can check the login status of the user and take appropriate actions based on the response. This can help in providing personalized experiences, redirecting users to the login page if necessary, or displaying content based on their authorization status.
Conclusion
Integrating Facebook login using the JavaScript SDK into your WordPress website offers a seamless and convenient way for users to sign in and engage with your content. By following the step-by-step instructions outlined in this guide, you can successfully implement Facebook login functionality in WordPress, empowering your users with a streamlined login experience. Enhance user engagement and simplify registration by leveraging the power of social media integration in your WordPress site.