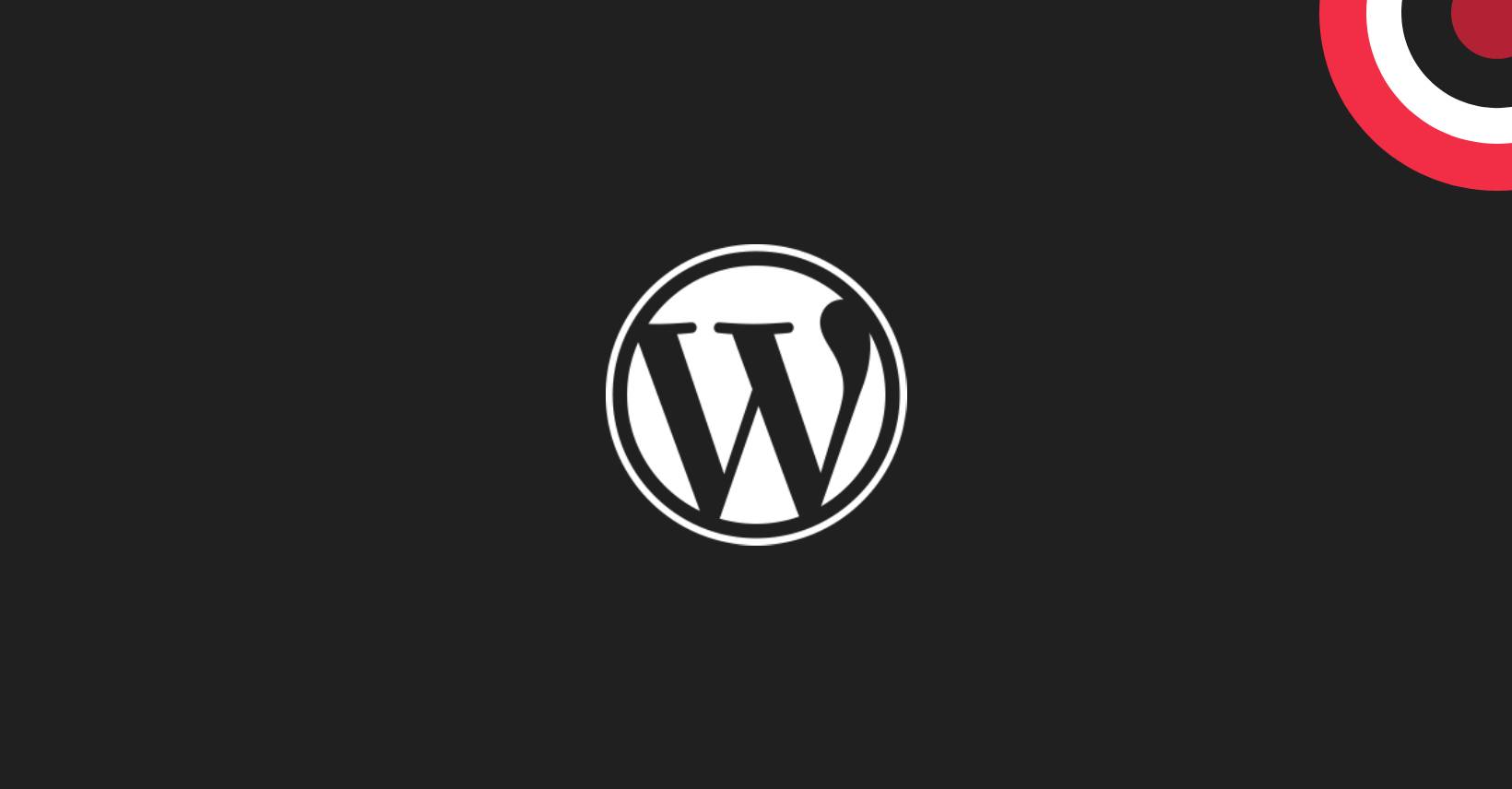
How to create custom post types without plugins using OOP
Discover a streamlined method for creating custom post types in WordPress without plugins. Follow our guide on implementing OOP for a cleaner, efficient website structure.
WordPress extends beyond its original blogging purpose. With custom post types, you can seamlessly integrate and showcase content beyond standard posts or pages. This adaptation transforms your WordPress site into a powerful Content Management System (CMS), providing versatility beyond the conventional blogging framework.
What is a custom post type (CPT)?
WordPress comes equipped with several standard post types, each serving a specific purpose to help you organize and display content effectively. These standard post types include:
- Post: The default and most common post type in WordPress, used for blog posts and regular content updates.
- Page: Unlike posts, pages are static and are typically used for timeless content such as About Us, Contact, and other permanent information.
- Attachment: Handles attachments like images and files, connecting them to the content within your site.
- Revision: Stores revisions of your content, allowing you to revert to earlier versions if needed.
- Navigation Menu: Represents individual items in custom menus, enabling you to organize and structure your site’s navigation.
While these standard post types cover a broad range of content, WordPress also offers the flexibility to create custom post types tailored to your specific needs. This customization allows you to extend WordPress beyond its default capabilities, making it a versatile platform for diverse types of content management.
Why use OOP for custom post types?
Object-Oriented Programming brings a structured and modular approach to coding. By implementing OOP principles, you can create custom post types in WordPress with better organization, scalability, and maintainability.
How to create a custom post type in WordPress?
Start by creating a new PHP class dedicated to handling custom post types. This class will encapsulate the functionalities related to your post type.
class Custom_Post_Type_Manager {
// Class methods and properties go here
}
Inside your class, set up a constructor method to initialize the necessary actions.
class Custom_Post_Type_Manager {
public function __construct() {
add_action('init', array($this, 'register_custom_post_type'));
}
// Additional methods go here
}
Create a method within your class to define labels and arguments for your custom post type.
public function register_custom_post_type() {
$labels = array(
'name' => _x('Custom Posts', 'Post Type General Name', 'text_domain'),
// Add more labels as needed
);
$args = array(
'labels' => $labels,
'public' => true,
// Add more arguments as needed
);
register_post_type('custom_post_type', $args);
}
Finally, instantiate your class to initiate the process.
// Instantiate the class
new Custom_Post_Type_Manager();
Extend the class with a method for registering a portfolio custom post type.
public function register_portfolio_post_type() {
$labels = array(
'name' => _x('Portfolio Items', 'Post Type General Name', 'text_domain'),
// Add more labels as needed
);
$args = array(
'labels' => $labels,
'supports' => array('title', 'editor', 'thumbnail', 'custom-fields'),
// Add more arguments as needed
);
register_post_type('portfolio', $args);
}
Similarly, create a method for registering a real estate custom post type.
public function register_real_estate_post_type() {
$labels = array(
'name' => _x('Real Estate Listings', 'Post Type General Name', 'text_domain'),
// Add more labels as needed
);
$args = array(
'labels' => $labels,
'supports' => array('title', 'editor', 'thumbnail', 'custom-fields'),
// Add more arguments as needed
);
register_post_type('real_estate', $args);
}
Continue to extend your class with methods for each custom post type.
Advanced OOP techniques for custom post types
Use inheritance to create a base class for common functionalities and then extend it for specific post types.
class Base_Post_Type {
// Common functionalities go here
}
class Portfolio_Post_Type extends Base_Post_Type {
// Specific functionalities for portfolio post type go here
}
Use encapsulation to protect your class properties and methods, preventing unintended interference.
class Custom_Post_Type_Manager {
private $post_type_name = 'custom_post_type';
public function get_post_type_name() {
return $this->post_type_name;
}
}
Conclusion
By implementing OOP principles for creating custom post types in WordPress, you elevate your website’s architecture to new heights. This approach provides a clean, organized, and scalable solution for managing diverse content types without relying on plugins. Experiment with the examples provided to craft a tailored, efficient website that aligns with your unique needs.