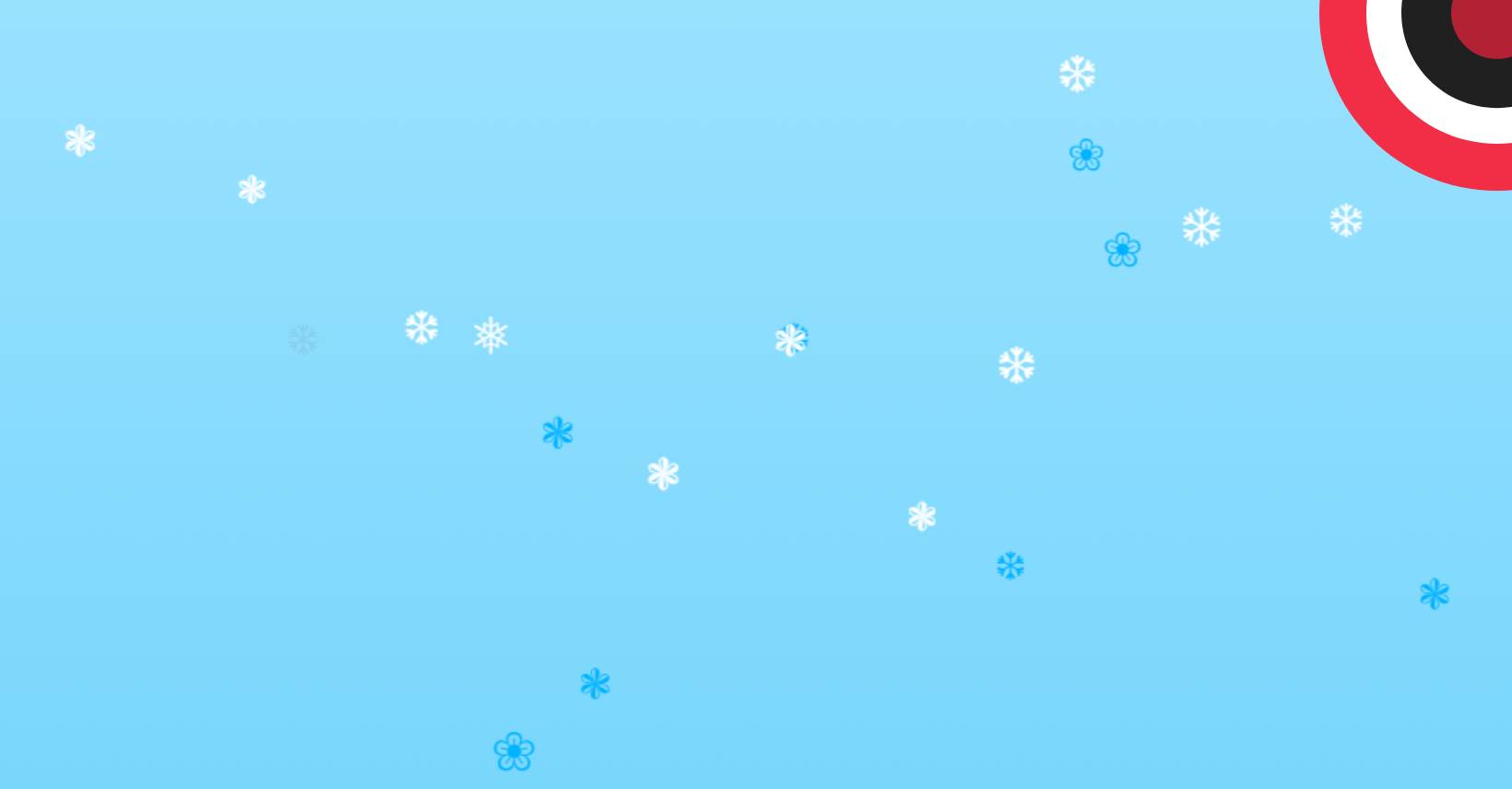
How to Create Animated Snowfall on a Website with CSS and JavaScript
Winter is a magical season, and adding a touch of snowfall to your website can create a delightful and festive atmosphere.
Table of Contents
Winter is a magical season, and adding a touch of snowfall to your website can create a delightful and festive atmosphere. In this tutorial, we’ll explore how to bring the charm of animated snowfall to your web pages using a combination of CSS and JavaScript.
Setting the stage
Before we dive into the code, make sure you have a container element in your HTML where the snowflakes will fall. Give it a unique identifier; for example, we’ll use “snow-container
“.
<div id="snow-container"></div>
The JavaScript magic
Let’s start by writing the JavaScript code that will dynamically generate and animate the snowflakes. This code creates a function to generate random styles for the snowflakes and another function to handle their creation and removal.
const snowContainer = document.getElementById("snow-container");
const snowContent = ['❄', '❅', '❆', '❃', '❀']
const random = (num) => {
return Math.floor(Math.random() * num);
}
const getRandomStyles = () => {
const top = random(100);
const left = random(100);
const dur = random(10) + 10;
const size = random(10) + 25;
return `
top: -${top}%;
left: ${left}%;
font-size: ${size}px;
animation-duration: ${dur}s;
`;
}
const createSnow = (num) => {
for(let i = num; i > 0; i--) {
const snow = document.createElement("div");
snow.className = "snow snow-" + i;
snow.style.cssText = getRandomStyles();
snow.innerHTML = snowContent[random(5)]
snowContainer.append(snow);
}
}
const removeSnow = () => {
snowContainer.style.opacity = "0";
setTimeout(() => {
snowContainer.remove()
}, 500)
}
if( snowContainer ){
window.addEventListener("load", () => {
createSnow(50)
setTimeout(removeSnow, (2000 * 60))
});
window.addEventListener("click", () => {
removeSnow();
});
}
Styling with CSS
Now, let’s add some CSS to make the snowflakes visually appealing and enable smooth animations. Create a style block or link to an external stylesheet in your HTML file.
#snow-container {
height: 100%;
overflow: hidden;
position: absolute;
top: 0;
transition: opacity 500ms;
width: 100%;
}
.snow {
animation: fall ease-in infinite, sway ease-in-out infinite;
color: #fff;
position: absolute;
}
@for $i from 1 through 50 {
@if ( 2*$i + 4 ) < 50 {
.snow-#{2*$i + 3} {
color: #87ceeb;
}
}
}
@for $i from 1 through 50 {
@if ( 2*$i + 4 ) < 50 {
.snow-#{2*$i + 5} {
color: #00b3ff;
}
}
}
@keyframes fall {
0% {
opacity: 0;
}
50% {
opacity: 1;
}
100% {
top: 100vh;
opacity: 1;
}
}
@keyframes sway {
0% {
margin-left: 0;
}
25% {
margin-left: 50px;
}
50% {
margin-left: -50px;
}
75% {
margin-left: 50px;
}
100% {
margin-left: 0;
}
}
With these simple steps, you can add a touch of winter magic to your website. Experiment with different styles, timings, and snowflake characters to create a snowfall effect that suits your site’s theme. Happy coding and enjoy the winter vibes on your web pages.
See the Pen How to Create Animated Snowfall on a Website with CSS and JavaScript by Projects Engine (@projectsengine) on CodePen.
Now that you have the JavaScript and CSS code, simply include them in your HTML file.