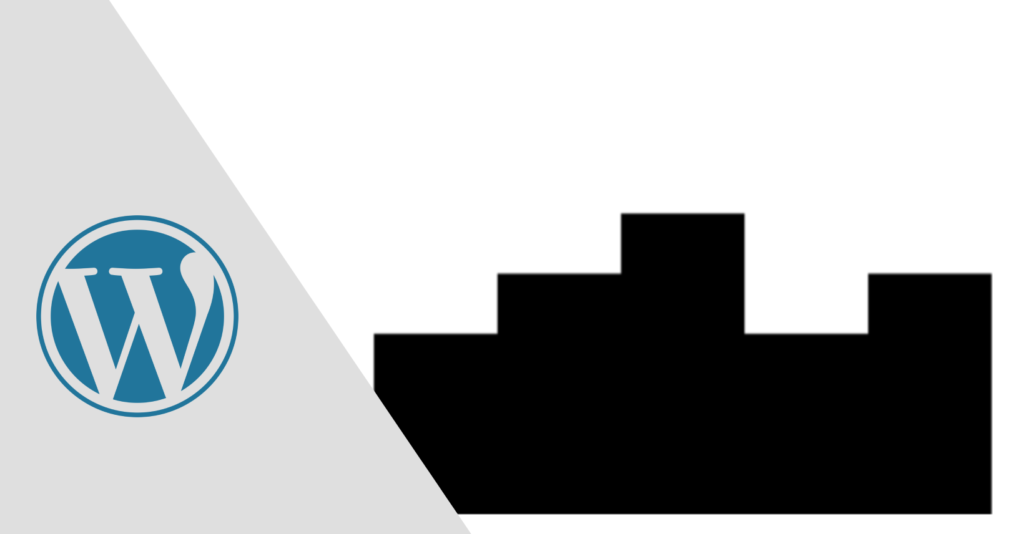
How to create a simple bar graph using the HTML5 Canvas API
HTML5 Canvas is a new web technology that allows developers to create dynamic, interactive graphics and animations directly in a web page without the need for plugins or additional software.
HTML5 Canvas is a new web technology that allows developers to create dynamic, interactive graphics and animations directly in a web page without the need for plugins or additional software. The canvas element is essentially a blank, rectangular space within an HTML document that can be drawn on using JavaScript.
One of the key benefits of using canvas is that it is a vector-based graphics format, which means that it can scale to any size without losing resolution or clarity. This makes it an ideal solution for visualizing data, such as graphs, charts, and other types of data visualization.
To use canvas, you need to start by creating a canvas element in your HTML document. This is done by adding the following code:
<canvas id="myCanvas" width="400" height="400"></canvas>
You can then use JavaScript to draw on the canvas. There are several ways to do this, but one of the most common is to use the 2D drawing API provided by HTML5 Canvas. This API provides a set of drawing functions that can be used to draw shapes, lines, images, and more on the canvas.
Here is an example of how to create a simple bar graph using the HTML5 Canvas API:
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
// Set up data for the graph
var data = [20, 40, 60, 80, 100, 60, 80];
var barWidth = 50;
var barHeight = 100;
// Draw the bars.
for (var i = 0; i < data.length; i++) {
ctx.fillRect(i * barWidth, canvas.height - data[i], barWidth, data[i]);
}
In this example, we start by getting a reference to the canvas element and the 2D context that we will use to draw on the canvas. Next, we set up some data for the graph. In this case, we have an array of values that we will use to determine the height of each bar. We also set the width and height of the bars.
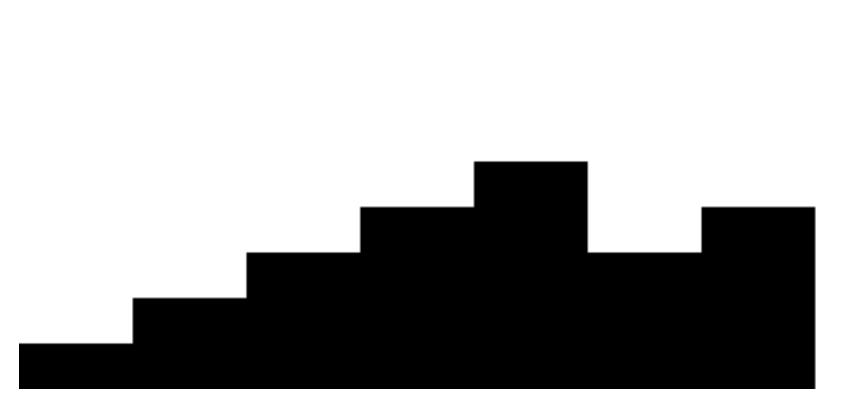
Finally, we use a for loop to draw each bar on the canvas. We use the fillRect
function to draw a rectangle on the canvas, with the position and size determined by the data array and the barWidth
and barHeight
variables.
This is just a simple example, but the HTML5 Canvas API provides many other functions and features that can be used to create more complex and sophisticated graphs and charts. For example, you can use the strokeRect function to draw a rectangle with an outline, or the arc function to draw a circular or elliptical shape. You can also use the fillStyle
and strokeStyle
properties to set the color and style of the shapes you draw.
In addition to the 2D drawing API, HTML5 Canvas also provides a 3D drawing API called WebGL. This API allows you to create 3D graphics and animations in your web pages, and is especially well suited for creating interactive visualizations of large, complex data sets.
One of the key benefits of using HTML5 Canvas for data visualization is that it provides a high level of interactivity. For example, you can add event listeners to your canvas elements to respond to user actions such as mouse clicks, mouse movements, and touch events. You can also use JavaScript to dynamically update the data and the visualization in real-time, making it an ideal solution for interactive data dashboards and other applications that need to display dynamic, real-time data.