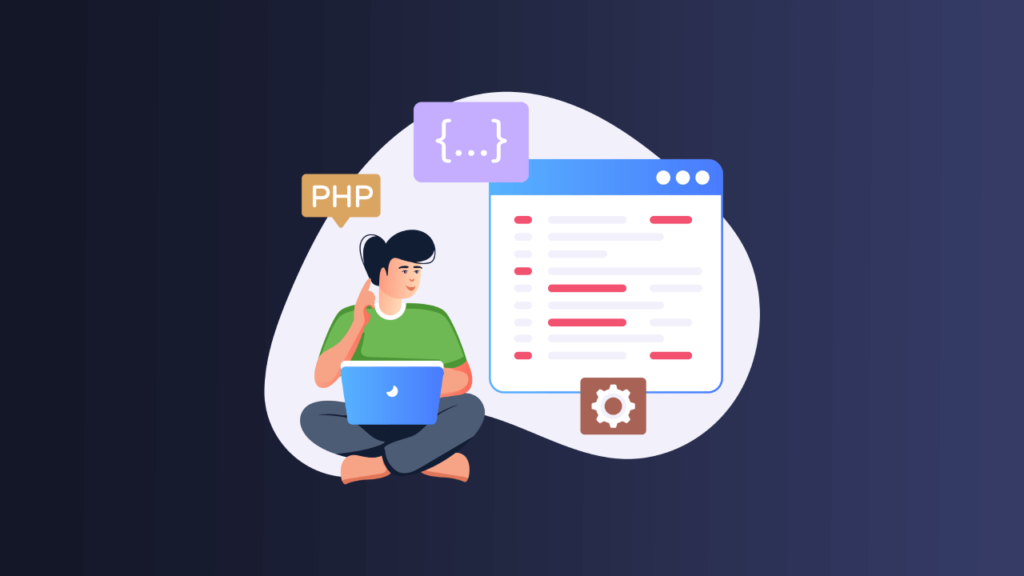
How to create a custom plugin in WordPress
Creating a custom plugin allows you to add specific functionality to your WordPress site that may not be available in existing plugins or themes.
Table of Contents
- Planning your plugin
- Creating your plugin
- Set Up Your Development Environment
- Create a New Folder for Your Plugin
- Create a New PHP File
- Add Plugin Header Information
- Define the Plugin Functions
- Add Activation and Deactivation Functions
- Test Your Plugin
- Publish Your Plugin
- Best practices for WordPress plugin development
- Conclusion
WordPress is a powerful content management system that allows users to easily create and manage websites. One of the key features of WordPress is its ability to extend its functionality through plugins. A plugin is a piece of software that adds new features or modifies existing ones in WordPress.
WordPress plugins can be created by anyone with some programming knowledge. Creating a custom plugin allows you to add specific functionality to your WordPress site that may not be available in existing plugins or themes. It also gives you complete control over the functionality, appearance, and behavior of your site.
In this article, we’ll walk you through the process of creating a custom WordPress plugin from scratch. We’ll cover everything from planning and development to testing and publishing.
Planning your plugin
Before you start writing any code, it’s important to plan out what you want your plugin to do. Start by identifying a specific problem or need that your plugin will address. This could be anything from adding a new feature to your site to optimizing performance.
Once you have a clear idea of what your plugin will do, create a list of the features and functionality it will provide. This will help you stay focused on the goals of your plugin and ensure that it meets your users’ needs.
It’s also important to research any existing plugins or solutions that already address the problem or need you’ve identified. This will help you avoid duplicating functionality and ensure that your plugin offers something new and valuable.
Creating your plugin
Once you’ve planned out your plugin, it’s time to start writing some code. Here are the steps you’ll need to follow
Set Up Your Development Environment
Before you start coding, you’ll need to set up your development environment. This involves installing WordPress on your local machine and setting up a development environment. There are several tools you can use to do this, such as XAMPP, WAMP, MAMP, or Laragon.
Create a New Folder for Your Plugin
Once you’ve set up your development environment, you’ll need to create a new folder in the wp-content/plugins
directory. Give the folder a name that reflects the name of your plugin.
Create a New PHP File
In the folder, you created, create a new PHP file and give it a name that reflects the name of your plugin. This file will be the main plugin file.
Add Plugin Header Information
At the top of your main plugin file, add some header information that WordPress uses to identify your plugin. This includes the plugin name, description, version, author, and other details.
Here’s an example of what the plugin header might look like:
/*
Plugin Name: My Custom Plugin
Plugin URI: https://projectsengine.com
Description: Adds custom functionality to WordPress
Version: 1.0.0
Author: Projects Engine
Author URI: https://projectsengine.com
License: GPL-2.0+
License URI: http://www.gnu.org/licenses/gpl-2.0.txt
*/
Define the Plugin Functions
Now it’s time to start adding the code that defines the functionality of your plugin. This can include functions that modify the way WordPress works, add new features, or interact with external APIs.
For example, let’s say you want to create a plugin that adds a custom post type to WordPress. You could define a function like this:
function my_custom_post_type() {
$args = array(
'public' => true,
'label' => 'My Custom Post Type',
'supports' => array( 'title', 'editor', 'thumbnail' ),
);
register_post_type( 'my_custom_post_type', $args );
}
add_action( 'init', 'my_custom_post_type' );
Add Activation and Deactivation Functions
WordPress runs some code when a plugin is activated or deactivated. You can add your own code to these functions to ensure that your plugin behaves correctly.
For example, let’s say your plugin creates a new database table when it’s activated. You could define a function like this:
function my_custom_plugin_activate() {
global $wpdb;
$table_name = $wpdb->prefix . 'my_custom_table';
$sql = "CREATE TABLE $table_name (
id int(11) NOT NULL AUTO_INCREMENT,
title varchar(255) NOT NULL,
content text NOT NULL,
PRIMARY KEY (id)
);";
require_once( ABSPATH . 'wp-admin/includes/upgrade.php' );
dbDelta( $sql );
}
register_activation_hook( __FILE__, 'my_custom_plugin_activate' );
This function uses the WordPress Database API to create a new table in the database. The register_activation_hook
function tells WordPress to run this function when the plugin is activated.
Similarly, you can define a function that cleans up any data or settings when the plugin is deactivated:
function my_custom_plugin_deactivate() {
// Clean up any data or settings
}
register_deactivation_hook( __FILE__, 'my_custom_plugin_deactivate' );
Test Your Plugin
Once you’ve written your plugin, it’s important to test it thoroughly to make sure it works as expected. Here are some things to check:
- Ensure that the plugin activates and deactivates correctly.
- Verify that any new functionality you’ve added works as expected.
- Test your plugin with different WordPress configurations, themes, and plugins.
- Look for any errors or warnings in the WordPress error log.
Publish Your Plugin
If you’re happy with your plugin and want to share it with others, you can publish it to the WordPress plugin repository. This will make it available to anyone who uses WordPress.
To publish your plugin, you’ll need to follow these steps:
- Sign up for a WordPress.org account.
- Create a readme.txt file that provides information about your plugin.
- Zip up your plugin files.
- Upload your plugin to the WordPress plugin repository.
- Wait for your plugin to be reviewed and approved by the WordPress.org team.
Best practices for WordPress plugin development
Here are some best practices to keep in mind when developing WordPress plugins:
- Use the WordPress Coding Standards: WordPress has its own set of coding standards that you should follow to ensure that your code is consistent and easy to read.
- Keep your code modular: Break your code up into small, reusable functions that can be easily tested and maintained.
- Use hooks and filters: Hooks and filters allow other developers to modify the behavior of your plugin without having to modify its code directly.
- Sanitize user input: Any time your plugin accepts user input, make sure to sanitize and validate it to prevent security vulnerabilities.
- Document your code: Use comments and documentation to explain what your code does and how to use it.
- Test your plugin thoroughly: Make sure to test your plugin in a variety of environments to ensure that it works as expected.
Conclusion
Creating a custom WordPress plugin can be a great way to add new functionality to your site and provide value to your users. By following the steps outlined in this article and following best practices for WordPress plugin development, you can create a plugin that’s secure, maintainable, and easy to use.