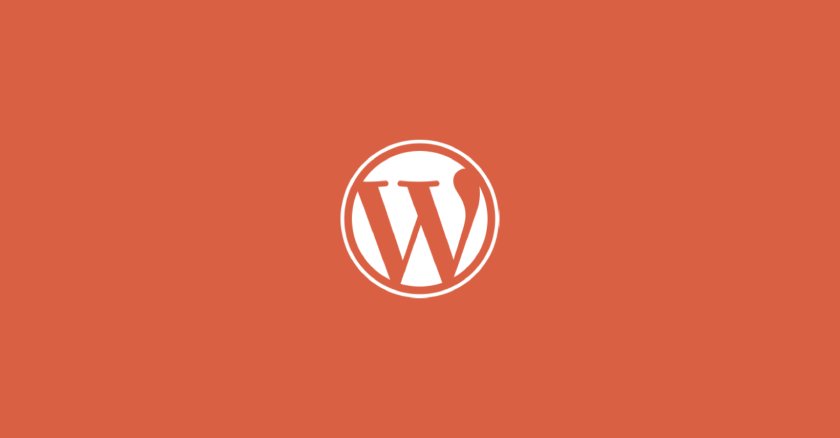
How to create a Custom Element for the WPBakery Page Builder
If you've used WPBakery before and want to create your own custom element, look no further. In this article, we will create our own WPBakery element that will allow us to use our own custom code and design it exactly as we want.
The WPBakery Page Builder formerly known as Visual Composer comes with lots of built-in features to help you customize the frontend more efficiently. If you’ve used WPBakery before and want to create your own custom element, look no further. In this article, we will create our own WPBakery element that will allow us to use our own custom code and design it exactly as we want.
Before we start
The WPBakery Page Builder plugin is not free and it is not available in the WordPress plugin repository. You can buy it from the CodeCanyon store or you can visit the official website.
Where should the code be added?
If you don’t want to use a plugin and instead keep the code in the theme folder, we propose making a separate file for your elements. While you could put all of the code in the functions.php file, it would rapidly become messy and confusing. Instead, create a folder called wpbakery-elements and inside it, create a file that corresponds to the component you’re about to add.
<?php
require_once get_template_directory() . '/wpbakery-elements/shortcodes.php';
require_once get_template_directory() . '/wpbakery-elements/services.php';
How to create a WPBakery element?
There are two ways of creating a custom element for the WPBakery Page Builder:
- extend the WPBakeryShortCode class
- create the shortcode sepeatly and include it in the vc_before_init hook
If you are not familiar with creating shortcodes this guide will help you better understand how they work and how to create them.
Once you have your shortcode ready it is time to include it in the builder. Follow these steps:
<?php
add_action( 'vc_before_init', 'prefix_wpbakery_element_services' );
function prefix_wpbakery_element_services() {
vc_map( array(
"name" => __( "Services", "textdomain" ),
// here you should add the name of your shortcode
"base" => "the_name_of_your_shortcode",
"class" => "",
"icon" => "vc_general vc_element-icon vc_icon-vc-gitem-post-date",
"category" => __( "Content", "textdomain"),
"description" => __( "Display the services we offer." ),
// add a few settings for your element
// for example you can add a dropdown of all cusotm post types
"params" => array(
array(
'type' => 'dropdown',
'heading' => __( 'Post type', 'textdomain' ),
'param_name' => 'cpt',
'value' => array(
array(
'value' => 'service',
'label' => __( 'Services', 'textdomain' ),
),
array(
'value' => 'testimonial',
'label' => __( 'Testimonials, 'textdomain' ),
),
),
'description' => __( 'Select post type', 'textdomain' ),
'admin_label' => false,
'group' => 'prefix-cpt-group',
),
)
) );
}
Parameters
An associative array that stores WPBakery Page Builder instructions that are used in the “mapping” process.
name: string
– Your shortcode’s name
base: string
– Shortcode tag
description: string
– Description of your element
class: string
– On WPBakery Page Builder backend edit mode, a CSS class will be assigned to the shortcode’s content element in the page edit screen.
show_settings_on_create: boolean
– If you don’t want the content element’s settings page not to appear automatically after adding it to the stage, set it to false.
weight: integer
– In the “Content Elements” grid, content elements with a higher weight will be rendered first. (WPBakery Page Builder 3.7 version)
category: string
– Default: Content, Social, Structure. You can add your own. Simply enter a new category title.
group: string
– Group your parameters; they will be separated into tabs in the edit element box. (Available from WPBakery Page Builder 4.1)
admin_enqueue_js: string|array
– This js will be loaded in the js_composer edit mode.
admin_enqueue_css: string|array
– If you need to add custom CSS
front_enqueue_js: string|array
– This js will be loaded in the js_composer frontend edit mode. (WPBakery Page Builder 4.2.2)
front_enqueue_css: string|array
– This css will be loaded in the js_composer frontend edit mode (WPBakery Page Builder 4.2.2)
icon: string
– More info here
custom_markup: string
– Custom HTML markup for displaying the shortcode in the editor
params: array
– List of attributes for the shortcode. An array that holds your shortcode params, these params will be editable on the shortcode settings page
Available type values
textarea_html
– Text area with the default WordPress WYSIWYG Editor.
textfield
– input field
textarea
– textarea field
dropdown
– Dropdown input field
attach_image
– image selection
attach_images
– Multiple images selection
posttypes
– Checkboxes with available post types
colorpicker
– Color picker
exploded_textarea
– Text area, each line will be imploded with a comma (,)
vc_link
– Link selection. use $href = vc_build_link( $href ); to parse link attribute
checkbox
– Creates checkboxes
loop
– Loop builder. Lets your users construct a loop that can later be used during the shortcode’s output
Create a custom element with a class
if ( ! class_exists( 'PREFIX_WPB_Services' ) ) {
class PREFIX_WPB_Services extends WPBakeryShortCode {
// Initialize
function __construct() {
add_action( 'init', array( $this, 'create_shortcode' ), 999 );
add_shortcode( 'vc_soda_blockquote', array( $this, 'render_shortcode' ) );
}
// Map
public function create_shortcode() {
vc_map( array( ) );
}
// Render
public function render_shortcode( $atts, $content, $tag ) {
// output your shortcode on forntend
}
}
new PREFIX_WPB_Services();
}