How to Create a Custom Comments Template in WordPress
WordPress uses the settings and code in the comments.php file in your WordPress theme to show comments in your theme.
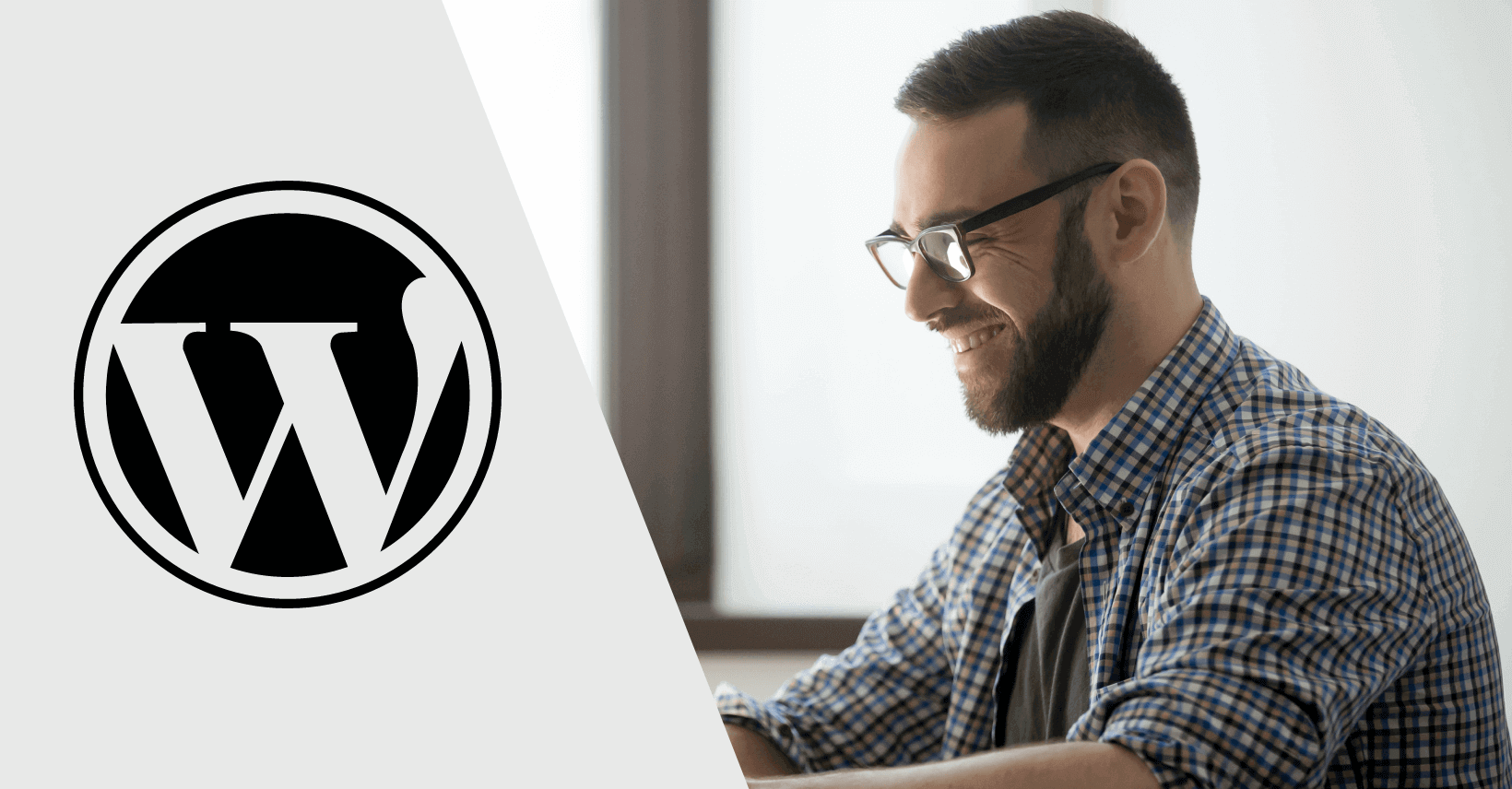
WordPress uses the settings and code in the comments.php file in your WordPress theme to show comments in your theme.
Simple comments loop would look like this:
// Add array of arguments here
$args = array(
);
// The comment Query
$query = new WP_Comment_Query;
$comments = $query->query( $args );
// Comment Loop
if ( $comments ) {
foreach ( $comments as $comment ) {
echo '<p>' . $comment->comment_content . '</p>';
}
} else {
echo '<p>No comments found.</p>';
}
All of the code needed to retrieve comments from the database and show them in your theme is included in the comments.php template.
Next, you need to do is include the template in your single.php or page.php file.
<?php
if ( comments_open() || get_comments_number() ) :
comments_template();
endif;
This means If the comments are open or there is at least one comment, load up the comments template.
It’s easier to create the comments.php template if you divide it down into sections:
- header
- title
- listing the comments
- pagination
- comments are closed
- end
Header
<?php
/**
* The template for displaying the comemnts.
*
* The section of the post or page that includes the comments and the form.
*
* @package WordPress
* @subpackage Projects_Engine
* @since Projects Engine 1.0
*/
Here you can check if the post is protected by a password. If so, you can add this code:
<?php
if ( post_password_required() ) {
return;
}
It means that if the post is protected by a password, the comments won’t be displayed.
Let’s wrap everything together:
<div id="comments" class="comments-area">
<?php if ( have_comments() ) : ?>
Title
<h2 class="comments-title">
<?php
printf( _nx( 'One comment on "%2$s"', '%1$s comments on "%2$s"', get_comments_number(), 'comments title', 'projectsengine' ),
number_format_i18n( get_comments_number() ), '<span>' . get_the_title() . '</span>' );
?>
</h2>
Listing
<ol class="comment-list">
<?php
wp_list_comments( array(
'style' => 'ol',
'short_ping' => true,
'avatar_size' => 50,
'callback' => 'pe_comments'
) );
?>
</ol><!-- .comment-list -->
Use the callback argument to tweak the default listing.
// Filter the comment list arguments
function pe_comments( $comment, $args, $depth ) {
} );
Pagination
<?php
if ( get_comment_pages_count() > 1 && get_option( 'page_comments' ) ) :
?>
<nav class="navigation comment-navigation" role="navigation">
<h3 class="screen-reader-text section-heading"><?php _e( 'Comment navigation', 'projectsengine' ); ?></h3>
<div class="nav-previous"><?php previous_comments_link( __( '← Older Comments', 'projectsengine' ) ); ?></div>
<div class="nav-next"><?php next_comments_link( __( 'Newer Comments →', 'projectsengine' ) ); ?></div>
</nav>
<?php endif; ?>
A condition to check whether there are enough comments to justify adding comment navigation and, if so, adds it.
Comments are closed
<?php if( ! comments_open() && get_comments_number() ) : ?><p class="no-comments"><?php _e( 'Comments are closed.', 'projectsengine'); ?></p><?php endif; ?>
End
<?php endif; // have_comments() ?>
<?php comment_form(); ?>
</div><!-- #comments -->
And you are done. Let’s wrap everything together.
<?php
/**
* The template for displaying the comemnts.
*
* The section of the post or page that includes the comments and the form.
*
* @package WordPress
* @subpackage Projects_Engine
* @since Projects Engine 1.0
*/
if ( post_password_required() )
return;
?>
<div id="comments" class="comments-area">
<?php if ( have_comments() ) : ?>
<h2 class="comments-title">
<?php
printf( _nx( 'One thought on "%2$s"', '%1$s thoughts on "%2$s"', get_comments_number(), 'comments title', 'projectsengine' ),
number_format_i18n( get_comments_number() ), '<span>' . get_the_title() . '</span>' );
?>
</h2>
<ol class="comment-list">
<?php
wp_list_comments( array(
'style' => 'ol',
'short_ping' => true,
'avatar_size' => 50,
) );
?>
</ol><!-- .comment-list -->
<?php
// Are there comments to navigate through?
if ( get_comment_pages_count() > 1 && get_option( 'page_comments' ) ) :
?>
<nav class="navigation comment-navigation" role="navigation">
<h1 class="screen-reader-text section-heading"><?php _e( 'Comment navigation', 'projectsengine' ); ?></h1>
<div class="nav-previous"><?php previous_comments_link( __( '← Older Comments', 'projectsengine' ) ); ?></div>
<div class="nav-next"><?php next_comments_link( __( 'Newer Comments →', 'projectsengine' ) ); ?></div>
</nav><!-- .comment-navigation -->
<?php endif; // Check for comment navigation ?>
<?php if ( ! comments_open() && get_comments_number() ) : ?>
<p class="no-comments"><?php _e( 'Comments are closed.' , 'projectsengine' ); ?></p>
<?php endif; ?>
<?php endif; // have_comments() ?>
<?php comment_form(); ?>
</div><!-- #comments -->
Style it a little bit, and you are good to go.
.post-comments {
margin-top: 1rem;
.social {
margin-bottom: 1.5rem;
.social-container {
background: #ec601c;
padding: 1rem 1rem .8rem 1rem;
.social-wrapper {
a {
background: $black1;
padding: 0.6rem 1rem;
color: $white;
display: inline-block;
margin-bottom: 0.3rem;
font-size: 20px;
&:hover {
background: $black2;
}
}
}
}
}
// next and previous post
nav.navigation.post-navigation {
padding: 1rem;
.nav-links {
display: flex;
flex-direction: row;
.nav-previous, .nav-next {
flex-basis: 50%;
a {
color: $black1;
font-size: 20px;
font-family: $paragraphFont;
.nav-subtitle {
font-family: $paragraphFont;
font-weight: 300;
display: block;
}
.nav-title {
font-family: $paragraphFont;
font-weight: 500;
display: block;
&:hover {
color: $orange;
}
}
}
}
}
}
// title how many comments
h3#comments {
margin: 1.5rem 0 0;
font-size: 20px;
padding: 1rem;
line-height: 24px;
}
// the comments
ol.comment-list {
padding-left: 0;
margin: 0;
padding-bottom: 1.5rem;
list-style-type: none;
li.comment {
img {
width: 50px;
}
article.comment-body {
background: $black1;
border-radius: 10px;
padding: 1rem;
margin-bottom: .8rem;
p, span {
color: $white;
font-size: 18px;
font-family: $paragraphFont;
font-weight: 300;
letter-spacing: .5px;
line-height: 1.5em;
}
.comment-content, .comment-awaiting-moderation {
color: $white;
font-size: 18px;
font-family: $paragraphFont;
font-weight: 300;
letter-spacing: .5px;
line-height: 1.5em;
}
.comment-awaiting-moderation {
margin-left: 1rem;
}
.reply {
display: none;
}
}
ul.children {
list-style-type: none;
li.comment {
article.comment-body {
background: $black2;
}
}
}
}
}
article.comment-body {
footer.comment-meta {
background: $black2;
display: flex;
flex-direction: row;
align-items: center;
.vcard {
display: flex;
flex-direction: row;
align-items: center;
img {
margin-right: 1rem;
}
span.says {
margin: 0 0.4rem;
}
b.fn {
color: #fff;
font-family: $paragraphFont;
font-weight: 400;
}
}
}
}
// form
#respond.comment-respond {
//background: $grey4;
padding: 1rem;
h3.comment-reply-title {
margin: 0;
}
textarea#comment {
width: 96.5%;
font-size: 20px;
border: 1px solid #e0e5e6;
font-family: $paragraphFont;
font-weight: 300;
height: 8rem;
display: block;
padding: 0.5rem;
border-radius: 5px;
margin-top: 0.2rem;
&:focus, &:active {
outline: none;
}
}
form.comment-form {
input[type="text"], input[type="email"], input[type="url"] {
width: 96.5%;
font-size: 20px;
border: 1px solid #e0e5e6;
font-family: $paragraphFont;
font-weight: 300;
height: 25px;
display: block;
padding: 0.5rem;
border-radius: 5px;
margin-top: 0.2rem;
&:focus, &:active {
outline: none;
}
}
}
}
}
Was this post helpful? ( Answers: 13 )
Leave a comment
If you enjoyed this post or have any questions, please leave a comment below. Your feedback is valuable!