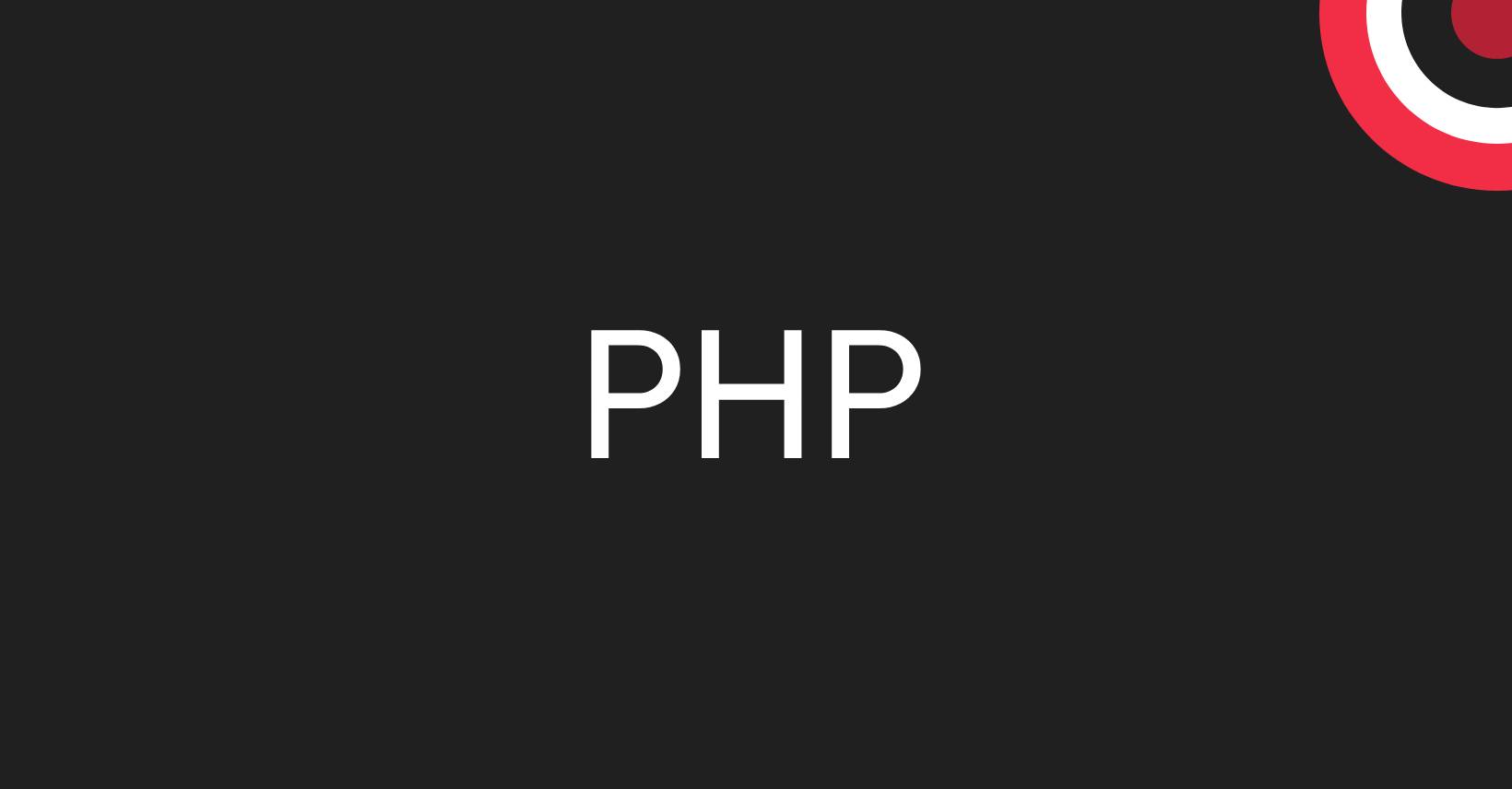
How to convert an array to a comma-separated list in PHP in various ways
How to convert an array into a comma-separated list, for example, when displaying the values of an array in a user-friendly format.
In PHP, arrays are a very useful data structure that allows developers to store multiple values in a single variable. However, there may be times when it is necessary to convert an array into a comma-separated list, for example, when displaying the values of an array in a user-friendly format. In this article, we will explore several ways to convert an array to a comma-separated list in PHP.
Method 1: Using implode() function
The most common and simplest way to convert an array to a comma-separated list is by using the implode()
function in PHP. The implode()
function takes two parameters, the first parameter is the delimiter, which in this case is a comma, and the second parameter is the array to be converted to a string.
Here is an example code snippet demonstrating the usage of implode()
function:
$array = array('apple', 'banana', 'orange');
$list = implode(', ', $array);
echo $list;
Output: apple, banana, orange
Method 2: Using foreach
loop
Another way to convert an array to a comma-separated list is by using a foreach
loop to iterate through the array and append each element to a string variable. In this method, we need to add a comma after each element except for the last one.
Here is an example code snippet demonstrating the usage of foreach
loop:
$array = array('apple', 'banana', 'orange');
$list = '';
$lastIndex = count($array) - 1;
foreach ($array as $index => $value) {
$list .= $value;
if ($index != $lastIndex) {
$list .= ', ';
}
}
echo $list;
Output: apple, banana, orange
Method 3: Using array_reduce() function
Another method to convert an array to a comma-separated list is by using the array_reduce()
function in PHP. The array_reduce()
function applies a callback function to each element of the array and returns a single value. In this method, we will use the callback function to concatenate the elements of the array with a comma.
Here is an example code snippet demonstrating the usage of array_reduce()
function:
$array = array('apple', 'banana', 'orange');
$list = array_reduce($array, function($carry, $item) {
return $carry . $item . ', ';
}, '');
$list = rtrim($list, ', ');
echo $list;
Output: apple, banana, orange
Method 4: Using implode() and array_map() functions
Another way to convert an array to a comma-separated list is by using the combination of implode() and array_map()
functions. The array_map()
function applies a callback function to each element of the array and returns a new array containing the modified elements. In this method, we will use the array_map()
function to add a comma after each element and then join the elements using the implode()
function.
Here is an example code snippet demonstrating the usage of implode()
and array_map()
functions:
$array = array('apple', 'banana', 'orange');
$list = implode(', ', array_map(function($item) {
return $item . ', ';
}, $array));
$list = rtrim($list, ', ');
echo $list;
Output: apple, banana, orange
Method 5: Using join() function
The join()
function is an alias of the implode()
function in PHP, which means that it can also be used to convert an array to a comma-separated list. The join() function takes two parameters, the first parameter is the delimiter, which in this case is a comma, and the second parameter is the array to be converted to a string.
Here is an example code snippet demonstrating the usage of join()
function:
$array = array('apple', 'banana', 'orange');
$list = join(', ', $array);
echo $list;
Output: apple, banana, orange
Conclusion
In this article, we have explored several ways to convert an array to a comma-separated list in PHP. The simplest and most commonly used method is by using the implode() function. However, depending on the situation, other methods can also be useful. It is up to the developer to choose the most appropriate method for their specific needs.