How to add reCaptcha to a Custom Form in WordPress Using Ajax
If you have a custom form in your WordPress site and want to add reCaptcha to it, using Ajax can be a great option.
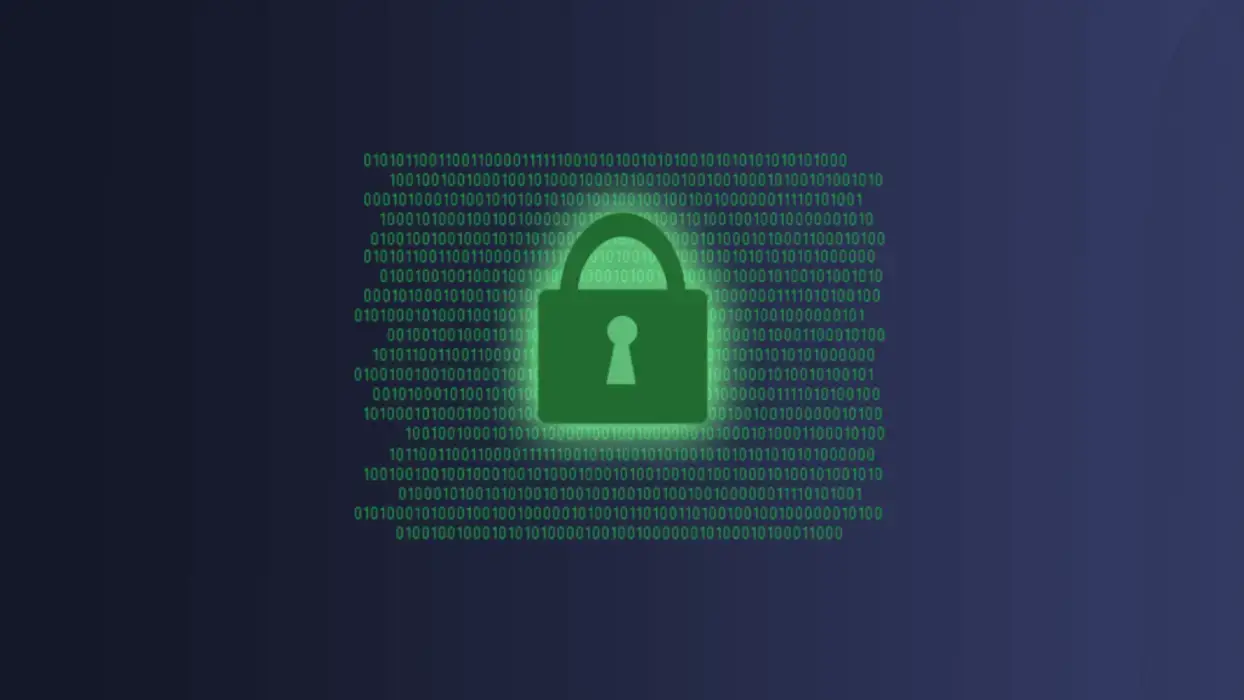
If you have a custom form in your WordPress site and want to add reCaptcha to it, using Ajax can be a great option. Ajax allows you to send data from the form to the server and receive a response without reloading the entire page. In this article, we will guide you through the steps to add reCaptcha to a custom form in WordPress using Ajax.
Step 1: Register for reCaptcha API keys
The first step is to register for reCaptcha API keys. You can do this by visiting the reCaptcha website and clicking on the “Admin Console” button in the top right corner. You will need to provide your website URL and a valid email address. Once you have registered, you will receive a site key and a secret key.
Step 2: Add reCaptcha to your form
To add reCaptcha to your form, you will need to include the reCaptcha API script in your HTML file. You can do this by adding the following code before the ending of the body section of your HTML file. In WordPress just add it to the footer.php
file. Add a condition so the script is loaded only where it is needed.
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
Next, add the reCaptcha widget to your form. You can do this by adding the following code to your form:
<form id="o_post_comment" method="post">
<div class="input-wrapper">
<label for="user_email">Email</label>
<input type="text" name="user_email" id="user_email" />
</div>
<div class="input-wrapper">
<label for="post_comment">Post comment</label>
<textarea name="post_comment" id="post_comment"></textarea>
</div>
<div class="input-wrapper">
<div class="g-recaptcha" data-sitekey="YOUR_SITE_KEY"></div>
</div>
<input type="hidden" name="ID" id="ID" value="<?= get_the_ID(); ?>">
<button class="button" type="submit">Submit</button>
</form>
Replace “YOUR_SITE_KEY” with the site key you received from reCaptcha.
Step 3: Register a custom API endpoint
Next, you need to register a custom API endpoint. You can do this by adding the following code to your functions.php file:
function register_custom_api_endpoint() {
register_rest_route( 'pe-api/v1', '/post-comment', array(
'methods' => 'POST',
'callback' => 'postComment',
'permission_callback' => '__return_true'
) );
}
add_action( 'rest_api_init', 'register_custom_api_endpoint' );
This code registers a custom API endpoint called “pe-api/v1/post-comment
“, using the REST API. The “methods” parameter specifies the HTTP method for the endpoint, and the “callback” parameter specifies the function that will handle the endpoint.
Step 4: Define the endpoint handler function
Next, you need to define the function that will handle the API endpoint. You can do this by adding the following code to your functions.php file:
function postComment( WP_REST_Request $request ) {
$params = $request->get_params();
$comment = $params['comment'];
$ID = $params['ID'];
$userEmail = $params['user_email'];
$reCAPTCHA = $params['recaptcha'];
// Google secret API
$secretAPIkey = 'YOUR_SECRET_KEY';
// reCAPTCHA response verification
$verifyResponse = file_get_contents( 'https://www.google.com/recaptcha/api/siteverify?secret='.$secretAPIkey.'&response='.$reCAPTCHA.'&remoteip='.$this->getIp() );
// Decode JSON data
$response = json_decode( $verifyResponse );
if( !$response->success ) {
return new WP_REST_Response( [
'response' => false,
'message' => __( 'Robot verification failed, please try again..', 'projectsengine' ),
'class' => 'alert-error'
] );
}
wp_insert_comment([
'comment_author_email' => $userEmail,
'comment_author' => $userEmail,
'comment_content' => $comment,
'comment_post_ID' => $ID,
'comment_approved' => 0
]);
return new WP_REST_Response( [
'response' => true,
'message' => __( 'Thank you for leaving a comment.', 'projectsengine' ),
'class' => 'alert-success'
] );
}
This code defines a function called “postComment” that processes the form data and returns a JSON response using the WP_REST_Response class.
Step 5: Add Ajax code to your form
Add the following code to your script file to submit the form data using Ajax:
const postComments = $('#o_post_comment');
postComments.on('submit', function (e) {
e.preventDefault();
const comment = postComments.find('textarea[name="post_comment"]').val();
const ID = postComments.find('input[name="ID"]').val()
const user_email = postComments.find('input[name="user_email"]');
let next = true;
if( grecaptcha === undefined ) {
console.log('Undefined!');
return;
}
const response = grecaptcha.getResponse();
if (!response) {
console.log('Response!');
return;
}
if( '' === user_email.val() ) {
console.log('Email fiedls is empty!');
next = false;
}
if( next ) {
$.ajax({
url: '/wp-json/pe-api/v1/post-comment',
type: 'GET',
data: { comment, ID, user_email:user_email.val(), recaptcha:response },
headers: {'X-WP-Nonce': projectsengine.nonce},
beforeSend: function (xhr) {
postComments.css('opacity', '.6');
}
}).done(function (data) {
postComments.css('opacity', '1');
});
}
});
The success function processes the response from the server and handles the success or error message accordingly.
Conclusion
By following the above steps, you can easily register a custom API endpoint and route in WordPress, and submit form data to it. This allows you to process form data securely and efficiently using the WordPress REST API. Remember to test your form thoroughly to ensure that it is functioning correctly.
Was this post helpful? ( Answers: 2 )
Leave a comment
If you enjoyed this post or have any questions, please leave a comment below. Your feedback is valuable!