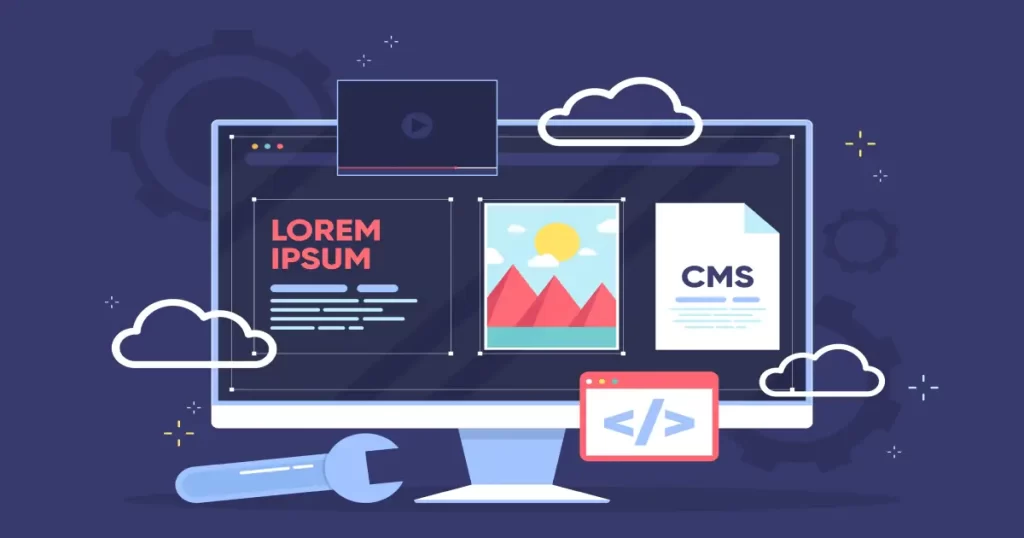
How to add an options page in WordPress
In WordPress, an options page is a page within the WordPress admin area where site owners can manage and configure settings for their WordPress site or plugin.
In WordPress, an options page is a page within the WordPress admin area where site owners can manage and configure settings for their WordPress site or plugin.
Options pages typically provide a user interface with input fields, dropdown menus, checkboxes, and other form elements for site owners to configure various settings related to their site or plugin. For example, an options page for a plugin might allow users to enable or disable certain features, set default values for different parameters, or customize the appearance of the plugin.
To add an options page in WordPress, you can follow these steps:
- Create a new PHP file in your WordPress theme or plugin directory.
- Define a function that will create the options page. You can use the WordPress function
add_menu_page()
oradd_submenu_page()
to create the page. - In the function, define the HTML and PHP code for the options page. This can include form elements, input fields, dropdown menus, and other types of input controls.
- Use the WordPress function
add_action()
to add the options page to the WordPress admin menu. - Save the PHP file and upload it to your WordPress site.
Here’s an example of what the code might look like:
<?php
function my_options_page() {
add_menu_page(
'My Options Page',
'Options',
'manage_options',
'my-options-page',
'my_options_page_content'
);
}
function my_options_page_content() {
echo '<div class="wrap">';
echo '<h2>My Options Page</h2>';
echo '<form method="post" action="options.php">';
settings_fields('my_options_group');
do_settings_sections('my-options-page');
submit_button('Save Settings');
echo '</form>';
echo '</div>';
}
add_action('admin_menu', 'my_options_page');
In this example, the my_options_page()
function uses add_menu_page()
to create the options page and the my_options_page_content()
function defines the content of the page. The settings_fields()
and do_settings_sections()
functions are used to handle the saving of options, and the submit_button()
function adds a button to save the settings.
You can customize this code to add your own options and functionality to your WordPress site.