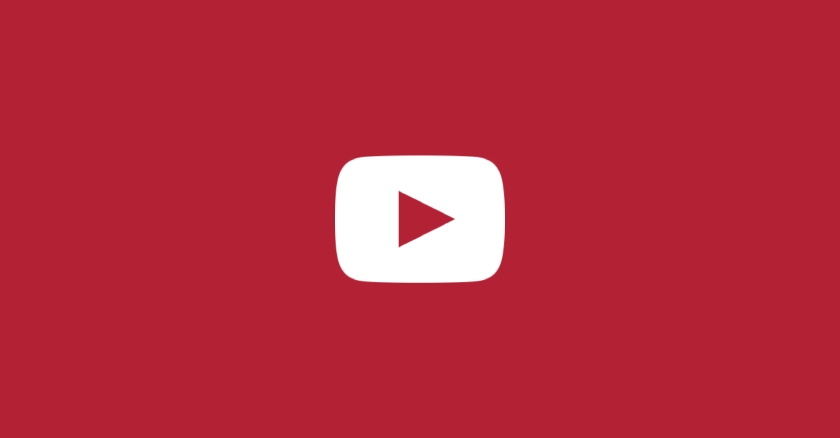
Extracting Embed URLs from YouTube Links Using PHP
Embedding YouTube videos in web pages is a common requirement for many web developers.
Embedding YouTube videos in web pages is a common requirement for many web developers. To facilitate this, it’s often necessary to convert a standard YouTube URL into an embed URL, which can then be used in an <iframe>
tag to display the video directly on your site.
PHP function for generating YouTube embed URLs
Embedding YouTube videos on a website can be greatly simplified with a PHP function designed to convert standard YouTube URLs into embed URLs. Below is an efficient function called getYoutubeEmbedUrl
that achieves this.
This function takes a YouTube URL as input and returns the corresponding embed URL if the input URL is valid. If the URL is invalid, it returns false
. Here is the complete code:
function getYoutubeEmbedUrl($url) {
// Pattern to match YouTube URLs Use heare
$pattern = '/^(?:https?:\/\/)?(?:www\.)?(?:youtube\.com\/(?:[^\/\n\s]+\/\S+\/|(?:v|e(?:mbed)?)\/|\S*?[?&]v=)|youtu\.be\/)([a-zA-Z0-9_-]{11})/';
// Check if the URL matches the pattern
if (preg_match($pattern, $url, $matches)) {
$video_id = $matches[1];
// Construct the embed URL
$embed_url = "https://www.youtube.com/embed/$video_id";
return $embed_url;
} else {
return false; // URL doesn't match the pattern
}
}
Breakdown of the function
The function uses a regular expression to identify and extract the video ID from various formats of YouTube URLs. The pattern matches the following formats:
- Standard YouTube URLs:
https://www.youtube.com/watch?v=VIDEO_ID
- Shortened URLs:
https://youtu.be/VIDEO_ID
- Embed URLs:
https://www.youtube.com/embed/VIDEO_ID
The regular expression used is:
$pattern = '/^(?:https?:\/\/)?(?:www\.)?(?:youtube\.com\/(?:[^\/\n\s]+\/\S+\/|(?:v|e(?:mbed)?)\/|\S*?[?&]v=)|youtu\.be\/)([a-zA-Z0-9_-]{11})/';
This pattern ensures that the function can handle various YouTube URL formats and correctly extract the video ID, which is always 11 characters long.
The preg_match
function is used to apply the pattern to the input URL. If a match is found, the video ID is extracted and stored in the $matches
array.
Once the video ID is extracted, the function constructs the embed URL using the following template:
$embed_url = "https://www.youtube.com/embed/$video_id";
Return value
- If the input URL matches the pattern, the function returns the constructed embed URL.
- If the input URL does not match the pattern, the function returns
false
, indicating an invalid URL.
JavaScript Function for Generating YouTube Embed URLs
Embedding YouTube videos on a website can be greatly simplified with a JavaScript function designed to convert standard YouTube URLs into embed URLs. Below is an efficient function called getYoutubeEmbedUrl
that achieves this in JavaScript.
const getYoutubeEmbedUrl = (url) => {
// Pattern to match YouTube URLs
const pattern = /^(?:https?:\/\/)?(?:www\.)?(?:youtube\.com\/(?:[^\/\n\s]+\/\S+\/|(?:v|e(?:mbed)?)\/|\S*?[?&]v=)|youtu\.be\/)([a-zA-Z0-9_-]{11})/;
// Check if the URL matches the pattern
const matches = url.match(pattern);
if (matches) {
const videoId = matches[1];
// Construct the embed URL
const embedUrl = `https://www.youtube.com/embed/${videoId}`;
return embedUrl;
} else {
return false; // URL doesn't match the pattern
}
}
Usage of the function:
const youtubeURL = 'https://www.youtube.com/watch?v=51zjlMhdSTE&list=RD51zjlMhdSTE';
console.log( getYoutubeEmbedUrl( youtubeURL ) );
// Result: https://www.youtube.com/embed/51zjlMhdSTE
Conclusion
This function is a simple yet powerful tool for web developers who need to embed YouTube videos on their websites. By handling various YouTube URL formats and extracting the necessary video ID, this function ensures that developers can easily convert any valid YouTube link into an embed-friendly format. This enhances the flexibility and user experience of web applications that incorporate video content.