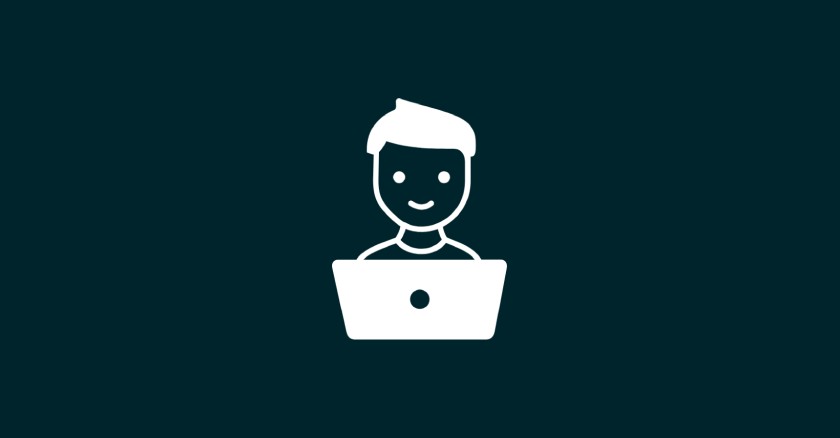
Dynamic Hex to RGBA Conversion in PHP and JavaScript
Learn how to convert hex colors to RGBA dynamically using PHP and JavaScript for flexible and transparent web design.
When building dynamic and responsive websites, the ability to convert hex color codes into RGBA values is invaluable. RGBA allows you to control color transparency, which is essential for modern web design. This guide covers how to implement this functionality in both PHP and JavaScript, so you can handle it server-side and client-side.
Step 1: Convert Hex to RGBA in PHP
Use the following PHP function to convert hex colors into RGBA values. This is ideal for generating dynamic styles server-side in WordPress or other PHP-based platforms.
if( !function_exists('horizon_hex_to_rgba')) {
/**
* Convert hex color to rgba.
*
* @param string $hex The hex color code.
* @param float $opacity The opacity value (0 to 1).
* @return string The RGBA color string.
*/
function horizon_hex_to_rgba($hex, $opacity = 1) {
// Remove the hash if it exists
$hex = str_replace('#', '', $hex);
// Handle short hex codes like #abc
if (strlen($hex) == 3) {
$hex = $hex[0] . $hex[0] . $hex[1] . $hex[1] . $hex[2] . $hex[2];
}
// Convert hex values to RGB
$r = hexdec(substr($hex, 0, 2));
$g = hexdec(substr($hex, 2, 2));
$b = hexdec(substr($hex, 4, 2));
// Ensure opacity is between 0 and 1
$opacity = min(max($opacity, 0), 1);
// Return the RGBA color string
return "rgba($r, $g, $b, $opacity)";
}
}
Usage Example:
$color = horizon_hex_to_rgba('#3498db', 0.5);
echo $color; // Outputs: rgba(52, 152, 219, 0.5)
Step 2: Convert Hex to RGBA in JavaScript
For client-side applications, use JavaScript to achieve the same functionality. This is particularly useful for dynamic UI updates without refreshing the page.
/**
* Convert a hex color to RGBA.
*
* @param {string} hex - The hex color code.
* @param {number} opacity - The opacity value (0 to 1).
* @returns {string} - The RGBA color string.
*/
function hexToRgba(hex, opacity = 1) {
// Remove the hash if it exists
hex = hex.replace('#', '');
// Handle short hex codes like #abc
if (hex.length === 3) {
hex = hex[0] + hex[0] + hex[1] + hex[1] + hex[2] + hex[2];
}
// Convert hex to RGB
const r = parseInt(hex.substr(0, 2), 16);
const g = parseInt(hex.substr(2, 2), 16);
const b = parseInt(hex.substr(4, 2), 16);
// Ensure opacity is between 0 and 1
opacity = Math.min(Math.max(opacity, 0), 1);
// Return the RGBA color string
return `rgba(${r}, ${g}, ${b}, ${opacity})`;
}
Usage Example:
const color = hexToRgba('#3498db', 0.5);
console.log(color); // Outputs: rgba(52, 152, 219, 0.5)
Step 3: Combine PHP and JavaScript for Full Flexibility
For maximum flexibility, combine PHP and JavaScript. Use PHP for server-side color processing and JavaScript for live, client-side updates. Here’s an example of passing the PHP result to JavaScript:
In PHP:
$color = horizon_hex_to_rgba('#e74c3c', 0.8);
echo "<script>const serverColor = '$color';</script>";
In JavaScript:
console.log(serverColor); // Outputs the RGBA value from PHP
Why Convert Hex to RGBA?
- Transparency Control: RGBA adds transparency to hex colors, enabling sleek designs.
- Dynamic Updates: Both server-side (PHP) and client-side (JavaScript) conversions allow dynamic UI updates.
- Cross-Platform Flexibility: Use PHP for backend systems and JavaScript for live user interactions.
Conclusion
Mastering hex to RGBA conversion in both PHP and JavaScript opens up possibilities for creating dynamic, visually stunning designs. Whether you’re processing colors server-side in WordPress or updating them in real-time on the client side, this functionality ensures your site remains modern and engaging.