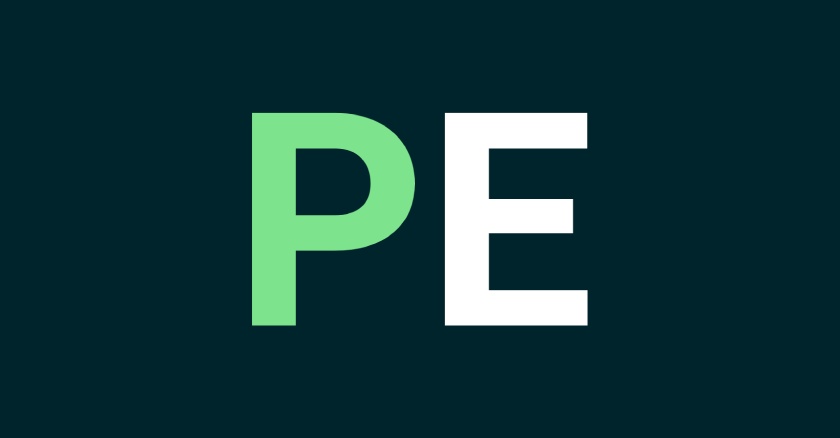
wp_insert_post
When building a website or a blog using WordPress, there may be times when you need to programmatically create posts. This is where the wp_insert_post() function comes in handy.
When building a website or a blog using WordPress, there may be times when you need to programmatically create posts. This is where the wp_insert_post()
function comes in handy.
The wp_insert_post()
function allows you to create a new post and insert it into your WordPress database. It takes an array of arguments that define the post content, such as the title, content, status, and author. Here’s an example of how to use the function:
// Define the post content
$post_data = array(
'post_title' => 'My New Post',
'post_content' => 'This is the content of my new post.',
'post_status' => 'publish',
'post_author' => 1,
);
// Insert the post into the database$post_id = wp_insert_post( $post_data );
In this example, we define the post content as an array of key-value pairs. The post_title
and post_content
keys contain the title and content of the post, respectively. The post_status
key specifies the status of the post, which can be “publish”, “draft”, “pending”, or “private”. The post_author
key specifies the user ID of the post author.
Once the post content is defined, we call wp_insert_post()
and pass the post data as an argument. The function returns the ID of the newly created post, which we can use for further processing.
Here’s another example that shows how to set post meta data using the wp_insert_post()
function:
// Define the post content and meta data
$post_data = array(
'post_title' => 'My New Post',
'post_content' => 'This is the content of my new post.',
'post_status' => 'publish',
'post_author' => 1,
'meta_input' => array(
'my_custom_field' => 'This is a custom field value.',
),
);
// Insert the post into the database
$post_id = wp_insert_post( $post_data );
In this example, we add a new key called meta_input
to the post data array. This key contains an array of key-value pairs that represent the post meta data. In this case, we’re setting a custom field called “my_custom_field” to the value “This is a custom field value.”
The wp_insert_post()
function is a powerful tool for creating posts in WordPress programmatically. With the ability to set post content, status, author, and meta data, you can automate the process of creating posts and save time when building your website or blog.