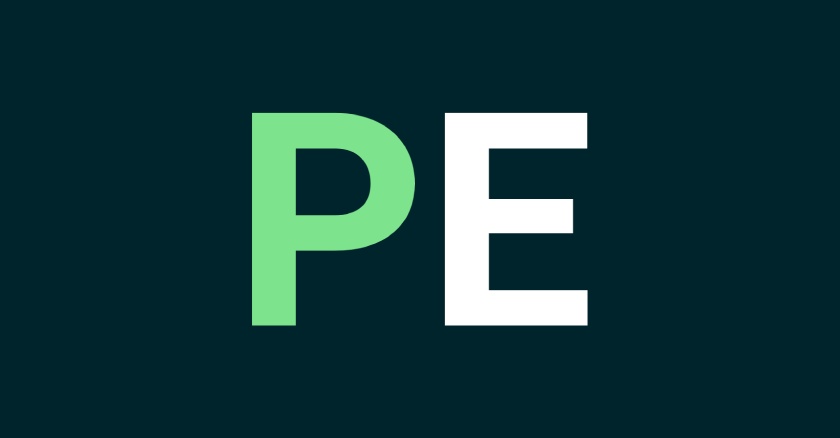
fuzzy searching
Fuzzy searching is a technique used in information retrieval and text processing to find matches for a query term even when the exact term is not known or spelled correctly.
Table of Contents
Fuzzy searching is a technique used in information retrieval and text processing to find matches for a query term even when the exact term is not known or spelled correctly. Instead of requiring an exact match, fuzzy searching algorithms allow for variations in spelling, transpositions, substitutions, or other types of errors or discrepancies.
Fuzzy searching is particularly useful in scenarios where users may make typographical errors, or when dealing with data that is noisy or has inconsistencies. It enables more flexible and forgiving search functionality, improving the chances of retrieving relevant results even when the input does not precisely match the stored data.
One popular algorithm for fuzzy searching is the Levenshtein distance algorithm, which calculates the minimum number of single-character edits (insertions, deletions, or substitutions) required to change one word into another. Other algorithms include the Damerau-Levenshtein distance algorithm, the Jaro-Winkler distance algorithm, and the Soundex algorithm.
Fuzzy searching is commonly used in search engines, spell checkers, data deduplication, and various natural language processing applications to enhance user experience and increase the accuracy of information retrieval.
What is Fuse.js?
Fuse.js is a lightweight fuzzy-search library written in JavaScript that provides a simple yet powerful way to implement fuzzy searching
functionality in web applications. It allows developers to easily add fuzzy-search capabilities to their applications without having to implement complex algorithms from scratch.
Fuse.js offers features such as:
- Fuzzy searching: Fuse.js uses various fuzzy-matching algorithms to find approximate matches for a given query term, allowing for typos, misspellings, and variations in the search input.
- Configurable options: Developers can customize various aspects of the search behavior, including the threshold for matching, the weights assigned to different fields, and the length of the search query.
- Support for multiple data sources: Fuse.js can work with various data structures, including arrays of objects, JSON data, and plain text, making it versatile for different use cases.
- Lightweight and fast: Fuse.js is designed to be lightweight and efficient, ensuring fast search performance even with large datasets.
- Flexibility: Fuse.js provides flexibility in terms of integration with different frameworks and libraries, making it suitable for a wide range of web development projects.
Overall, Fuse.js simplifies the process of implementing fuzzy searching in web applications, making it a popular choice among developers for enhancing search functionality and improving user experience.
Fuse.js is widely used within the web development community, especially in projects where fuzzy-search functionality is required.
Fuse.js and React
Fuse.js can be seamlessly integrated with React applications to provide fuzzy-search functionality. Here’s a general approach to integrating Fuse.js with React:
npm install fuse.js
Import Fuse.js: Import the Fuse.js library into your React component where you want to implement fuzzy search:
import Fuse from 'fuse.js';
Prepare your data: You need to have the data you want to search through available in your React component’s state or props.
Initialize Fuse: Create an instance of Fuse.js with your data and configuration options:
const fuse = new Fuse(yourData, options);
Replace yourData
with the array of objects or other data structures you want to search through, and options
with the configuration options for Fuse.js. Configuration options include things like the keys to search on, the search algorithm, and the threshold for matching.
Perform searches: In your React component, handle user input (e.g., in an input field) and use the Fuse instance to perform searches:
const handleSearch = (query) => {
const result = fuse.search(query);
// Update state with search results
}
- You can call
fuse.search(query)
with the user’s input query to perform a fuzzy search and get back the search results. - Display search results: Update your React component’s state with the search results and render them in your UI.
This is just a basic outline of how you can integrate Fuse.js with React. Depending on your specific requirements and UI structure, you may need to customize the implementation further. Additionally, you might consider using React hooks or state management libraries like Redux to manage the state of your search results and input queries more efficiently.
Fuse.js and Next.js
Integrating Fuse.js with Next.js, a popular React framework for building server-side rendered and statically generated web applications, follows a similar pattern to integrating it with React. Here’s a basic approach:
- Install Fuse.js: First, install Fuse.js in your Next.js project using npm or yarn:
npm install fuse.js
oryarn add fuse.js
- Import Fuse.js: Import the Fuse.js library into your Next.js component where you want to implement fuzzy search:
import Fuse from 'fuse.js';
- Prepare your data: Ensure that the data you want to search through is available within your Next.js component. This could be fetched from an API, loaded from a file, or provided through props.
- Initialize Fuse: Create an instance of Fuse.js with your data and configuration options:
const fuse = new Fuse(yourData, options);
ReplaceyourData
with the array of objects or other data structures you want to search through, andoptions
with the configuration options for Fuse.js, such as the keys to search on and the search algorithm. - Perform searches: Handle user input (e.g., in an input field) and use the Fuse instance to perform searches:
const handleSearch = (query) => { const result = fuse.search(query); // Update state with search results }
Callfuse.search(query)
with the user’s input query to perform a fuzzy search and obtain the search results. - Display search results: Update your Next.js component’s state with the search results and render them in your UI.
Remember that in Next.js, you may be working with server-side rendering or static site generation. Depending on your specific use case, you may need to handle data fetching and state management differently. For server-side rendering, you might perform data fetching in getServerSideProps
or getInitialProps
and pass the data as props to your component. For statically generated pages, you might use getStaticProps
or getStaticPaths
to fetch and pre-render data at build time.
By integrating Fuse.js with Next.js, you can enhance the search functionality of your server-side rendered or statically generated web applications, providing users with a more flexible and forgiving search experience.
What is Redux?
Redux is a predictable state container for JavaScript applications, primarily used with libraries like React or Angular for building user interfaces. It provides a centralized store to manage the state of an application in a predictable way.
Here are some key concepts in Redux:
- Store: The store is a single source of truth that holds the state of your entire application. It is an immutable object tree in Redux. You can think of it as a database for the state of your application.
- Actions: Actions are payloads of information that send data from your application to the Redux store. They are plain JavaScript objects and must have a
type
property that indicates the type of action being performed. Actions are typically created by action creators, which are functions that return action objects. - Reducers: Reducers specify how the application’s state changes in response to actions sent to the store. They are pure functions that take the previous state and an action and return the next state. Reducers must be pure functions, meaning they should not mutate the state directly and should return a new state object if the state changes.
- Dispatch: Dispatch is a method of the Redux store that is used to dispatch actions to the store. When an action is dispatched, the store calls the reducer with the current state and the action, and the state returned by the reducer becomes the new state of the application.
- Selectors: Selectors are functions used to extract specific pieces of data from the store. They help in keeping the components decoupled from the store structure and make it easier to access the required data.
Redux promotes a unidirectional data flow, where data flows in a single direction from the store to the components. This makes it easier to understand how data changes in the application over time and helps in debugging and maintaining large-scale applications.
Redux is often used in complex applications with a large amount of state that needs to be shared across multiple components. It helps in predictably managing the state, making the application easier to debug and test. However, for smaller applications or simpler state management needs, simpler solutions like React’s built-in state management might be more appropriate.