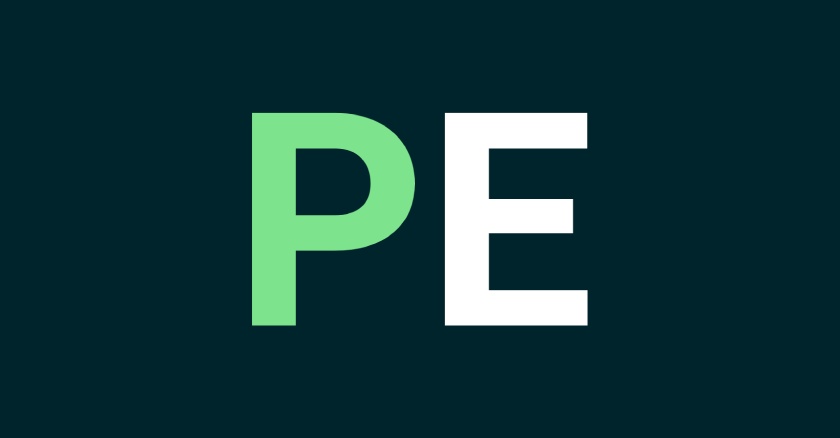
array_unshift
array_unshift() is a PHP function used to add one or more elements to the beginning of an array.
array_unshift()
is a PHP function used to add one or more elements to the beginning of an array. It modifies the original array by adding elements at the start rather than at the end.
Here’s the basic syntax:
array_unshift(array &$array, mixed ...$values): int
$array
: The array to which elements will be added.$values
: The values to be added to the beginning of the array.
The function returns the new number of elements in the array after the operation.
Here are some example:
$colors = array("green", "blue", "yellow");
array_unshift($colors, "red", "orange");
// Resulting array: ["red", "orange", "green", "blue", "yellow"]
$numbers = array(3, 4, 5);
array_unshift($numbers, 1, 2);
// Resulting array: [1, 2, 3, 4, 5]
$assoc_array = array("key1" => "value1", "key2" => "value2");
$main_array = array(3, 4, 5);
array_unshift($main_array, $assoc_array);
// Resulting array: [["key1" => "value1", "key2" => "value2"], 3, 4, 5]
$foods = [
'apples' => [
'McIntosh' => 'red',
'Granny Smith' => 'green',
],
'oranges' => [
'Navel' => 'orange',
'Valencia' => 'orange',
],
'bananas' => [
'Cavendish' => 'yellow',
'Red' => 'red',
],
];
$vegetables = [
'lettuce' => [
'Iceberg' => 'green',
'Butterhead' => 'green',
],
'carrots' => [
'Deep Purple Hybrid' => 'purple',
'Imperator' => 'orange',
],
'cucumber' => [
'Kirby' => 'green',
'Gherkin' => 'green',
],
'tomatoes' => [
'Roma' => 'red',
'Cherry' => 'red',
],
];
// When an associative array is prepended to another associative array, the prepended array becomes numerically indexed within the former array.
$foods = array_unshift($foods, $vegetables);