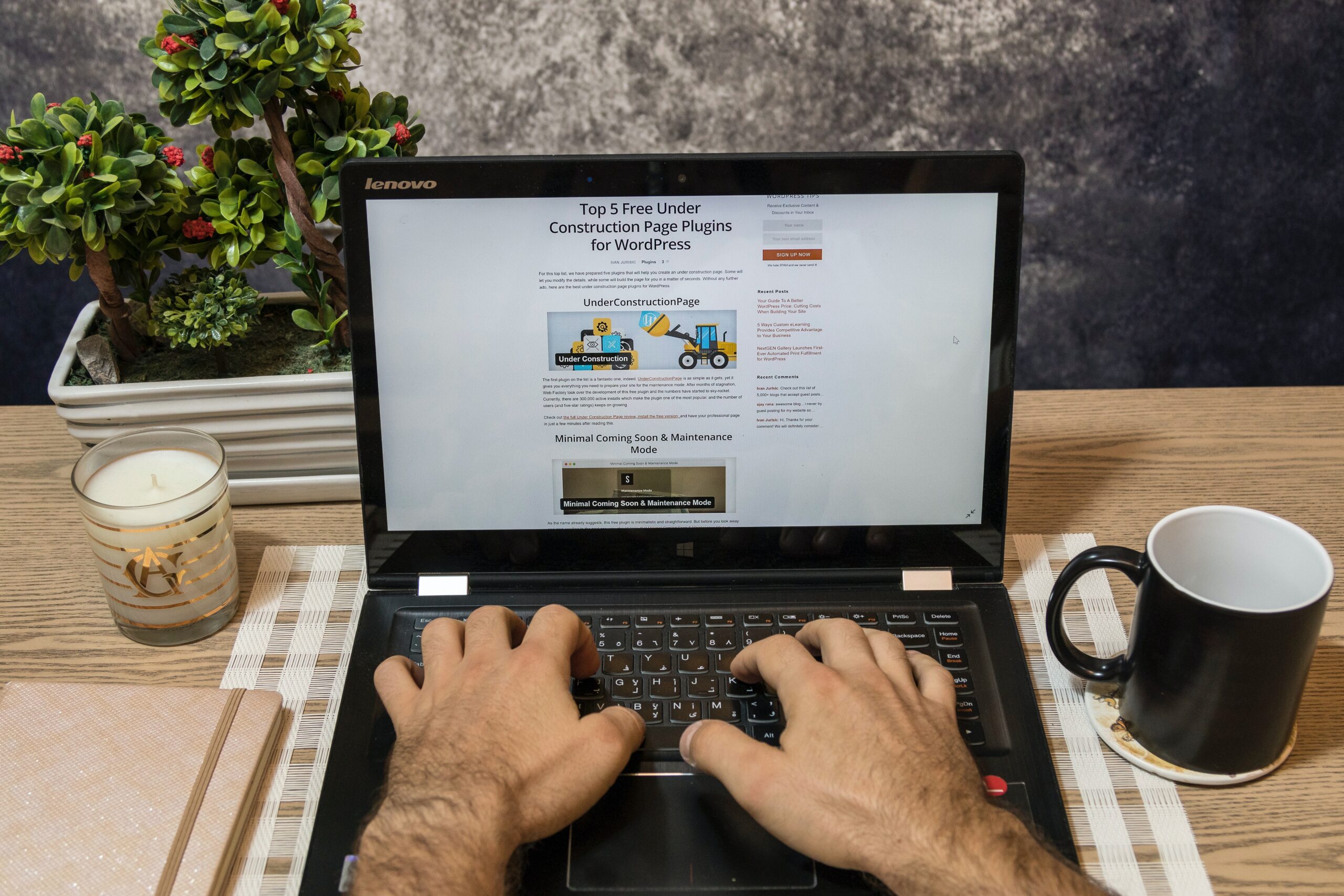
Customizer Settings using Class in WordPress
The Customize API is object-oriented. There are four main types of Customizer objects: Panels, Sections, Settings, and Controls.
Table of Contents
The Customize API is object-oriented. There are four main types of Customizer objects: Panels, Sections, Settings, and Controls. Settings associate UI elements (controls) with settings that are saved in the database. Sections are UI containers for controls, to improve their organization. Panels are containers for sections, allowing multiple sections to be grouped together. Each Customizer object is represented by a PHP class, and all of the objects are managed by the Customize Manager object, WP_Customize_Manager. The Theme Customization API, added in WordPress 3.4, allows developers to customize and add controls to the “Appearance” → “Customize” admin screen. The Theme Customization screen (i.e. “Theme Customizer”) allows site admins to tweak a theme’s settings, color scheme or widgets, and see a preview of those changes in real time.
Developing for the Customizer
To add your own options to the Customizer, you need to use a minimum of 2 hooks:
customize_register
This hook allows you to define new Customizer panels, sections, settings, and controls.
wp_head
This hook allows you to output custom-generated CSS so that your changes show up correctly on the live website.
Include this class in your functions.php
file.
<?php
if ( ! defined('ABSPATH') )
die( esc_html__('Exit if accessed directly.', 'projectsengine'));
class PECustomizer {
public function __construct() {
$this->customizeRegister();
}
public function customizeRegister() {
add_action('customize_register', array($this, 'wp_customize_register'));
add_action('wp_enqueue_scripts', array($this, 'wp_customize_css'));
}
public function wp_customize_register($wp_customize) {
require get_stylesheet_directory() . '/customizer_settings.php';
}
public function wp_customize_css() {
get_template_part('wp_add_inline_style');
}
}
new PECustomizer;
Create these files: customizer_settings.php
and wp_add_inline_style
.
Next, add a theme panel.
// theme options panel
$wp_customize->add_panel( 'pe_panel_theme_options', array(
'priority' => 10,
'capability' => 'edit_theme_options',
'theme_supports' => '',
'title' => __('Theme Options', 'projectsengine'),
'description' => __('Several settings to add to the theme.', 'projectsengine'),
) );
Add sections to the theme panel. The second parameter of the the add_section
is an array where you can specify the panel where you want the section to be added.
$wp_customize->add_section( 'pe_section_header' , array(
'title' => __('Section - Header', 'projectsengine'),
'priority' => 30,
'panel' => 'pe_panel_theme_options',
) );
$wp_customize->add_section( 'pe_section_social_media' , array(
'title' => __('Section - Social Media', 'projectsengine'),
'priority' => 30,
'panel' => 'pe_panel_theme_options',
) );
Add customizer settings to the theme section. Use the add_control
to connect the section and the setting. The second parameter of the add_control
is an array where you can specify the section and the setting.
// Add Background Control
$wp_customize->add_control( new WP_Customize_Image_Control( $wp_customize, 'pe_section_header_bg', array(
'label' => __( 'Background', 'projectsengine' ),
'section' => 'pe_section_header',
'settings' => 'pe_section_header_bg',
)));
// Header - Background
$wp_customize->add_setting(
// Give it an ID
'pe_section_header_bg'
);
Interested in functions, hooks, classes, or methods? Check out the new WordPress Code Reference!
For more up-to-date, detailed information about the Customizer API, please see the new official documentation in the theme handbook: https://developer.wordpress.org/themes/customize-api/.