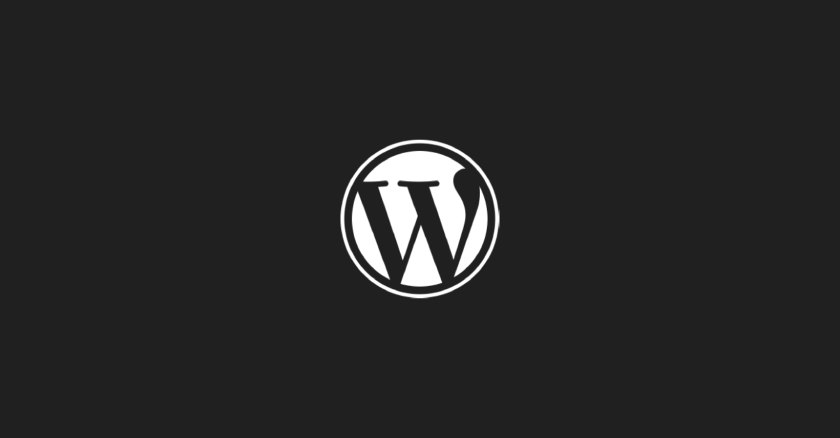
Custom Settings Sidebar for Gutenberg in WordPress
Gutenberg is built using React. This means we are going to add some code in order to be able to create the custom sidebar. This can be done using the registerPlugin function.
Table of Contents
Gutenberg is built using React. This means we are going to add some code in order to be able to create the custom sidebar. This can be done using the registerPlugin
function.
If you are new to React we highly recommend this free course where you can learn the basics of React.
If you are not familiar with how to set up a development environment for Gutenberg, read this post first.
Import registerPlugin
registerPlugin
is a handy helper function. However, before we can access it, we must first import it.
const { registerPlugin } = wp.plugins;
registerPlugin("pe-custom-sidebar", {
icon: 'editor-customchar',
render: CustomSidebarComponent, // Some component for the sidebar.
});
Parameters:
- name, the name of your plugin
- settings – add some settings to your plugin
- seettings.icon – the sidebar cison
- settings.render – componetn to be renderd
Sidebar Component
In order to build the component, we are going to have to import a few more dependencies.
const { __ } = wp.i18n;
const { Fragment } = wp.element;
const { PluginSidebarMoreMenuItem, PluginSidebar } = wp.editPost;
const { withDispatch, withSelect, useSelect } = wp.data;
- Fragment – it is not allowed in React to have sibiling elements, so we will use this component to wrap everyting
- PluginSidebarMoreMenuItem – this redners a menu component in teh sidebar
- Fragment – this compononet will hold the HTML we will provide
const CustomSidebarComponent = () => {
return(
<Fragment>
<PluginSidebar
name="pe-custom-sidebar"
title={__('PE Settings', 'projectsengine')}
>
<PanelBody
title={__('This is a panel section', 'projectsengine')}
initialOpen={true}
>
<PanelRow>
<p>Custom Settings Sidebar for Gutenberg in WordPress</p>
</PanelRow>
</PanelBody>
</PluginSidebar>
<PluginSidebarMoreMenuItem
target='pe-custom-sidebar'
>{__('PE Settings', 'projectsengine')}</PluginSidebarMoreMenuItem>
</Fragment>
);
}
So far, your sidebar should look like this:
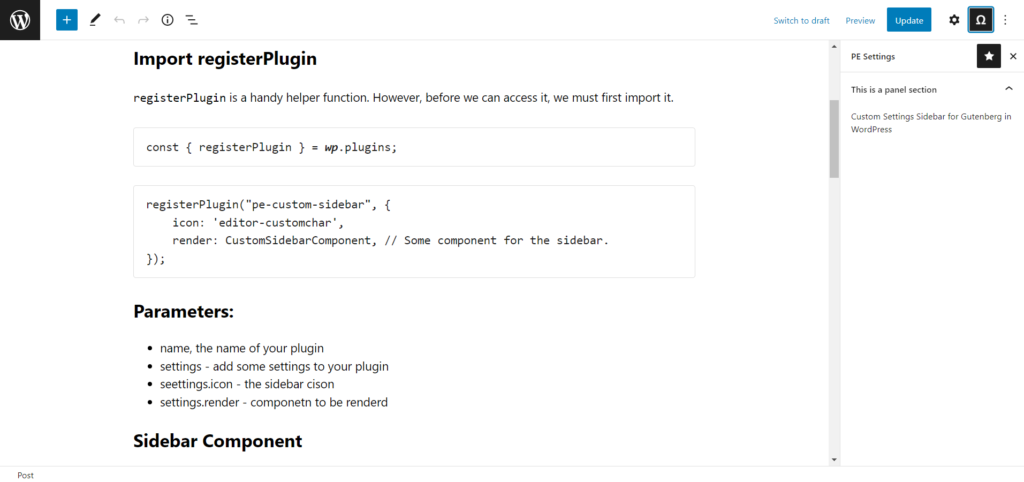
Instead of <p>Custom Settings Sidebar for Gutenberg in WordPress</p>
let’s try and add something else, a toggle button, for example.
Add this code instead of the text: <CustomSidebarPostMeta />.
We will write the code for this component separately.
const EnableSidebarMetaComponent = (props) => {
return(
<ToggleControl
label={__('Enable toggle button', 'projectsengine')}
checked={props.enable}
onChange={props.setPostMeta}
/>
);
}
const CustomSidebarPostMeta = compose([
withSelect(select => {
return { enable: select('core/editor').getEditedPostAttribute('meta')['_enable_random_post'] }
}),
withDispatch(dispatch => {
return {
setPostMeta: function(value) {
dispatch('core/editor').editPost({ meta: { _enable_random_post: value } });
}
}
})
])(EnableSidebarMetaComponent);
Don’t forget to register the post meta for your custom post type like this:
register_post_meta( 'post', '_enable_random_post', [
'show_in_rest' => true,
'single' => true,
'type' => 'boolean',
'auth_callback' => function() {
return current_user_can( 'edit_posts' );
}
] );
Parameters:
- custom post type
- meta key
- array of settings
- show_in_rest – make the field accesable to the API and Gutenberg
If you did everything right the sidebar should look like this:
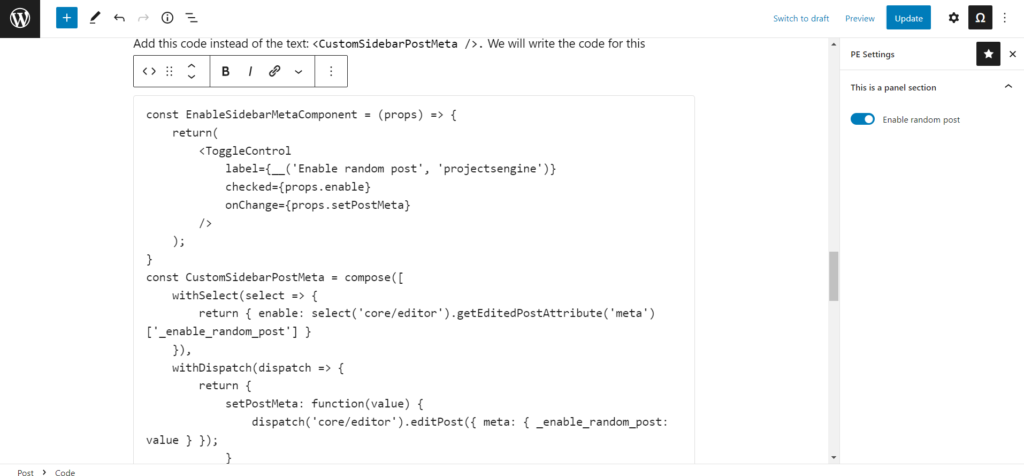
That’s all there is to it when it comes to registering the plugin with Gutenberg and configuring the sidebar. If you have any questions please let us know.