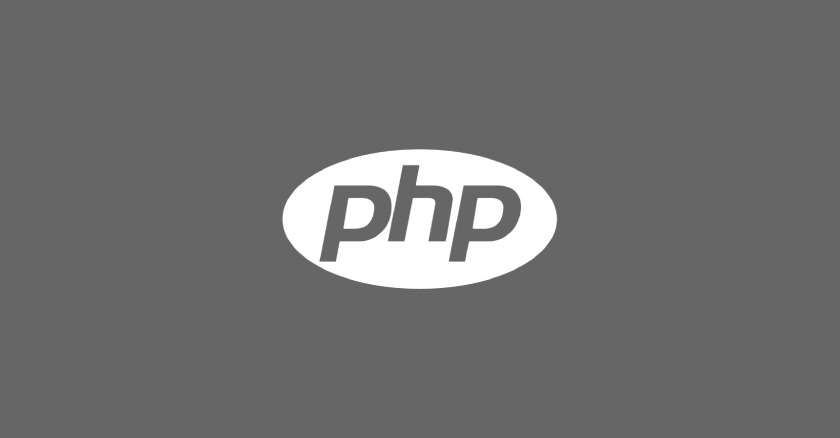
Custom PHP Function to Return Text with Specified Character Length
Creating a custom PHP function to return a specified number of characters from a given text is a common task in web development.
Table of Contents
Creating a custom PHP function to return a specified number of characters from a given text is a common task in web development. Here’s a custom function that does exactly that:
if ( ! function_exists( 'get_text' ) ) {
/**
* Return a specified number of characters from a given text
*
* @param int $length excerpt length.
* @param null|int $post_id post_id.
* @returns string
*/
function get_text( $text, $length = 60 ) {
if( strlen($text) <= $length )
return $text;
// phpcs:ignore
return substr( strip_tags( $text ), 0, $length ) . '...';
}
}
Function definition and check:
- The
get_text
function is defined only if it does not already exist to prevent redeclaration errors. - The function takes two parameters:
$text
(the text to be truncated) and$length
(the number of characters to return, defaulting to 60).
Input validation:
- The function checks if the provided
$text
is a string. If not, it returns an empty string.
Length check:
- If the length of
$text
is less than or equal to$length
, the function returns the original text.
Text truncation:
- If the text exceeds the specified length, it is truncated using
substr
, and an ellipsis (...
) is appended. - The
strip_tags
function is used to remove any HTML tags from the text to ensure the length calculation is accurate and HTML does not interfere with the display.
Usage
This function is particularly useful in contexts where you need to display a preview or excerpt of a larger body of text, such as in blog listings, news articles, or product descriptions. Here’s how you can use it:
$post_content = "<p>This is an example of a longer text that needs to be truncated.</p>";
$excerpt = me_get_text( $post_content, 50 );
echo $excerpt; // Outputs: "This is an example of a longer text that needs..."
By using this function, you ensure that your text snippets are concise and consistent in length, enhancing the overall readability and aesthetics of your website.