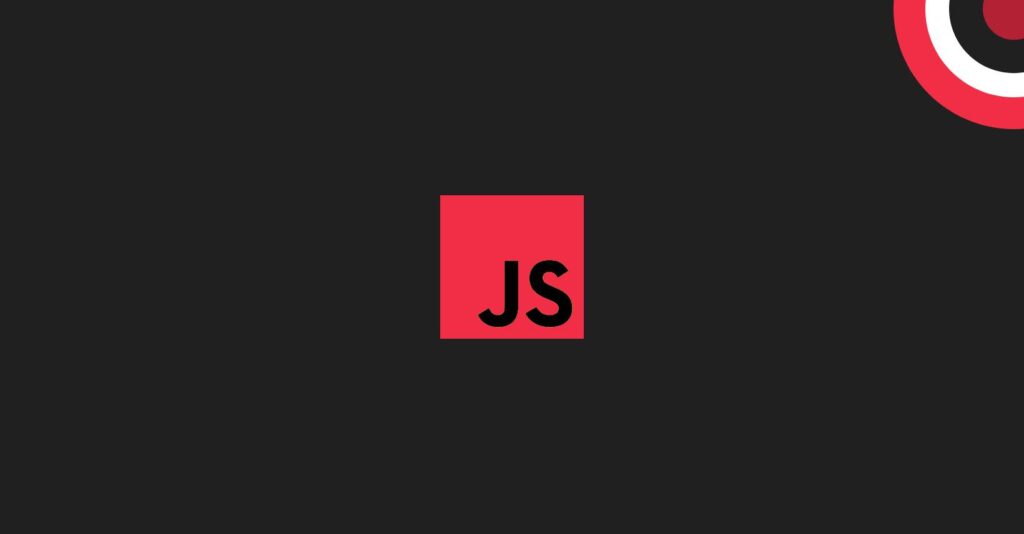
Creating Dynamic Interactions with JavaScript Event Listeners
Explore the power of JavaScript Event Listeners for creating dynamic web interactions. Learn to harness events for responsive and interactive user experiences.
Explore the power of JavaScript Event Listeners for creating dynamic web interactions. Learn to harness events for responsive and interactive user experiences.
Explore the world of web interactivity through JavaScript Event Listeners. Discover how these tools empower developers to create dynamic and responsive user experiences, transforming static pages into engaging web applications. Whether you’re a novice or a seasoned coder, join us in unraveling the intricacies of event handling and unlocking the keys to crafting immersive online environments.
What is an Event?
In the context of web development, an event refers to any occurrence or happening that takes place within a web page. These events can be triggered by various interactions, such as user actions (like clicking a button or pressing a key) or the browser itself (such as the completion of page loading). Events are crucial in enabling dynamic and responsive web applications, as developers can use them to initiate specific actions or execute code in response to user behavior.
What are some of the most common types of events that developers use in JavaScript?
In JavaScript, developers commonly use various events to respond to user interactions and other activities on a webpage. Here are some of the most common types of events:
click
events
Click events are triggered when a user interacts with an element by clicking on it. These events are fundamental for handling user actions like button clicks, link navigation, or interactive elements.
document.getElementById("myButton").addEventListener("click", function() {
alert("Button clicked!");
});
mouseover
and mouseout
events:
Mouseover and mouseout events occur when the mouse pointer enters or leaves a specified element, respectively. These events are useful for creating hover effects and tracking user interactions.
document.getElementById("myElement").addEventListener("mouseover", function() {
console.log("Mouse over the element!");
});
document.getElementById("myElement").addEventListener("mouseout", function() {
console.log("Mouse out of the element!");
});
keydown
, keypress
, and keyup
events:
These events capture keyboard interactions. Keydown
is triggered when a key is pressed, keypress
is triggered when a character key is pressed, and keyup
is triggered when a key is released.
document.addEventListener("keydown", function(event) {
console.log("Key pressed:", event.key);
});
submit
events:
Submit events are associated with form elements and are triggered when a form is submitted, either by clicking a submit button or pressing Enter.
document.getElementById("myForm").addEventListener("submit", function(event) {
event.preventDefault(); // Prevents the default form submission
console.log("Form submitted!");
});
Here, the event listener prevents the default form submission and logs a message when the form with the ID “myForm” is submitted.
change
events:
Change events are triggered when the values of form elements, such as checkboxes or dropdowns, change. They are commonly used for real-time updates or validation.
const checkbox = document.getElementById("myCheckbox");
checkbox.addEventListener("change", function() {
console.log("Checkbox state changed!");
});
This example logs a message when the state of a checkbox with the ID “myCheckbox
” changes.
load
events:
Load events occur when a webpage or a specific element has finished loading. This is useful for executing scripts or initializing components after the page has loaded.
window.addEventListener("load", function() {
console.log("Page loaded!");
});
The event listener logs a message when the entire page, including its resources, has finished loading.
resize
events:
Resize events are triggered when the browser window is resized. These events are useful for adjusting the layout or updating elements based on the window size.
window.addEventListener("resize", function() {
console.log("Window resized!");
});
window.addEventListener("resize", function() {
const screenWidth = window.innerWidth;
if (screenWidth < 600) {
document.body.style.backgroundColor = "lightblue";
} else {
document.body.style.backgroundColor = "white";
}
});
window.addEventListener("resize", function() {
const imageElement = document.getElementById("responsiveImage");
if (window.innerWidth < 768) {
imageElement.src = "small-image.jpg";
} else {
imageElement.src = "large-image.jpg";
}
});
const updateLayout = () => {
const container = document.getElementById("layoutContainer");
const sidebar = document.getElementById("sidebar");
const content = document.getElementById("content");
if (window.innerWidth < 768) {
container.style.flexDirection = "column";
sidebar.style.order = 2; // Move sidebar below content
} else {
container.style.flexDirection = "row";
sidebar.style.order = 1; // Reset order
}
};
window.addEventListener("resize", updateLayout);
window.addEventListener("load", updateLayout);
focus
and blur
events:
Focus events are triggered when an element gains focus, and blur events occur when an element loses focus. These events are often used for form validation or enhancing user experience.
document.getElementById("myInput").addEventListener("focus", function() {
console.log("Input field focused!");
});
document.getElementById("myInput").addEventListener("blur", function() {
console.log("Input field lost focus!");
});
The event listeners log messages when an input field with the ID “myInput” gains or loses focus.
scroll
events:
Scroll events are fired when the user scrolls through a webpage. These events are useful for implementing scroll-based animations or updating content dynamically.
window.addEventListener("scroll", function() {
console.log("Page scrolled!");
});
This example logs a message when the user scrolls the page.
input
events
Triggered when the value of an input field changes, including typing, pasting, or deleting content.
document.getElementById("textInput").addEventListener("input", function(event) {
console.log("Input changed:", event.target.value);
});
These detailed examples showcase how JavaScript event listeners can be applied to various scenarios, making web pages more interactive and responsive to user actions.
touch
events
A set of events like touchstart
, touchmove
, and touchend
for handling touch-based interactions on touch-enabled devices.
document.getElementById("touchElement").addEventListener("touchstart", function(event) {
console.log("Touch started at:", event.touches[0].clientX, event.touches[0].clientY);
});
Triggered when a user places a touch on a touch-enabled device. This event captures the initial touch position.
document.getElementById("touchElement").addEventListener("touchmove", function(event) {
console.log("Touch moved to:", event.touches[0].clientX, event.touches[0].clientY);
});
Fired when a user moves their finger across the screen during a touch interaction. Useful for tracking continuous touch movements.
document.getElementById("touchElement").addEventListener("touchend", function(event) {
console.log("Touch ended.");
});
Triggered when a user lifts their finger off the touch-enabled device. This event indicates the end of a touch interaction.
document.getElementById("touchElement").addEventListener("touchcancel", function(event) {
console.log("Touch canceled.");
});
Fired when a touch event is abruptly canceled, for instance, due to a system interruption. Useful for handling unexpected touch interruptions.
These touch events enable developers to create touch-friendly interfaces, providing a seamless and responsive experience for users on touch-enabled devices.
Advanced Event Listeners and “this” in JavaScript
While basic event listeners offer great interactivity, advanced techniques unlock even more possibilities. Understanding how “this” behaves within these advanced scenarios is crucial to avoid unexpected behavior in your code. Here’s a deep dive:
1. Event Delegation:
- Instead of attaching individual listeners to every element, listen for events on a higher-level parent element and check “this” within the handler to identify the specific child element that triggered the event.
- This improves performance and simplifies code maintenance.
Example:
document.body.addEventListener("click", function(event) {
if (event.target.classList.contains("my-button")) {
// Handle button click
}
});
2. Anonymous vs. Named Functions:
- Using anonymous functions for event listeners creates a new “this” scope, often referencing the window object.
- Using named functions preserves the “this” context from the surrounding scope.
- Consider arrow functions, which inherit “this” from the enclosing scope.
Example:
button.addEventListener("click", function() {
// This refers to the window object (anonymous)
});
button.addEventListener("click", handleClick);
function handleClick() {
// This refers to the button element (named)
}
3. Callbacks and Binding:
- When passing functions as arguments, ensure “this” behaves as expected within the callback.
- Use
.bind(this)
to explicitly bind “this” to a specific context before passing the function. - Arrow functions often provide simpler solutions due to implicit “this” binding.
Example:
setTimeout(function() {
// This could refer to the window object
}, 1000);
setTimeout(myFunction.bind(this), 1000); // Explicitly bind "this"
setTimeout(() => {
// This inherits "this" from the enclosing scope (often useful)
}, 1000);
4. Custom Events:
- Create custom events to trigger specific actions within your application.
- Use
dispatchEvent
to fire the event on an element, and event listeners on other elements can react to it.
Example:
const myEvent = new CustomEvent("myCustomEvent", { detail: { data: "Hello!" } });
element.dispatchEvent(myEvent);
element.addEventListener("myCustomEvent", function(event) {
console.log(event.detail.data); // Outputs "Hello!"
});
5. Event Bubbling and Capturing:
- Understand how events propagate up the DOM tree (bubbling) and how
event.stopPropagation()
can control it. - Use
event.currentTarget
to access the element the event listener is attached to, even during bubbling. - The capturing phase (rarely used) allows handling events before they reach the target element.
Example:
document.body.addEventListener("click", function() {
console.log("body clicked"); // Bubbling phase
}, true); // Use capture phase
element.addEventListener("click", function() {
console.log("element clicked"); // Bubbling phase
event.stopPropagation(); // Stop further bubbling
});
By mastering these advanced concepts, you can create dynamic and flexible event-driven interactions in your JavaScript applications. Remember to always test and debug your code carefully, as “this” behavior can sometimes be tricky.
How to pass arguments through event listeners?
While directly passing arguments to event listener functions isn’t possible, it’s crucial to understand alternative approaches to achieve dynamic interactions.
- Anonymous functions with closure
- Named functions with binding
- Data attributes
- Custom events
- Arrow functions with lexical scope
These approaches ensure that the function is executed only when the event occurs and that the arguments are properly passed within the event context. Here’s a detailed exploration with various methods and examples:
Anonymous Function with Arguments
This method leverages an anonymous function that captures arguments within its closure and accesses them when the event occurs.
// Passing a single argument:
button.addEventListener("click", function(event, message) {
console.log(message); // Outputs "Hello!"
}, "Hello!");
// Passing multiple arguments:
button.addEventListener("click", function(event, data, flag) {
console.log(data, flag); // Outputs "user", true
}, { data: "user", flag: true });
Named Function with Binding
Create a named function, use .bind(this)
to fix its “this” context and pre-bind arguments before attaching the listener.
function handleClick(message) {
console.log(message);
}
button.addEventListener("click", handleClick.bind(this, "Goodbye!")); // Pre-bind the argument
// Bind multiple arguments and "this":
function handleInput(event, value1, value2) {
console.log(value1, value2, this.value); // Access element's value
}
input.addEventListener("change", handleInput.bind(this, 10, 20));
Data Attributes on Elements
Store data directly on the element using data attributes and access it within the event listener.
<button data-action="delete" data-id="123">Delete</button>
button.addEventListener("click", function() {
const action = this.dataset.action;
const id = this.dataset.id;
// Handle action based on data attributes
});
Custom Event with Details
Create a custom event with a “detail” property holding arguments and dispatch it on the element.
const myEvent = new CustomEvent("myCustomEvent", { detail: { data: "Hi!", value: 5 } });
element.dispatchEvent(myEvent);
element.addEventListener("myCustomEvent", function(event) {
const data = event.detail.data;
const value = event.detail.value;
console.log(data, value); // Outputs "Hi!", 5
});
Arrow Functions with Lexical Scope
Utilize arrow functions’ implicit “this” binding and closure to access variables from the surrounding scope.
let message = "Welcome!";
button.addEventListener("click", () => {
console.log(message); // Outputs "Welcome!"
});
Additional Considerations
- Dynamic Data Loading: When arguments depend on external data, fetch them through AJAX or data fetching libraries within the event listener function.
- Performance: For performance-critical scenarios, consider methods like data attributes or custom events, avoiding unnecessary function creations.
- Readability: Choose the method that best suits your code’s clarity and maintainability. Consider using comments to explain complex logic.
Remember: Always test your code thoroughly in different scenarios to ensure arguments are passed correctly and your event listeners function as intended.
Conclusion
In conclusion, mastering event listeners unlocks the door to crafting truly engaging and responsive web experiences. By understanding how to create, attach, and strategically pass arguments to event listeners, you hold the power to transform static pages into dynamic applications that respond seamlessly to user actions.
From simple button clicks to complex animations, form validations, and beyond, event listeners serve as the backbone of interactivity in modern web development. Embrace their potential, experiment with the techniques you’ve learned, and continue exploring the limitless possibilities they offer. Remember, with event listeners at your fingertips, you’re only a click away from breathing life into your web pages and captivating your users in a whole new way.