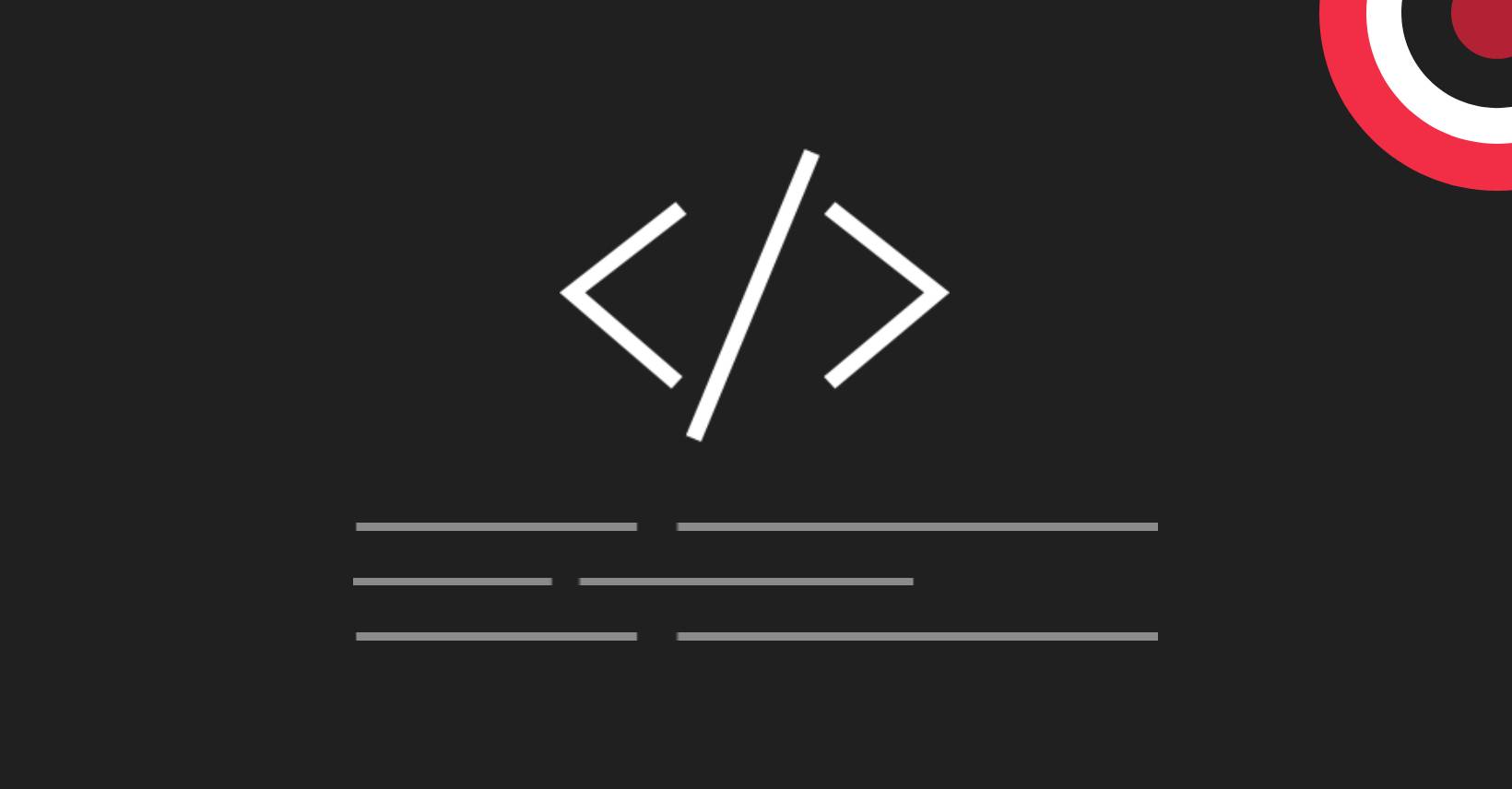
How to use the Math.random() method in JavaScript
Explore the potential of the Math.random() method in JavaScript with our detailed guide. Master random number generation for dynamic and interactive web development.
Math.random()
is a built-in function in JavaScript that returns a floating-point, pseudo-random number in the range from 0 (inclusive) to 1 (exclusive). Here’s an example of how it is typically used:
const randomValue = Math.random();
console.log(randomValue); // Output: A random number between 0 (inclusive) and 1 (exclusive)
If you want to generate a random number within a specific range, you can use Math.random()
along with other mathematical operations. For example, to generate a random integer between min
(inclusive) and max
(exclusive), you can use the following formula:
const getRandomInteger = (min, max) => Math.floor(Math.random() * (max - min)) + min;
const randomInt = getRandomInteger(1, 11); // Generates a random integer between 1 (inclusive) and 11 (exclusive)
console.log(randomInt);
This function, getRandomInteger
, takes min
and max
as parameters and returns a random integer within the specified range.
You can generate a random integer between 1 and 10 in JavaScript using the Math.random()
function. Here’s an example:
const randomInteger = Math.floor(Math.random() * 10) + 1;
console.log(randomInteger);
Math.random()
: Generates a random floating-point number between 0 (inclusive) and 1 (exclusive).Math.floor(Math.random() * 10)
: Multiplies the random number by 10 and rounds it down to the nearest integer, giving a range from 0 to 9.+ 1
: Adds 1 to the result to shift the range from 1 to 10.
This way, randomInteger
will hold a random integer between 1 and 10.