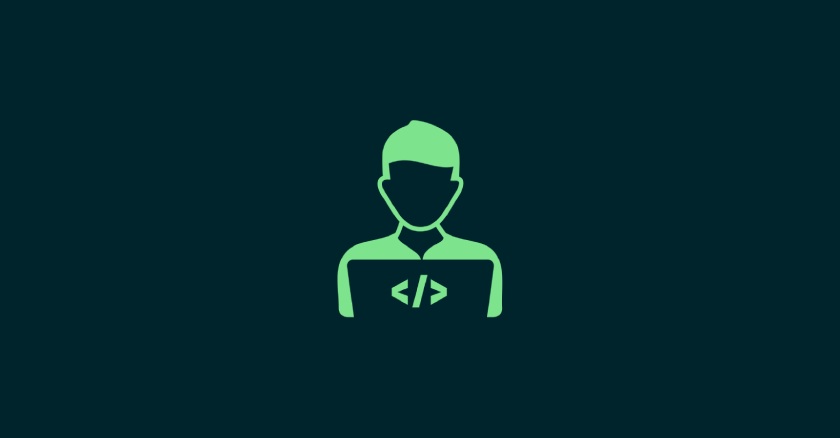
Shortening Anonymous Functions in JavaScript for Cleaner Code
Learn how to make your JavaScript code more concise by shortening anonymous functions using arrow functions, implicit returns, and other best practices.
Table of Contents
- Understanding Anonymous Functions and Arrow Functions
- What Are Anonymous Functions?
- What Are Arrow Functions?
- The Arrow Function: A Shortcut for Anonymous Functions
- Implicit Return for Shorter Code
- Single Argument Arrow Functions
- Object Methods: Shorthand for Anonymous Functions
- Event Listeners: Arrow Functions for a Cleaner Callback
- Best Practices for Shortening Anonymous Functions
- Conclusion
In JavaScript, functions are the backbone of much of the logic in applications. They allow developers to group code together into reusable blocks that can perform specific tasks. One of the most common types of functions are anonymous functions, which are defined without a name and are typically used as arguments for other functions or in event handling.
Another significant advancement in JavaScript is the introduction of arrow functions, which provide a more concise syntax for writing functions. These arrow functions offer several advantages, especially for anonymous functions, making your code shorter, cleaner, and easier to maintain.
In this article, we will dive into the various ways to shorten anonymous functions and explore how using arrow functions and other techniques can improve the readability and performance of your JavaScript code.
Understanding Anonymous Functions and Arrow Functions
What Are Anonymous Functions?
An anonymous function in JavaScript is simply a function that is defined without a name. It’s often used as a function expression, meaning it’s assigned to a variable or passed as an argument to another function. While they provide flexibility, anonymous functions can quickly become bulky and hard to read, especially when used repeatedly.
Example of an Anonymous Function:
const greet = function(name) {
console.log('Hello, ' + name);
};
greet('John'); // Outputs: "Hello, John"
These functions are often used when the function is only needed in the context of an immediate operation, such as in callbacks or event handlers.
What Are Arrow Functions?
Arrow functions were introduced in ES6 (ECMAScript 2015) as a way to make function syntax more concise. They are a shorthand for traditional function expressions and offer benefits like automatic binding of the this
keyword, which is helpful in object methods and callbacks.
Basic Syntax of Arrow Function:
const greet = (name) => {
console.log('Hello, ' + name);
};
greet('John'); // Outputs: "Hello, John"
Arrow functions help reduce the verbosity of code, especially when it comes to anonymous functions. Now, let’s dive into the specific ways you can shorten anonymous functions using arrow functions and other best practices.
The Arrow Function: A Shortcut for Anonymous Functions
Arrow functions provide a more concise syntax for writing anonymous functions. Instead of using the traditional function
keyword, you can define functions with an arrow (=>
), which results in cleaner and more readable code.
Traditional Anonymous Function:
const greet = function(name) {
return 'Hello, ' + name;
};
Shortened Arrow Function:
const greet = (name) => 'Hello, ' + name;
The arrow syntax automatically eliminates the need for the function
keyword and the return
statement (in cases where there’s only a single expression), making the code more succinct.
Implicit Return for Shorter Code
One of the best features of arrow functions is the implicit return. If the function consists of a single expression, you can skip the return
keyword and curly braces.
Traditional Anonymous Function with Return:
const add = function(a, b) {
return a + b;
};
Shortened Arrow Function with Implicit Return:
const add = (a, b) => a + b;
This shorthand saves lines of code while still maintaining the function’s behavior, making it perfect for simple operations.
Single Argument Arrow Functions
For functions that only take a single argument, you can even omit the parentheses around the parameter, further simplifying the code.
Traditional Anonymous Function with One Argument:
const square = function(x) {
return x * x;
};
Shortened Arrow Function with Single Argument:
const square = x => x * x;
This small change results in cleaner and more compact code, especially when dealing with small, straightforward functions.
Object Methods: Shorthand for Anonymous Functions
When defining methods inside an object, JavaScript allows you to use a shorthand syntax for functions. This is particularly useful when you’re working with object-oriented code and can make your code much easier to follow.
Traditional Anonymous Function in an Object:
const person = {
greet: function() {
console.log('Hello!');
}
};
Shorthand Method Definition:
const person = {
greet() {
console.log('Hello!');
}
};
The shorthand method syntax removes redundancy and makes the object definition much cleaner.
Event Listeners: Arrow Functions for a Cleaner Callback
In web development, anonymous functions are often used as callbacks for event listeners. Arrow functions are perfect for this, as they allow you to write the event handler in a more concise and readable manner.
Traditional Event Listener Function:
button.addEventListener('click', function() {
console.log('Button clicked!');
});
Shortened Arrow Function in Event Listener:
button.addEventListener('click', () => {
console.log('Button clicked!');
});
Using arrow functions here eliminates the need for the function
keyword and results in cleaner, more modern code.
Best Practices for Shortening Anonymous Functions
While arrow functions and other techniques for shortening anonymous functions can make your code more concise, it’s important to keep readability in mind. Here are some best practices:
- Use Arrow Functions for Short, Simple Logic: Arrow functions are ideal for small, one-liner functions. If the function logic becomes more complex, consider using a traditional function declaration.
- Avoid Over-Nesting: While shortening functions can be helpful, avoid making your code too terse. If a function becomes too complicated, it’s better to write it out fully for clarity.
- Maintain Consistency: Choose one method for defining functions (either using arrow functions or traditional methods) and stick with it throughout your project for consistency.
Conclusion
Shortening anonymous functions using arrow functions and other shorthand techniques not only makes your JavaScript code more concise but also improves its readability and maintainability. By understanding when and how to use these techniques, you can write cleaner, more efficient code that is easier to work with in the long run.
So, whether you’re working with callbacks, event listeners, or simple operations, leveraging these methods will help streamline your code and make your development process more enjoyable!