Text-to-Speech in JavaScript: Enhancing Web Interactions
By converting text into spoken words, Text-to-Speech in JavaScript allows users to consume information more easily.
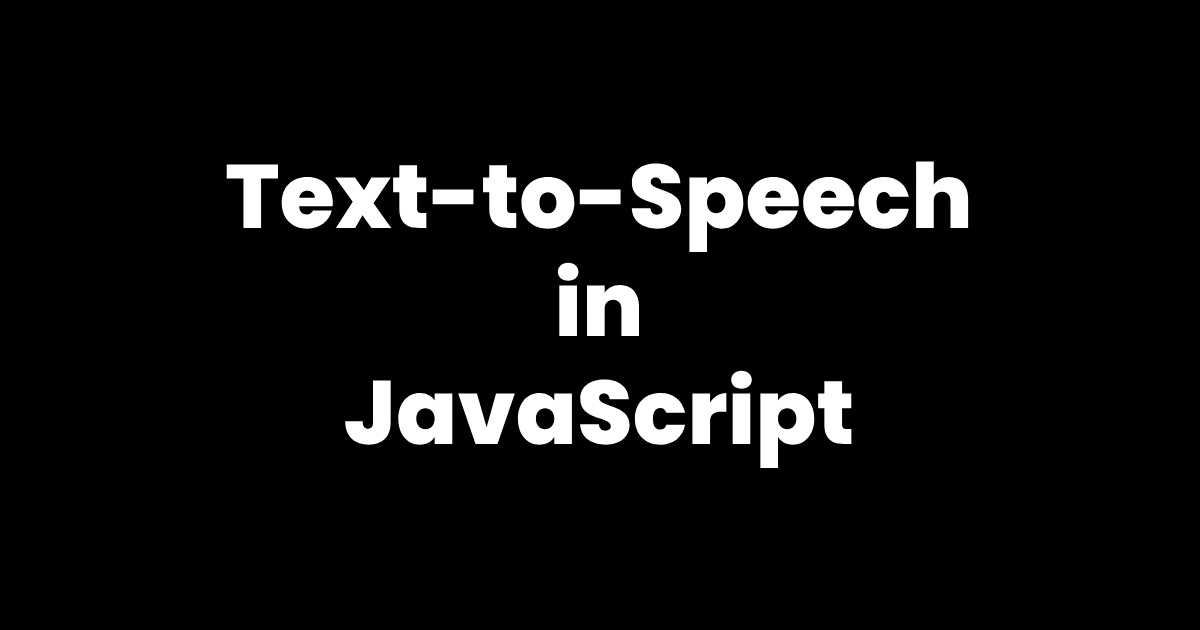
In today’s digital era, accessibility, and user experience are of utmost importance in web development. One powerful tool that can significantly enhance the user experience is Text-to-Speech (TTS) technology. By converting text into spoken words, Text-to-Speech allows users with visual impairments, learning disabilities, or those who prefer audio content to consume information more easily. This article will explore how to implement Text-to-Speech functionality using JavaScript, providing users with an interactive and inclusive web experience.
Setting up the HTML Structure
To begin, we need to set up a basic HTML structure. Let’s create an input field where the user can enter the text they wish to convert into speech and a button to trigger the speech synthesis.
<!DOCTYPE html>
<html>
<head>
<title>Text-to-Speech in JavaScript</title>
</head>
<body>
<input type="text" id="text-input" placeholder="Enter text...">
<button id="speak-button">Speak</button>
<script src="script.js"></script>
</body>
</html>
Implementing Text-to-Speech Functionality
Next, we’ll implement the JavaScript code that handles the conversion of text to speech. Create a new JavaScript file (e.g., script.js
) and link it to the HTML file.
// Retrieve DOM elements
const inputField = document.getElementById('text-input');
const speakButton = document.getElementById('speak-button');
// Create a SpeechSynthesisUtterance instance
const speech = new SpeechSynthesisUtterance();
// Set default speech properties
speech.lang = 'en-US'; // Set the language (e.g., US English)
speech.volume = 1; // Set the volume (0 to 1)
speech.rate = 1; // Set the speech rate (0.1 to 10)
speech.pitch = 1; // Set the speech pitch (0 to 2)
// Event listener for the Speak button
speakButton.addEventListener('click', () => {
const text = inputField.value.trim(); // Get the input text
// Check if text is not empty
if (text !== '') {
speech.text = text; // Set the text to be spoken
speechSynthesis.speak(speech); // Initiate speech synthesis
}
});
Conclusion
By implementing Text-to-Speech functionality in JavaScript, we can create a more inclusive and engaging web experience for users. This technology allows individuals with visual impairments or those who prefer audio content to access information more easily. The provided code demonstrates a simple way to convert text into speech using JavaScript. Feel free to enhance and customize the implementation based on your specific requirements. With Text-to-Speech, we can empower a broader audience to access and interact with digital content.
Was this post helpful? ( Answers: 0 )
Leave a comment
If you enjoyed this post or have any questions, please leave a comment below. Your feedback is valuable!