Welcome to ENGINE Official Website
ENGINE
“Engineering curricula modernization in renewable energy in Albanian
Universities”
Ref.
No. 619338-EPP-1-2020-1-AL-EPPKA2-CBHE-JP
ENGINE – “Engineering curricula modernization in
renewable energy in Albanian Universities”, co-funded by the Erasmus+ Programme
of the European Union and led by Polytechnic University brings together 11
partners. The project aim is the modernization and internationalization of VET
and Bachelor curricula in Engineering for Renewable Energies in the targeted
universities in Albania through innovation of curricula in line with the new
development in the area and the labor market demand. The use of renewable energy
sources and the improvement of energy efficiency ensures a sustainable economy
based on the conditions of scientific development. In order to meet all
national objectives and obligations set by the EU for member states, but also
for candidate countries (such as Albania), it is necessary to raise awareness
of the younger generation on the use of RES, improve energy efficiency, design
curricula and upgrading existing ones, training academic staff and building
laboratories, expanding the network of collaboration with International Higher
Education Institutions for the recognition and implementation of modern energy
technologies.
Project Purpose
The ENGINE project aims to adapt, modernize and restructure existing engineering curricula in renewable energy that perfectly fits into the development strategies of Albania in curriculum modernization of engineering education.
Its specific objectives are:
- To analyze the
educational needs in engineering for renewable energies through problem and job
analysis, and to define the necessary knowledge, skills and competencies of
engineers in the sector of renewable energies in terms of learning outcomes.
- To design syllabi and course content and assessment
for compulsory and elective courses in VET and bachelor engineering education
for renewable energy to meet the market needs and upgrade the university
academic offer accordingly.
- To develop new e-learning courses with modular
structure for the innovated curricula of Partner HEIs and to establish a
platform for knowledge sharing between Albanian HEIs and program partner
institutions.
- To innovate the laboratory equipment and to perform a pilot test
and to start the implementation of the joint modules/courses’ delivery during
the last project year.
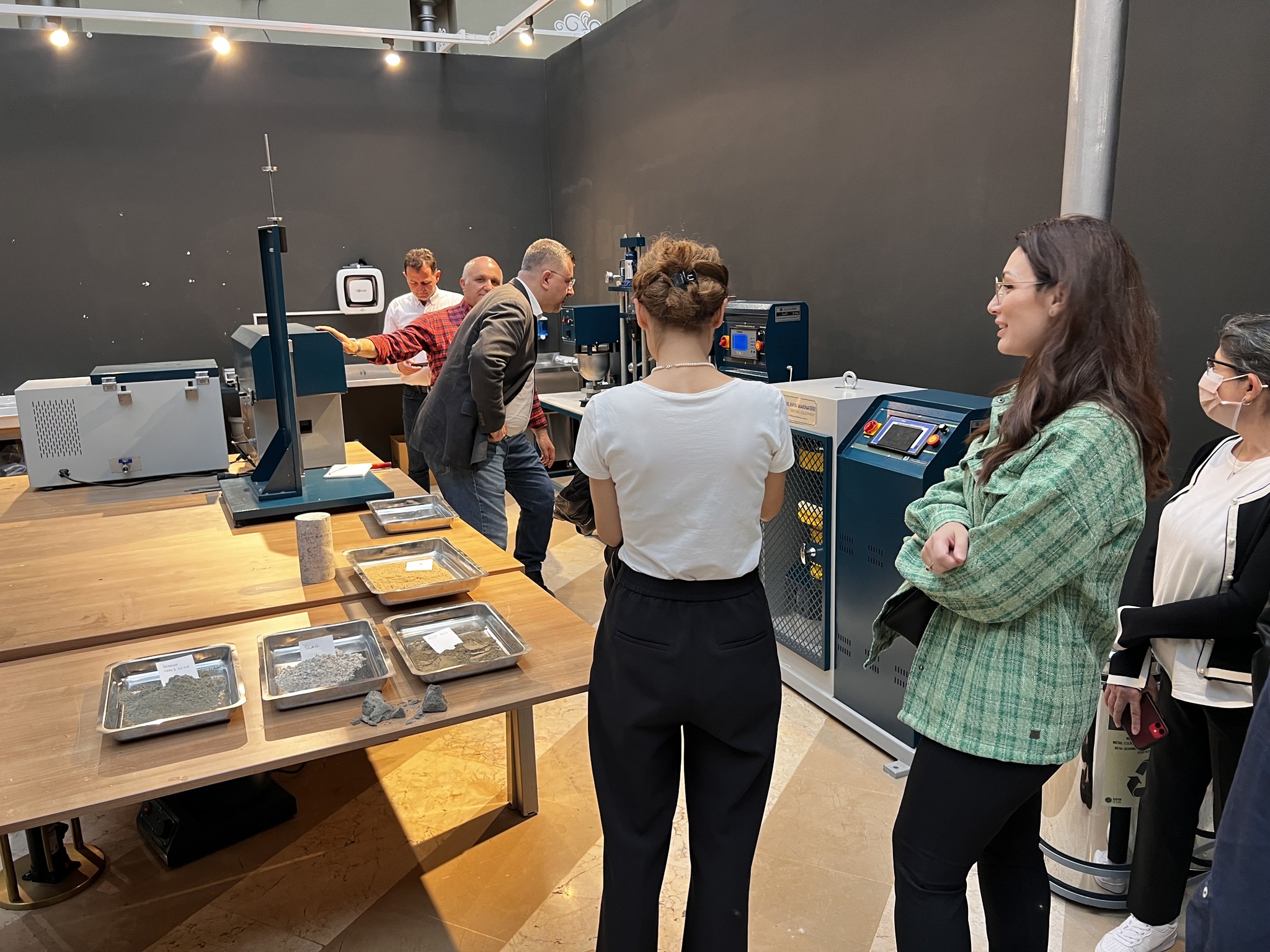
Activities
OUR PARTNERS