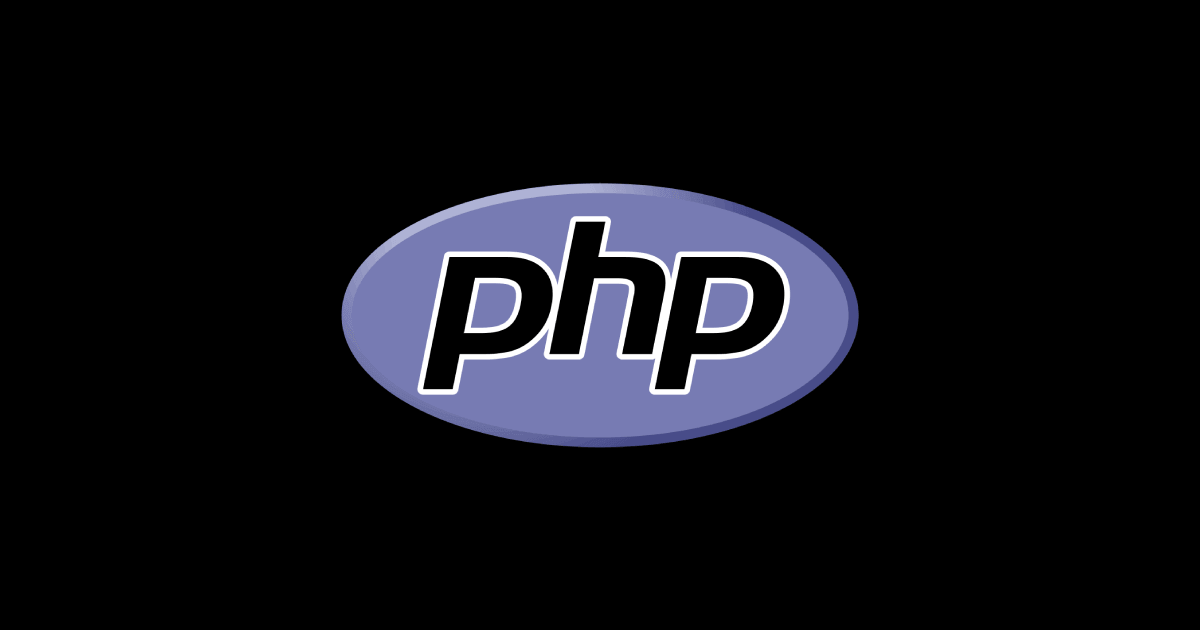
Converting nested arrays to comma-separated lists in PHP in various ways
Converting nested arrays to comma-separated lists is very common in PHP programming. It allows you to convert arrays into a readable format.
Converting nested arrays to comma-separated lists is a common requirement in PHP programming. It allows you to transform complex data structures into a format that is more readable, manageable, and compatible with various applications. In this article, we will explore the top 5 popular methods to achieve this conversion, providing versatile options for effectively handling nested arrays.
Using Recursive Iteration
One of the most straightforward ways to convert nested arrays to comma-separated lists is by using recursive iteration. This method involves recursively traversing the array elements and appending them to a string with commas separating each value. Here’s an example implementation:
function convertArrayToList($array) {
$result = '';
foreach ( $array as $value ) {
if ( is_array($value) ) {
$result .= convertArrayToList( $value ) . ',';
} else {
$result .= $value . ',';
}
}
return rtrim( $result, ',' );
}
// Usage:
$array = [
1,
[2, 3],
[4, [5, 6] ]
];
$array = [
'key1' => 'Value1', [
'key2' => 'Value2',
'key3' => 'Value3'
], [
'key4' => 'Value4',
'key5' => [
'key6' => 'Value5',
'key7' => 'Value6',
]
]
];
$list = convertArrayToList( $array );
echo $list;
// Output: 1,2,3,4,5,6
// Output: Value1,Value2,Value3,Value4,Value5,Value6
Using array_walk_recursive
PHP provides the array_walk_recursive
function, which allows you to apply a custom callback to each nested array element. This method simplifies the conversion process by automatically handling the recursion for you. Here’s an example:
$array = [ 1, [2, 3], [4, [5, 6] ], 7, [8, 9] ];
$array = [
'key1' => 'Value1', [ 'key2' => 'Value2', 'key3' => 'Value3' ],
[ 'key4' => 'Value4', 'key5' => [ 'key6' => 'Value5', 'key7' => 'Value6', ] ]
];
$result = [];
array_walk_recursive($array, function ($value) use (&$result) {
$result[] = $value;
});
$list = implode(',', $result);
echo $list;
// Output: 1,2,3,4,5,6,7,8,9
// Output: Value1,Value2,Value3,Value4,Value5,Value6
Using RecursiveIteratorIterator
PHP’s RecursiveIteratorIterator
is a handy class that allows you to iterate through nested arrays quickly. By combining it with iterator_to_array
, you can flatten the nested array and then convert it to a comma-separated list. Here’s an example:
$array1 = [1, [2, 3], [4, [5, 6]]];
$array2 = [
'key1' => 'Value1', [ 'key2' => 'Value2', 'key3' => 'Value3' ],
[ 'key4' => 'Value4', 'key5' => [ 'key6' => 'Value5', 'key7' => 'Value6', ] ]
];
$iterator = new RecursiveIteratorIterator(new RecursiveArrayIterator($array1));
$result = [];
foreach ($iterator as $value) {
$result[] = $value;
}
$list = implode(',', $result);
echo $list;
// Output: 1,2,3,4,5,6
// Output: Value1,Value2,Value3,Value4,Value5,Value6
We use RecursiveIteratorIterator to iterate through the nested array and RecursiveArrayIterator
as the underlying iterator. By looping through the iterator and collecting the values in the $result
array, we obtain a flattened array. Finally, we implode the flattened array with commas to create the desired comma-separated list.
Using array_map and implode
The combination of array_map and implode functions provide a concise and efficient way to convert nested arrays to comma-separated lists. Here’s an example:
$array = [1, [2, 3], [4, [5, 6]]];
$flatten = function ($item) use (&$flatten) {
return is_array($item) ? implode(',', array_map($flatten, $item)) : $item;
};
$list = implode(',', array_map($flatten, $array));
echo $list; // Output: 1,2,3,4,5,6
Conclusion
Converting nested arrays to comma-separated lists is a common task in PHP development. By leveraging the various methods discussed in this article, you can efficiently transform complex data structures into a format that is more suitable for your needs.