Exploring Object-Oriented Programming in PHP
Object-Oriented Programming (OOP) is a programming paradigm that allows developers to structure their code around objects, which are instances of classes.
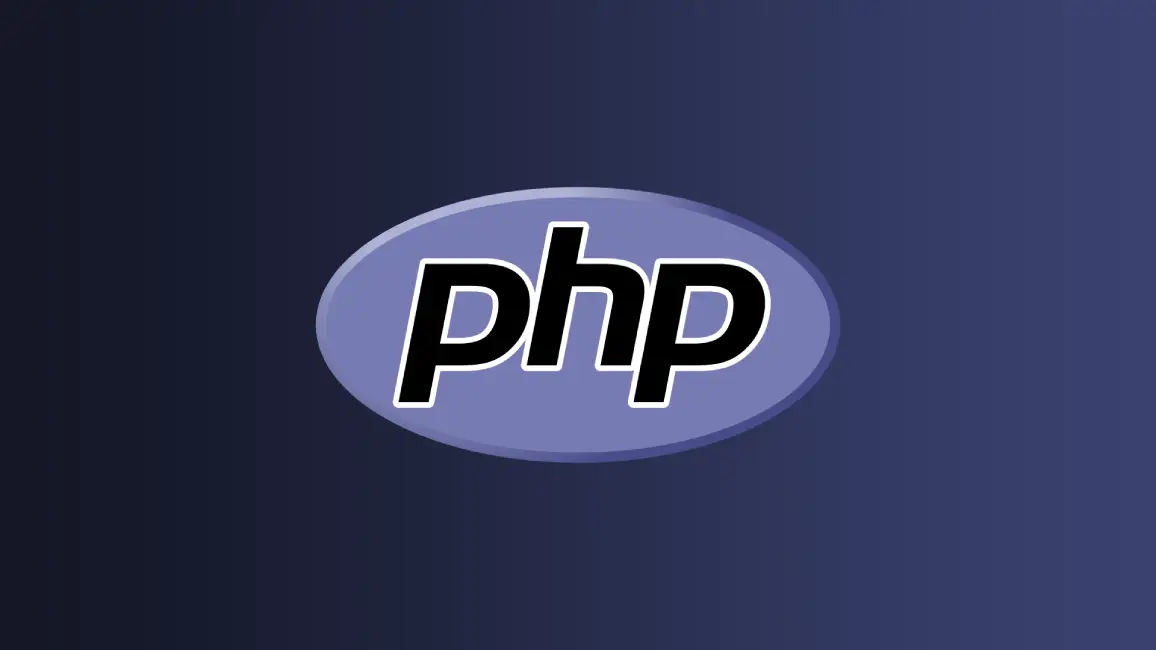
Object-Oriented Programming (OOP) is a programming paradigm that allows developers to structure their code around objects, which are instances of classes. PHP, a popular server-side scripting language, also supports object-oriented programming.
In this article, we will delve into the world of object-oriented programming in PHP, exploring its concepts, principles, and practical implementation.
What is Object-Oriented Programming in PHP?
Object-Oriented Programming in PHP is an approach that enables developers to create modular, reusable, and maintainable code by organizing it into objects. These objects are instances of classes, which encapsulate data and functionality. OOP in PHP provides a way to model real-world entities and their interactions, making code easier to understand and manage.
How to Work with Objects in PHP?
Working with objects in PHP involves several essential operations. First, you must create an object instance by instantiating a class using the ‘new’ keyword. For example:
$car = new Car();
Once you have an object, you can access its properties and methods using the object operator (->). Properties store data related to the object, while methods define its behavior. Here’s an example:
$car->color = 'red'; // Setting a property
echo $car->color; // Accessing a property
$car->startEngine(); // Calling a method
Objects can also be passed as arguments to functions or other methods, enabling you to build complex interactions between objects.
Object-Oriented Concepts and Principles in PHP
To fully understand and utilize object-oriented programming in PHP, it’s crucial to grasp its fundamental concepts and principles. Some of these include:
Classes
Classes are blueprints for creating objects. They define the properties and methods that objects of that class will possess.
Objects
Objects are instances of classes. They encapsulate data and behavior, allowing for modular and reusable code.
Inheritance
Inheritance enables the creation of new classes based on existing ones. Subclasses inherit properties and methods from their parent class, facilitating code reuse and hierarchical relationships.
Polymorphism
Polymorphism allows objects of different classes to be treated as instances of a common superclass. It provides flexibility and enables the use of abstract classes and interfaces.
Encapsulation
Encapsulation ensures that the internal details of an object are hidden from outside access. This protects the integrity of the object’s data and allows for controlled access through public interfaces.
How to Define PHP Classes?
Defining a class in PHP is relatively straightforward. It involves using the ‘class’ keyword followed by the class name and curly braces. Here’s an example of a simple class definition:
class Car {
// Properties
public $color;
public $brand;
// Methods
public function startEngine() {
echo "The car's engine is running.";
}
}
In the above example, we define a class named “Car
” with two properties, “color
” and “brand
,” and a method called “startEngine
.”
class Company {
// Properties
public $name;
public $address;
public function __construct( $name, $address ) {
$this->name = $name
$this->address = $address
}
// Methods
public function getCompanyName() {
return $this->name;
}
public function getCompanyAddress() {
return $this->name;
}
}
In the above example, we define a class named “Company
” with two properties, “name
” and “address
,” and two methods called “getCompanyName
“, and “getCompanyAddress
“.
What are Class Properties in PHP?
Class properties in PHP store data specific to an object. They can be accessed and modified using the object operator (->). Properties can have different visibility levels, such as public, private, or protected. Public properties are accessible from anywhere, while private and protected properties have restricted access. Here’s an example:
class Company {
public $name; // Public property
private $address; // Private property
public function setAddress($address) {
$this->address = $address;
}
public function getAddress() {
return $this->address;
}
}
In the above example, the “price
” property is private and can only be accessed or modified through the class methods.
Constructors for PHP Classes
Constructors are special methods in PHP classes that are automatically called when an object is created. They allow you to initialize object properties and perform any necessary setup. In PHP, the constructor method is named “__construct()
” Here’s an example:
class Project {
private $name;
public function __construct($name) {
$this->name = $name;
echo "A new project has been created.";
}
}
In the above example, the constructor sets the value of the “name” property when a new Project object is created.
Encapsulation
Encapsulation is a fundamental principle of OOP that promotes data protection and controlled access. In PHP, encapsulation is achieved by declaring properties as private or protected and providing public methods (getters and setters) to access or modify the properties. This allows for data integrity and prevents direct manipulation of object properties from outside the class.
Inheritance
Inheritance is a powerful concept in OOP that allows you to create new classes based on existing ones. In PHP, a class can inherit properties and methods from a parent class using the “extends” keyword. This enables code reuse, as well as the ability to specialize or extend the functionality of the parent class in the child class.
Conclusion
Object-Oriented Programming in PHP provides a robust and organized approach to developing applications.
By leveraging classes, objects, and principles such as encapsulation and inheritance, developers can create modular, reusable, and maintainable code. Understanding these concepts and employing them effectively can significantly enhance your PHP programming skills and productivity.
Was this post helpful? ( Answers: 0 )
Leave a comment
If you enjoyed this post or have any questions, please leave a comment below. Your feedback is valuable!