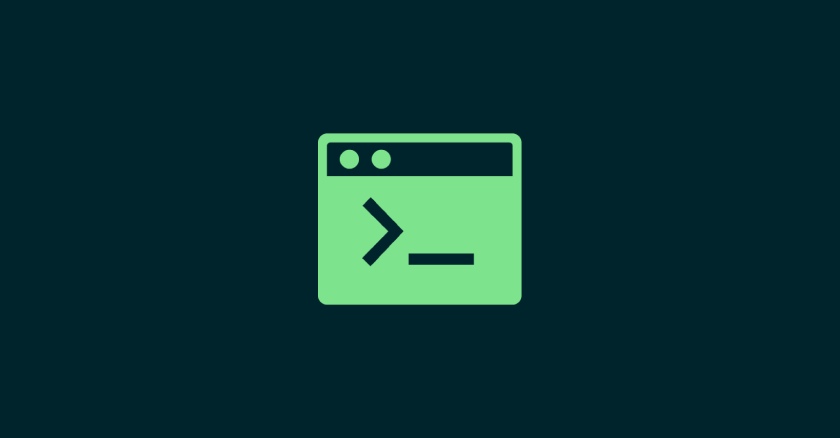
Convert Backslashes to Line Breaks Dynamically in PHP
Learn how to use a simple PHP function to replace backslashes in strings with HTML line breaks for clean and formatted output.
When dealing with dynamic text, you might encounter strings where a backslash (\
) is used to separate lines. To display such text cleanly in HTML, converting backslashes into <br>
tags is essential. This guide introduces a simple PHP function to handle this transformation effectively.
Step 1: Define the Line-to-Break Conversion Function
The following PHP function replaces all occurrences of backslashes in a string with <br>
tags:
if ( ! function_exists( 'horizon_line_to_break' ) ) {
/**
* Convert backslashes to HTML line breaks.
*
* Example: "Line 1\Line 2" becomes "Line 1<br>Line 2".
*
* @param string $text The input string containing backslashes.
*
* @return string The formatted string with line breaks.
*/
function horizon_line_to_break( $text = '' ) {
// Check if the input is not empty, then replace backslashes with <br>
return ! empty( $text ) ? str_replace( '\\', '<br>', $text ) : '';
}
}
Step 2: Use the Function in Your PHP Code
This function can be used in any PHP project where you need to replace backslashes with line breaks. Below are a few examples of how to use it.
Example 1: Basic String Transformation
$text = "Hello\World\PHP";
echo horizon_line_to_break( $text );
Output:
Hello<br>World<br>PHP
Example 2: Formatting an Array of Strings
You can use the function to process multiple strings in an array:
$texts = [
"Item 1\Item 2\Item 3",
"Apple\Orange\Banana"
];
foreach ( $texts as $text ) {
echo horizon_line_to_break( $text ) . '<br><br>';
}
Output:
Item 1<br>Item 2<br>Item 3
Apple<br>Orange<br>Banana
Step 3: Combine with HTML Templates
The function works seamlessly in HTML templates where dynamic content is rendered:
<?php
$message = "Dear Customer\Thank you for your order\Have a great day!";
?>
<div class="formatted-message">
<?php echo horizon_line_to_break( $message ); ?>
</div>
Rendered HTML:
<div class="formatted-message">
Dear Customer<br>Thank you for your order<br>Have a great day!
</div>
Benefits of Using This Function
- Simplifies String Formatting: No need to manually edit strings for line breaks.
- Reusable: Centralize the logic in a single function to use across multiple projects.
- Error-Free Conversion: Automatically handles empty strings and edge cases.
Conclusion
The horizon_line_to_break
function is a straightforward yet powerful tool for transforming backslashes into HTML line breaks. Whether you’re working on email templates, dynamic web content, or text processing scripts, this function makes your PHP projects cleaner and more user-friendly. Start using it today to enhance your text formatting!